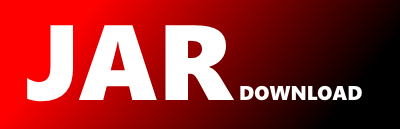
org.jsoup.helper.DescendableLinkedList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of html2pdf Show documentation
Show all versions of html2pdf Show documentation
pdfHTML is an iText add-on that lets you to parse (X)HTML snippets and the associated CSS and converts
them to PDF.
package org.jsoup.helper;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.ListIterator;
/**
* Provides a descending iterator and other 1.6 methods to allow support on the 1.5 JRE.
* @param Type of elements
*/
public class DescendableLinkedList extends LinkedList {
/**
* Create a new DescendableLinkedList.
*/
public DescendableLinkedList() {
super();
}
/**
* Add a new element to the start of the list.
* @param e element to add
*/
public void push(E e) {
addFirst(e);
}
/**
* Look at the last element, if there is one.
* @return the last element, or null
*/
public E peekLast() {
return size() == 0 ? null : getLast();
}
/**
* Remove and return the last element, if there is one
* @return the last element, or null
*/
public E pollLast() {
return size() == 0 ? null : removeLast();
}
/**
* Get an iterator that starts and the end of the list and works towards the start.
* @return an iterator that starts and the end of the list and works towards the start.
*/
public Iterator descendingIterator() {
return new DescendingIterator(size());
}
private class DescendingIterator implements Iterator {
private final ListIterator iter;
@SuppressWarnings("unchecked")
private DescendingIterator(int index) {
iter = (ListIterator) listIterator(index);
}
/**
* Check if there is another element on the list.
* @return if another element
*/
public boolean hasNext() {
return iter.hasPrevious();
}
/**
* Get the next element.
* @return the next element.
*/
public E next() {
return iter.previous();
}
/**
* Remove the current element.
*/
public void remove() {
iter.remove();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy