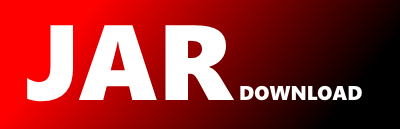
com.isomorphic.maven.mojo.reify.ImportTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of isc-maven-plugin Show documentation
Show all versions of isc-maven-plugin Show documentation
An officially supported collection of goals useful for using SmartClient / SmartGWT products in a Maven environment.
package com.isomorphic.maven.mojo.reify;
import java.io.File;
import org.apache.http.auth.UsernamePasswordCredentials;
import org.apache.maven.settings.Proxy;
import org.apache.tools.ant.BuildException;
import org.apache.tools.ant.PropertyHelper;
import org.apache.tools.ant.Task;
import com.isomorphic.maven.util.AntProjectLogger;
import com.isomorphic.util.ErrorMessage.Severity;
/**
* An Ant task allowing the Reify {@link ImportMojo} to be run from Ant builds. Note that
* default values have been changed to accomodate a typical Ant project structure, otherwise
* functionality is unchanged.
*
* To use this Task, just add a taskdef to the desired build target, and make sure you have the
* required classpath entries set up correctly. If you've built your project using one of the
* Maven archetypes for either
* SmartGWT
* or
* SmartClient
* (and subsequently issued the ant 'unmaven' target), this should all be done for you.
* Otherwise, you'll want to add something like the following to your build:
*
* <property name="reify.lib" value="${basedir}/build/ivy/reify/lib" />
*
* <path id="reify.classpath">
* <fileset dir="${reify.lib}" erroronmissingdir="false">
* <include name="**/*.jar" />
* </fileset>
* </path>
*
* <target name="reify-tasklibs">
* <mkdir dir="${reify.lib}" />
* <ivy:resolve conf="reify" />
* <ivy:retrieve conf="reify" pattern="${reify.lib}/[artifact]-[revision](-[classifier]).[ext]"/>
* </target>
*
* <target name="reify-import" depends="reify-tasklibs">
*
* <taskdef name="reify-import" classname="com.isomorphic.maven.mojo.reify.ImportTask" classpathref="reify.classpath"/>
* <reify-import projectName="MyProject" username="${username}" password="${password}"
* datasourcesDir="WEB-INF/ds/classic-models"
* smartclientRuntimeDir="${basedir}/war/isomorphic" />
* </target>
*
* Use of ivy is of course optional, you just need somehow to get the isomorphic_m2pluginextras module and its
* dependencies
* on your classpath - here they are copied to the directory defined in the reify.lib
property)
*
* Note that users working behind a proxy may need to provide server information to Ant
* by way of the ANT_OPTS environment variable:
*
* export ANT_OPTS="-Dhttp.proxyHost=myproxyhost -Dhttp.proxyPort=8080 -Dhttp.proxyUser=myproxyusername -Dhttp.proxyPassword=myproxypassword"
*
*/
public class ImportTask extends Task {
protected String serverUrl = "https://create.reify.com";
protected String workdir;
protected String webappDir = "war";
protected String smartclientRuntimeDir;
protected boolean includeTestJsp;
protected String testJspPathname;
protected boolean includeTestHtml;
protected String testHtmlPathname;
protected String dataSourcesDir = "WEB-INF/ds";
protected String mockDataSourcesDir = "WEB-INF/ds/mock";
protected boolean skipValidationOnImport;
protected Severity validationFailureThreshold = Severity.ERROR;
protected String uiDir = "WEB-INF/ui";
protected String projectFileDir = "WEB-INF/ui";
protected boolean modifyWelcomeFiles;
protected boolean drawOnWelcomeFiles;
protected String projectName;
protected String projectFileName;
protected String zipFileName;
protected String username;
protected String password;
@Override
public void execute() throws BuildException {
ImportMojo mojo = new ImportMojo();
mojo.setLog(new AntProjectLogger(getProject()));
if (username == null || password == null) {
throw new BuildException("Username and password parameters required.");
}
if (projectName == null) {
projectName = getProject().getName();
}
mojo.setHost(String.valueOf(serverUrl));
mojo.setCredentials(new UsernamePasswordCredentials(username, password));
mojo.setWorkdir(workdir != null ? new File(workdir) : new File(getProject().getBaseDir(), "build/reify"));
mojo.setWebappDir(webappDir != null ? new File(webappDir) : new File(getProject().getBaseDir(), webappDir));
mojo.setSmartclientRuntimeDir(smartclientRuntimeDir != null ? new File(smartclientRuntimeDir) : new File(getProject().getBaseDir(), "war/isomorphic"));
mojo.setIncludeTestJsp(includeTestJsp);
mojo.setTestJspPathname(testJspPathname = testJspPathname != null ? testJspPathname : projectName + ".run.jsp");
mojo.setIncludeTestHtml(includeTestHtml);
mojo.setTestHtmlPathname(testHtmlPathname = testHtmlPathname != null ? testHtmlPathname : projectName + ".run.html");
mojo.setDataSourcesDir(dataSourcesDir);
mojo.setMockDataSourcesDir(mockDataSourcesDir);
mojo.setSkipValidationOnImport(skipValidationOnImport);
mojo.setValidationFailureThreshold(validationFailureThreshold);
mojo.setUiDir(uiDir);
mojo.setProjectFileDir(projectFileDir);
mojo.setModifyWelcomeFiles(modifyWelcomeFiles);
mojo.setDrawOnWelcomeFiles(drawOnWelcomeFiles);
mojo.setProjectName(projectName);
mojo.setProjectFileName(projectFileName != null ? projectFileName : projectName + "proj.xml");
mojo.setZipFileName(zipFileName != null ? zipFileName : projectName + "proj.zip");
String proxyHost = System.getProperty("http.proxyHost");
String proxyPort = System.getProperty("http.proxyPort");
String proxyUser = System.getProperty("http.proxyUser");
String proxyPassword = System.getProperty("http.proxyPassword");
//undocumented by Ant, allowed / expected by Maven so allow it here
String proxyBypass = System.getProperty("http.nonProxyHosts");
if (proxyHost != null || proxyPort != null) {
Proxy p = new Proxy();
p.setHost(proxyHost != null ? proxyHost : "");
p.setPort(Integer.valueOf(proxyPort != null ? proxyPort : "8080"));
p.setUsername(proxyUser != null ? proxyUser : "");
p.setPassword(proxyPassword != null ? proxyPassword : "");
p.setNonProxyHosts(proxyBypass != null ? proxyBypass : "localhost");
p.setActive(true);
mojo.setProxy(p);
}
try {
mojo.execute();
} catch (Exception e) {
throw new BuildException(e);
}
}
/**
* Change the default value of https://create.reify.com.
* @param serverUrl the new value
* @see ImportMojo#serverUrl
*/
public void setServerUrl(String serverUrl) {
this.serverUrl = serverUrl;
}
/**
* Change the default value of ${basedir}/build/reify.
* @param workdir the new value
* @see ImportMojo#workdir
*/
public void setWorkdir(String workdir) {
this.workdir = workdir;
}
/**
* Change the default value of ${basedir}/war.
* @param webappDir the new value
* @see ImportMojo#webappDir
*/
public void setWebappDir(String webappDir) {
this.webappDir = webappDir;
}
/**
* Change the default value of ${basedir}/war/isomorphic.
* @param smartclientRuntimeDir the new value
* @see ImportMojo#smartclientRuntimeDir
*/
public void setSmartclientRuntimeDir(String smartclientRuntimeDir) {
this.smartclientRuntimeDir = smartclientRuntimeDir;
}
/**
* Change the default value of false.
* @param includeTestJsp the new value
* @see ImportMojo#includeTestJsp
*/
public void setIncludeTestJsp(boolean includeTestJsp) {
this.includeTestJsp = includeTestJsp;
}
/**
* Change the default value of {@link #projectName}.run.jsp.
* @param testJspPathname the new value
* @see ImportMojo#testJspPathname
*/
public void setTestJspPathname(String testJspPathname) {
this.testJspPathname = testJspPathname;
}
/**
* Change the default value of false.
* @param includeTestHtml the new value
* @see ImportMojo#includeTestHtml
*/
public void setIncludeTestHtml(boolean includeTestHtml) {
this.includeTestHtml = includeTestHtml;
}
/**
* Change the default value of {@link #projectName}.run.html.
* @param testHtmlPathname the new value
* @see ImportMojo#testHtmlPathname
*/
public void setTestHtmlPathname(String testHtmlPathname) {
this.testHtmlPathname = testHtmlPathname;
}
/**
* Change the default value of WEB-INF/ds.
* @param dataSourcesDir the new value
* @see ImportMojo#dataSourcesDir
*/
public void setDataSourcesDir(String dataSourcesDir) {
this.dataSourcesDir = dataSourcesDir;
}
/**
* Change the default value of {@link #dataSourcesDir}/mock.
* @param mockDataSourcesDir the new value
* @see ImportMojo#mockDataSourcesDir
*/
public void setMockDataSourcesDir(String mockDataSourcesDir) {
this.mockDataSourcesDir = mockDataSourcesDir;
}
/**
* Change the default value of false.
* @param skipValidationOnImport the new value
* @see ImportMojo#skipValidationOnImport
*/
public void setSkipValidationOnImport(boolean skipValidationOnImport) {
this.skipValidationOnImport = skipValidationOnImport;
}
/**
* Change the default value of ERROR.
* Other legal values include INFO and WARN.
* @param validationFailureThreshold the new value
* @see ImportMojo#validationFailureThreshold
*/
public void setValidationFailureThreshold(Severity validationFailureThreshold) {
this.validationFailureThreshold = validationFailureThreshold;
}
/**
* Change the default value of WEB-INF/ui.
* @param uiDir the new value
* @see ImportMojo#uiDir
*/
public void setUiDir(String uiDir) {
this.uiDir = uiDir;
}
/**
* Change the default value of WEB-INF/ui.
* @param projectFileDir the new value
* @see ImportMojo#projectFileDir
*/
public void setProjectFileDir(String projectFileDir) {
this.projectFileDir = projectFileDir;
}
/**
* Change the default value of false.
* @param modifyWelcomeFiles the new value
* @see ImportMojo#modifyWelcomeFiles
*/
public void setModifyWelcomeFiles(boolean modifyWelcomeFiles) {
this.modifyWelcomeFiles = modifyWelcomeFiles;
}
/**
* Change the default value of false.
* @param drawOnWelcomeFiles the new value
* @see ImportMojo#drawOnWelcomeFiles
*/
public void setDrawOnWelcomeFiles(boolean drawOnWelcomeFiles) {
this.drawOnWelcomeFiles = drawOnWelcomeFiles;
}
/**
* Change the default value of ${ant.project.name}.
* @param projectName the new value
* @see ImportMojo#projectName
*/
public void setProjectName(String projectName) {
this.projectName = projectName;
}
/**
* Set the username needed to authenticate to
* @param username the new value
*/
public void setUsername(String username) {
this.username = username;
}
/**
* Set the password needed to authenticate to
* @param password the new value
*/
public void setPassword(String password) {
this.password = password;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy