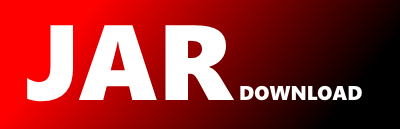
com.j2bugzilla.rpc.BugSearch Maven / Gradle / Ivy
/*
* Copyright 2011 Thomas Golden
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.j2bugzilla.rpc;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.j2bugzilla.base.Bug;
import com.j2bugzilla.base.BugFactory;
import com.j2bugzilla.base.BugzillaMethod;
/**
* This class provides convenience methods for searching for {@link Bug Bugs} on your installation.
*
* Clients should note that Bugzilla does not seem to respond well when returning large numbers of
* search results via the webservice interface (around 2000 in some tests caused it to fail with an HTTP 500 error).
* Try and keep searches as focused as possible for best results.
*
* @author Tom
*
*/
public class BugSearch implements BugzillaMethod {
/**
* The method Bugzilla will execute via XML-RPC
*/
private static final String METHOD_NAME = "Bug.search";
/**
* A {@code Map} returned by the XML-RPC method.
*/
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy