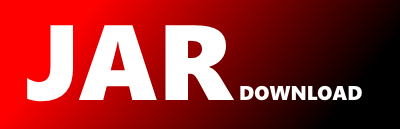
com.irurueta.ar.epipolar.SampsonCorrector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of irurueta-ar Show documentation
Show all versions of irurueta-ar Show documentation
Augmented Reality and 3D reconstruction library
/*
* Copyright (C) 2015 Alberto Irurueta Carro ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.irurueta.ar.epipolar;
import com.irurueta.geometry.CoordinatesType;
import com.irurueta.geometry.Line2D;
import com.irurueta.geometry.Point2D;
import com.irurueta.geometry.estimators.LockedException;
import com.irurueta.geometry.estimators.NotReadyException;
import java.util.ArrayList;
import java.util.List;
/**
* Fixes matched pairs of points so that they perfectly follow a given epipolar
* geometry.
* When matching points typically the matching precision is about 1 pixel,
* however this makes that matched points under a given epipolar geometry (i.e.
* fundamental or essential matrix), do not lie perfectly on the corresponding
* epipolar plane or epipolar lines.
* The consequence is that triangularization of these matches will fail or
* produce inacurate results.
* By fixing matched points using a corrector following a given epipolar
* geometry, this effect is alleviated.
* This corrector uses the Sampson approximation which is capable to remove
* small errors when matches are close to their real epipolar lines. This method
* is faster than the Gold Standard method, but can only correct small errors
* (1 or 2 pixels).
*/
public class SampsonCorrector extends Corrector {
/**
* Constructor.
*/
public SampsonCorrector() {
super();
}
/**
* Constructor.
*
* @param fundamentalMatrix fundamental matrix to be set.
*/
public SampsonCorrector(final FundamentalMatrix fundamentalMatrix) {
super(fundamentalMatrix);
}
/**
* Constructor.
*
* @param leftPoints points to be corrected on left view.
* @param rightPoints points to be corrected on right view.
* @throws IllegalArgumentException if provided lists of points don't have
* the same size.
*/
public SampsonCorrector(final List leftPoints, final List rightPoints) {
super(leftPoints, rightPoints);
}
/**
* Constructor.
*
* @param leftPoints points to be corrected on left view.
* @param rightPoints points to be corrected on right view.
* @param fundamentalMatrix fundamental matrix to be set.
* @throws IllegalArgumentException if provided lists of points don't have
* the same size.
*/
public SampsonCorrector(final List leftPoints, final List rightPoints,
final FundamentalMatrix fundamentalMatrix) {
super(leftPoints, rightPoints, fundamentalMatrix);
}
/**
* Constructor.
*
* @param listener listener to handle events generated by this class.
*/
public SampsonCorrector(final CorrectorListener listener) {
super(listener);
}
/**
* Constructor.
*
* @param fundamentalMatrix fundamental matrix to be set.
* @param listener listener to handle events generated by this class.
*/
public SampsonCorrector(final FundamentalMatrix fundamentalMatrix,
final CorrectorListener listener) {
super(fundamentalMatrix, listener);
}
/**
* Constructor.
*
* @param leftPoints points to be corrected on left view.
* @param rightPoints points to be corrected on right view.
* @param listener listener to handle events generated by this class.
* @throws IllegalArgumentException if provided lists of points don't have
* the same size.
*/
public SampsonCorrector(final List leftPoints, final List rightPoints,
final CorrectorListener listener) {
super(leftPoints, rightPoints, listener);
}
/**
* Constructor.
*
* @param leftPoints points to be corrected on left view.
* @param rightPoints points to be corrected on right view.
* @param fundamentalMatrix fundamental matrix to be set.
* @param listener listener to handle events generated by this class.
* @throws IllegalArgumentException if provided lists of points don't have
* the same size.
*/
public SampsonCorrector(final List leftPoints, final List rightPoints,
final FundamentalMatrix fundamentalMatrix, final CorrectorListener listener) {
super(leftPoints, rightPoints, fundamentalMatrix, listener);
}
/**
* Corrects the lists of provided matched points to be corrected.
*
* @throws NotReadyException if this instance is not ready (either points or
* fundamental matrix has not been provided yet).
* @throws LockedException if this instance is locked doing computations.
*/
@SuppressWarnings("DuplicatedCode")
@Override
public void correct() throws NotReadyException, LockedException {
if (isLocked()) {
throw new LockedException();
}
if (!isReady()) {
throw new NotReadyException();
}
mLocked = true;
if (mListener != null) {
mListener.onCorrectStart(this);
}
// epipolar lines to be reused for memory efficiency
final Line2D leftEpipolarLine = new Line2D();
final Line2D rightEpipolarLine = new Line2D();
Point2D leftPoint;
Point2D rightPoint;
Point2D leftCorrectedPoint;
Point2D rightCorrectedPoint;
mLeftCorrectedPoints = new ArrayList<>();
mRightCorrectedPoints = new ArrayList<>();
final int size = mLeftPoints.size();
float progress;
float previousProgress = 0.0f;
for (int i = 0; i < size; i++) {
leftPoint = mLeftPoints.get(i);
rightPoint = mRightPoints.get(i);
leftCorrectedPoint = Point2D.create(
CoordinatesType.HOMOGENEOUS_COORDINATES);
rightCorrectedPoint = Point2D.create(
CoordinatesType.HOMOGENEOUS_COORDINATES);
// correct single pair
SampsonSingleCorrector.correct(leftPoint, rightPoint,
mFundamentalMatrix, leftCorrectedPoint, rightCorrectedPoint,
leftEpipolarLine, rightEpipolarLine);
mLeftCorrectedPoints.add(leftCorrectedPoint);
mRightCorrectedPoints.add(rightCorrectedPoint);
if (mListener != null) {
progress = (float) i / (float) size;
if (progress - previousProgress > mProgressDelta) {
// progress has changed significantly
previousProgress = progress;
mListener.onCorrectProgressChange(this, progress);
}
}
}
if (mListener != null) {
mListener.onCorrectEnd(this);
}
mLocked = false;
}
/**
* Gets type of correction being used.
*
* @return type of correction.
*/
@Override
public CorrectorType getType() {
return CorrectorType.SAMPSON_CORRECTOR;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy