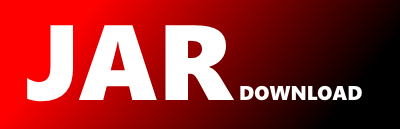
com.iohao.profile.ProfileManager Maven / Gradle / Ivy
package com.iohao.profile;
import jodd.props.Props;
import jodd.props.PropsEntry;
import lombok.AccessLevel;
import lombok.Setter;
import lombok.experimental.FieldDefaults;
import lombok.experimental.UtilityClass;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.*;
/**
* profile 配置与构建
* create time : 2019-02-02 10:14
*
* @author [email protected]
*/
@UtilityClass
public final class ProfileManager {
/** 主配置key */
final String MAIN_CONFIG = "main_config";
private Map profileMap = new HashMap<>();
static {
Profile profile = new Profile();
profile.key = MAIN_CONFIG;
profileMap.put(profile.key, profile);
}
public Builder newBuilder() {
return newBuilder(MAIN_CONFIG);
}
public Builder newBuilder(String configKey) {
Profile profile = new Profile();
profile.key = configKey;
profileMap.put(profile.key, profile);
return new ProfileManager.Builder(profile);
}
public Profile profile() {
return profileMap.get(MAIN_CONFIG);
}
public Profile profile(String key) {
return profileMap.get(key);
}
public void putValue(Profile profile, Properties properties) {
for (Object o : properties.keySet()) {
String key = o.toString();
Object value = properties.get(o);
profile.putValue(key, value);
}
}
public void putFileString(String value) {
putFileString(profile(), value);
}
public void putFileString(Profile profile, String value) {
String[] lines = value.split("\r\n");
for (String line : lines) {
line = line.trim();
if (line.length() == 0 || line.charAt(0) == '#') {
continue;
}
// 分割数据
String[] kvs = line.split("=");
if (kvs.length != 2) {
continue;
}
String pKey = kvs[0].trim();
String pValue = kvs[1].trim();
profile.putValue(pKey, pValue);
}
}
@FieldDefaults(level = AccessLevel.PRIVATE)
@Setter
public static class Builder {
final Profile profile;
public Builder(Profile profile) {
this.profile = profile;
}
public Builder addPropFile(List urls) {
urls.forEach(url -> {
try (InputStream inputStream = url.openStream()) {
Props prop = new Props();
prop.load(inputStream);
Iterator iterator = prop.iterator();
while (iterator.hasNext()) {
PropsEntry entry = iterator.next();
Object value = entry.getValue();
String key = entry.getKey();
profile.putValue(key, value);
}
} catch (IOException e) {
e.printStackTrace();
}
});
/** 需要加载的配置文件 */
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy