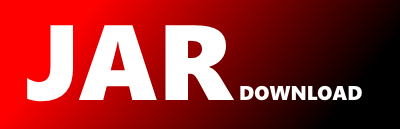
commonMain.com.ionspin.kotlin.bignum.integer.BigIntegerList63Arithmetic.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bignum-jvm Show documentation
Show all versions of bignum-jvm Show documentation
Kotlin Multiplatform BigNum library
/*
* Copyright 2019 Ugljesa Jovanovic
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.ionspin.kotlin.bignum.integer
/**
* Interface defining big integer operations
*
* Created by Ugljesa Jovanovic
* [email protected]
* on 10-Mar-2019
*/
interface BigIntegerList63Arithmetic {
val ZERO: List
val ONE: List
val TWO: List
val TEN: List
val basePowerOfTwo: Int
/**
* Returns the number of leading zeros in a word
*/
fun numberOfLeadingZerosInAWord(value: ULong): Int
/**
* Number of bits needed to represent this number
*/
fun bitLength(value: List): Int
/**
* Number of consecutive zeros count from the right in binary representation
*/
fun trailingZeroBits(value: List): Int
/**
* Arithmetic shift left. Shifts the number to the left, by required places of bits, creating new words if necessary
*/
fun shiftLeft(operand: List, places: Int): List
/**
* Arithmetic shift right. Shifts the number to the right, by required places of bits, removing words that no longer relevant
*/
fun shiftRight(operand: List, places: Int): List
/**
* Compares two numbers
*
* @return -1 if first is bigger, 0 if equal, +1 if second is bigger
*/
fun compare(first: List, second: List): Int
/**
* Adds two big integers
* @return result of add
*/
fun add(first: List, second: List): List
/**
* Subtracts two big integers
* @return result of subtract
*/
fun subtract(first: List, second: List): List
/**
* Multiplies two big integers
* @return result of multiply
*/
fun multiply(first: List, second: List): List
/**
* Divide two big integers
* @return A pair representing quotient (first member of the pair) and remainder (second member of the pair)
*/
fun divide(
first: List,
second: List
): Pair, List>
/**
* Returns a integer reciprocal of this number such that 0 <= base ^ word - operand * reciprocal <= operand,
* and remainder such that 0 < reciprocal < operand
*/
fun reciprocal(operand: List): Pair, List>
/**
* Exponentiation function
* @return BigInteger result of exponentiation of number by exponent
*/
fun pow(base: List, exponent: Long): List
fun sqrt(operand: List): Pair, List>
fun gcd(first: List, second: List): List
/**
* Parse a string in a specific base into a big integer
*/
fun parseForBase(number: String, base: Int): List
/**
* return a string representation of big integer in a specific number base
*/
fun toString(operand: List, base: Int): String
fun numberOfDecimalDigits(operand: List): Long
fun fromULong(uLong: ULong): List
fun fromUInt(uInt: UInt): List
fun fromUShort(uShort: UShort): List
fun fromUByte(uByte: UByte): List
fun fromLong(long: Long): List
fun fromInt(int: Int): List
fun fromShort(short: Short): List
fun fromByte(byte: Byte): List
fun or(operand: List, mask: List): List
fun xor(operand: List, mask: List): List
fun and(operand: List, mask: List): List
fun not(operand: List): List
fun bitAt(operand: List, position: Long): Boolean
fun setBitAt(operand: List, position: Long, bit: Boolean): List
fun fromUByteArray(source: UByteArray): List
fun fromByteArray(source: ByteArray): List
fun toUByteArray(operand: List): UByteArray
fun toByteArray(operand: List): ByteArray
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy