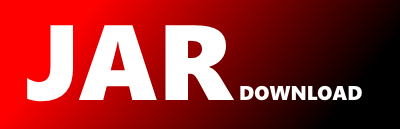
com.itcoon.common.jpa.page.JpaPageableSupplier Maven / Gradle / Ivy
package com.itcoon.common.jpa.page;
import com.itcoon.common.core.page.AbstractSortPageRequest;
import com.itcoon.common.core.page.ISortPage;
import com.itcoon.common.exception.ex.Assertion;
import com.itcoon.common.exception.ex.CommonResponseEnum;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.data.domain.Sort;
import java.util.function.Supplier;
public class JpaPageableSupplier extends AbstractSortPageRequest implements Supplier {
private static final String SORT_DIRECTIONS_PROPERTY_NAME = "sortDirections";
private static final String SORT_PROPERTIES_PROPERTY_NAME = "sortProperties";
private static final String DEFAULT_SORT_PROPERTY = "updateTime";
private static final Integer DEFAULT_FIND_LIMIT = 2000;
private static final Sort DEFAULT_SORT = Sort.by(Sort.Direction.DESC, DEFAULT_SORT_PROPERTY);
public static JpaPageableSupplier of(int pageNo, int pageSize, Integer[] sortDirections, String[] sortProperties){
return new JpaPageableSupplier(pageNo, pageSize, sortDirections, sortProperties);
}
public static JpaPageableSupplier of(ISortPage sortPage){
return new JpaPageableSupplier(sortPage);
}
private JpaPageableSupplier(ISortPage sortPage) {
super(sortPage);
}
private JpaPageableSupplier(int pageNo, int pageSize, Integer[] sortDirections, String[] sortProperties) {
super(pageNo, pageSize, sortDirections, sortProperties);
}
private Sort getSort() {
Sort sort = DEFAULT_SORT;
Integer[] sortDirections = getSortDirections();
String[] sortProperties = getSortProperties();
if(sortProperties!=null && sortDirections != null && sortProperties.length > 0){
Assertion.assertTrue(sortProperties.length == sortDirections.length).raise(CommonResponseEnum.PARAMETER_VALID_FAILURE,SORT_PROPERTIES_PROPERTY_NAME, SORT_DIRECTIONS_PROPERTY_NAME);
Sort.Order[] orders = new Sort.Order[sortProperties.length];
for (int i = 0; i < sortProperties.length; i++) {
Assertion.assertTrue(sortProperties[i] != null && sortDirections[i] != null).raise(CommonResponseEnum.PARAMETER_VALID_FAILURE,SORT_PROPERTIES_PROPERTY_NAME, SORT_DIRECTIONS_PROPERTY_NAME);
Assertion.assertTrue(sortDirections[i] < 3 && sortDirections[i] > 0).raise(CommonResponseEnum.PARAMETER_VALID_FAILURE,SORT_PROPERTIES_PROPERTY_NAME, SORT_DIRECTIONS_PROPERTY_NAME);
orders[i] = sortDirections[i] == 1 ? Sort.Order.asc(sortProperties[i]) : Sort.Order.desc(sortProperties[i]);
}
sort = Sort.by(orders);
}
return sort;
}
@Override
public Pageable get() {
Integer pageNo = getPageNo();
Integer pageSize = getPageSize();
Assertion.assertNotNull(pageNo).raise(CommonResponseEnum.CUSTOM_MESSAGE_EXCEPTION, "[Assertion Failure] - Parameter#PageNo is required, it must be not null.");
Assertion.assertNotNull(pageSize).raise(CommonResponseEnum.CUSTOM_MESSAGE_EXCEPTION, "[Assertion Failure] - Parameter#PageSize is required, it must be not null.");
Sort sort = getSort();
Pageable pageable = PageRequest.of(pageNo, pageSize, sort);
if(pageNo == 0){
pageable = PageRequest.of(pageNo, pageSize == 0 ? DEFAULT_FIND_LIMIT : pageSize, sort);
}
return pageable;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy