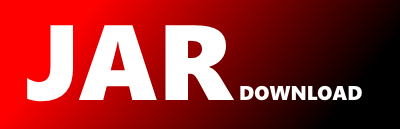
com.iteaj.iot.client.codec.WebSocketClientDecoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iot-client Show documentation
Show all versions of iot-client Show documentation
基于netty的tcp, http, udp等协议的客户端
The newest version!
package com.iteaj.iot.client.codec;
import com.iteaj.iot.Message;
import com.iteaj.iot.SocketMessage;
import com.iteaj.iot.client.websocket.WebSocketClientComponent;
import com.iteaj.iot.client.websocket.WebSocketClientMessage;
import com.iteaj.iot.codec.SocketMessageDecoder;
import com.iteaj.iot.codec.adapter.SocketMessageDecoderDelegation;
import com.iteaj.iot.websocket.WebSocketConnectHead;
import com.iteaj.iot.websocket.WebSocketException;
import com.iteaj.iot.websocket.WebSocketFrameType;
import com.iteaj.iot.websocket.WebSocketMessage;
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.handler.codec.DecoderResult;
import io.netty.handler.codec.http.HttpResponse;
import io.netty.handler.codec.http.websocketx.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class WebSocketClientDecoder extends SimpleChannelInboundHandler
© 2015 - 2025 Weber Informatics LLC | Privacy Policy