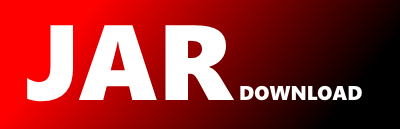
com.itemis.maven.plugins.unleash.scm.requests.CheckoutRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unleash-scm-provider-api Show documentation
Show all versions of unleash-scm-provider-api Show documentation
The API used by the Unleash Maven Plugin to talk to different SCM providers.
package com.itemis.maven.plugins.unleash.scm.requests;
import java.util.Set;
import com.google.common.base.Optional;
import com.google.common.base.Preconditions;
import com.google.common.collect.Sets;
/**
* A Request for the checking out a remote repository into the local working directory. In case of distributed SCMs this
* is equal to cloning the repository.
* USE {@link #builder()} TO CREATE A REQUEST!
*
* The following configuration options are possible:
*
* - Specify a repository base URL in conjunction with a branch or tag name.
* - Specify the full repository URL including the branch or tag name if this information can be included in the URL.
*
* - Checkout only a few files from the repository by setting the repository-relative paths of these files or
* directories.
*
*
* @author Stanley Hillner
* @since 0.1.0
*/
public class CheckoutRequest {
private String remoteRepositoryUrl;
private String revision;
private String branch;
private String tag;
private Set pathsToCheckout;
private CheckoutRequest() {
this.pathsToCheckout = Sets.newHashSet();
// use builder!
}
public static Builder builder() {
return new Builder();
}
public String getRemoteRepositoryUrl() {
return this.remoteRepositoryUrl;
}
public Optional getRevision() {
return Optional.fromNullable(this.revision);
}
public Optional getBranch() {
return Optional.fromNullable(this.branch);
}
public Optional getTag() {
return Optional.fromNullable(this.tag);
}
public Set getPathsToCheckout() {
return this.pathsToCheckout;
}
public boolean checkoutWholeRepository() {
return this.pathsToCheckout.isEmpty();
}
public boolean checkoutBranch() {
return this.branch != null;
}
public boolean checkoutTag() {
return this.tag != null;
}
/**
* The builder for a {@link CheckoutRequest}.
*
* @author Stanley Hillner
* @since 0.1.0
*/
public static class Builder {
private CheckoutRequest request = new CheckoutRequest();
/**
* @param remoteRepositoryUrl the URL of the remote repository to check out (mandatory).
* @return the builder itself.
*/
public Builder from(String remoteRepositoryUrl) {
this.request.remoteRepositoryUrl = remoteRepositoryUrl;
return this;
}
/**
* @param revision the revision of the repository branch to checkout. If this revision is omitted, HEAD is assumed.
* @return the builder itself.
*/
public Builder revision(String revision) {
this.request.revision = revision;
return this;
}
/**
* Sets the name of the branch from which the SCM provider shall checkout.
* Setting this parameter makes only sense if the remote URL links to the repository base, not a specific branch.
*
*
* This parameter excludes the usage of {@link #tag(String)} and unsets the tag parameter value.
*
* @param branchName the name of the branch to checkout.
* @return the builder itself.
*/
public Builder branch(String branchName) {
this.request.branch = branchName;
this.request.tag = null;
return this;
}
/**
* Sets the name of the tag from which the SCM provider shall checkout.
* Setting this parameter makes only sense if the remote URL links to the repository base, not a specific branch.
*
*
* This parameter excludes the usage of {@link #branch(String)} and unsets the branch parameter value.
*
* @param tagName the name of the tag to checkout.
* @return the builder itself.
*/
public Builder tag(String tagName) {
this.request.tag = tagName;
this.request.branch = null;
return this;
}
/**
* Adds some repository-relative paths of files or folders to the list of paths to checkout.
* Once some paths are added only these files are checked out, nothing else!
* You can use {@link #paths(null)} to unset the list of files and checkout the whole repository.
*
* @param paths some filepaths to checkout.
* @return the builder itself.
*/
public Builder addPaths(String... paths) {
for (String path : paths) {
this.request.pathsToCheckout.add(path);
}
return this;
}
/**
* Sets the repository-relative paths of files or folders to checkout. This method totally overrides all paths added
* previously!
* Once some paths are added only these files are checked out, nothing else!
* Use {@link #paths(null)} to unset the list of files and checkout the whole repository.
*
* @param paths the filepaths to checkout.
* @return the builder itself.
*/
public Builder paths(Set paths) {
if (paths != null) {
this.request.pathsToCheckout = paths;
} else {
this.request.pathsToCheckout = Sets.newHashSet();
}
return this;
}
/**
* Checks the settings of the request to build and builds the actual checkout request.
*
* @return the request for checking out the repository.
*/
public CheckoutRequest build() {
Preconditions.checkState(this.request.getRemoteRepositoryUrl() != null, "No remote repository URL specified!");
return this.request;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy