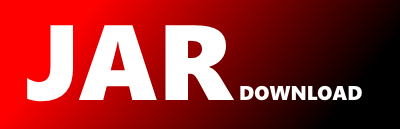
com.itemis.maven.plugins.artifact.spy.ArtifactSpyMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of artifact-spy-plugin Show documentation
Show all versions of artifact-spy-plugin Show documentation
A small Maven plugin that spies out all the artifacts that are produced by a Maven build.
package com.itemis.maven.plugins.artifact.spy;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.Properties;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Component;
import org.apache.maven.plugins.annotations.LifecyclePhase;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
/**
* A small Mojo that only detects the artifacts produced by the build of the current project and writes them out to a
* properties file.
* The Mojo should be bound to a late build phase in order to detect all artifacts produced by the build. By default it
* is bound to the verify phase.
*
*
* The properties will have the following format:
*
* - key: artifact coordinates
* - value: filepath of the artifact (relative to the project's base directory)
*
*
* @author Stanley Hillner
* @since 1.0.0
*/
@Mojo(name = "spy", defaultPhase = LifecyclePhase.VERIFY)
public class ArtifactSpyMojo extends AbstractMojo {
@Component
private MavenProject project;
/**
* The path of the properties file which will then contain all artifacts that have been produced by the build of the
* current project.
*/
@Parameter(required = true, defaultValue = "${project.build.directory}/artifact-spy/artifacts.properties")
private File outputFile;
public void execute() throws MojoExecutionException, MojoFailureException {
Properties props = new Properties();
Artifact projectArtifact = this.project.getArtifact();
if ("pom".equals(projectArtifact.getType())) {
projectArtifact.setFile(this.project.getFile());
}
props.setProperty(projectArtifact.toString(), getProjectRelativePath(projectArtifact.getFile()));
for (Artifact a : this.project.getAttachedArtifacts()) {
props.setProperty(a.toString(), getProjectRelativePath(a.getFile()));
}
FileOutputStream fos = null;
try {
this.outputFile.getParentFile().mkdirs();
fos = new FileOutputStream(this.outputFile);
props.store(fos, "");
} catch (IOException e) {
throw new MojoFailureException(
"Error serializing the project artifacts to file: " + this.outputFile.getAbsolutePath(), e);
} finally {
try {
fos.close();
} catch (IOException e) {
throw new MojoExecutionException("Could not close output stream after writing project artifacts to file: "
+ this.outputFile.getAbsolutePath(), e);
}
}
}
private String getProjectRelativePath(File f) {
return this.project.getBasedir().toURI().relativize(f.toURI()).getPath();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy