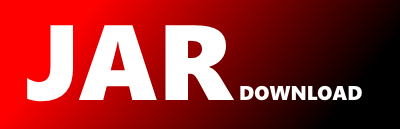
com.itangcent.intellij.jvm.scala.ScKit.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of intellij-scala-support Show documentation
Show all versions of intellij-scala-support Show documentation
Help for developing plugins for JetBrains products.
support kotlin feature
The newest version!
package com.itangcent.intellij.jvm.scala
import scala.Option
import scala.util.Either
import java.util.*
@Suppress("UNCHECKED_CAST")
fun Any?.getOrNull(): T? {
try {
when {
this == null -> return null
this is Option<*> -> return if (this.isDefined) {
return this.get() as? T
} else null
this is Optional<*> -> return if (this.isPresent) {
return this.get() as? T
} else null
this is Either<*, *> -> return if (this.isRight) {
return this.right().get() as? T
} else null
else -> return this as? T?
}
} catch (e: Throwable) {
return null
}
}
fun Any.castToList(): List {
(this as? scala.collection.Iterable<*>)?.toKtList()?.filterNotNull()?.let { return it }
(this as? scala.collection.Iterator<*>)?.toKtList()?.filterNotNull()?.let { return it }
(this as? Iterator<*>)?.toList()?.filterNotNull()?.let { return it }
(this as? Iterable<*>)?.toList()?.filterNotNull()?.let { return it }
return emptyList()
}
@Suppress("UNCHECKED_CAST")
fun Any.castToTypedList(): List {
(this as? scala.collection.Iterable)?.toKtList()?.filterNotNull()?.let { return it }
(this as? scala.collection.Iterator)?.toKtList()?.let { return it }
(this as? Iterator)?.toList()?.filterNotNull()?.let { return it }
(this as? Iterable)?.toList()?.filterNotNull()?.let { return it }
return emptyList()
}
private fun Iterator.toList(): List {
val result = LinkedList()
this.forEach { result.add(it) }
return result
}
fun scala.collection.Iterable.toKtList(): List {
val result = LinkedList()
for (r in this) {
result.add(r)
}
return result
}
fun scala.collection.Iterator.toKtList(): List {
val result = LinkedList()
this.forall { result.add(it) }
return result
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy