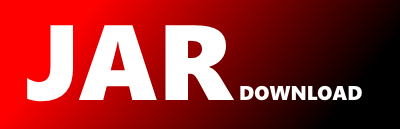
com.j2mvc.framework.config.Config Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of j2mvc-framework-web Show documentation
Show all versions of j2mvc-framework-web Show documentation
强烈建议使用J2mvc 2.1以后的版本。
version 2.1.01
1.优化路径跳转,Servlet和Filter方式的路径设置
2.优化内存销毁
3.更换JSON依赖包
4.优化接收方法RequestMethod,封装不同ContentType格式提交
封装JSON,XML数据提交模块
JSON请求示例
{
"id":"JSON134851",
"title":"JSON提交的标题",
"price":65.1,
"stock":100
}
XML请求示例
<!DOCTYPE root [
<!ELEMENT root ANY>
<!ATTLIST Product SSN ID #REQUIRED>]>
<root>
<Product SSN='id'>XMLID12354</Product>
<Product SSN='title'>XML提交的标题 </Product>
<Product SSN='price'>55 </Product>
<Product SSN='stock'>32 </Product>
</root>
version 2.1.02
1.解决URL无后缀情况无法加载静态资源,解决无法渲染CSS文件。
version 2.1.03
1.移除com.j2mvc.StringUtils.getUtf8()方法调用
更改为getCharset()
version 2.1.04
1.去除Servlet和Filter的全局变量销毁,只交给Listener处理。
version 2.1.05,2.1.06,2.1.07
1.完善POST提交的JSON数据
支持接收基础数据类型、任意对象类型、任意数组类型。
不支持接收参数为集合类型或Map类型,但可以定义为接收对象类型的元素。
version 2.1.05,2.1.06,2.1.07
1.修改连接池变量
version 2.1.09
增加上传功能,修改RequestMethod,ContentType设置方式
version 2.1.10,2.1.11
更改上传文件名格式为UUID格式,移除JSON映射类,更改接收多文件上传。
version 2.1.12
删除文件列有的空对象
version 2.1.13
增加配置文件目录/conf,加载上传功能配置/conf/upload.properties
version 2.1.18
拦截器也能获取ActionBean
version 2.1.20
添加上传文件只读权限
version 2.1.21
支持同时接收文件和文本数据
version 2.1.22
增加文件接收类型media
version 2.1.23
删除upload类printJson方法
package com.j2mvc.framework.config;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.apache.log4j.Logger;
import javax.servlet.ServletContext;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import com.j2mvc.framework.Session;
import com.j2mvc.framework.dao.DataSourceBean;
import com.j2mvc.framework.dao.DataSourceJndi;
import com.j2mvc.framework.i18n.I18n;
import com.j2mvc.framework.interceptor.DispatcherInterceptor;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXParseException;
/**
* 配置
*
* 2014-2-22 创建@杨朔
*/
public class Config {
static final Logger log = Logger.getLogger(Config.class);
private ServletContext context;
/** 主配置文件名 */
private String fileName;
/** properties 配置文件键值集合 */
public static Map> props = new HashMap>();
/** properties 配置文件键值集合 */
public static Map attributes = new HashMap();
/**
* 加载XML配置文件构造器
* @param context
* @param fileName
*/
public Config(ServletContext context,String fileName) {
this.context = context;
this.fileName = fileName;
// Properties配置导入
PropsConfig.init(context);
loadDom();
// 绑定数据源
DataSourceJndi.init();
}
/**
* 加载XML
* @param inputStream
*/
private void loadDom() {
try {
InputStream inputStream = new FileInputStream(new File(context.getRealPath("/")+fileName));
// 解析XML
DocumentBuilder parser;
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
try {
parser = factory.newDocumentBuilder();
log.info("解析配置文件:"+fileName+".");
Document doc = parser.parse(inputStream);
log.info("解析完成,读取文档Document.");
processConfDoc(doc);
} catch (ParserConfigurationException e) {
e.printStackTrace();
}catch (SAXParseException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
/**
* 解析XML
*/
private void processConfDoc(Document doc) {
Element rootElement = doc.getDocumentElement();
NodeList nodeList = rootElement.getChildNodes();
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
// 初始化Actions包节点
if (node.getNodeName().equals("actions-packages")) {
log.info("读取Action包节点:"+node.getNodeName()+".");
initActionsPackages(node);
}
// 初始化拦截器
if (node.getNodeName().equals("interceptors")) {
log.info("读取拦截器interceptors节点:"+node.getNodeName()+".");
initInterceptors((Element)node);
}
// 初始化数据源
if (node.getNodeName().equals("DataSource")) {
log.info("读取数据源DataSource节点:"+node.getNodeName()+".");
initDataSource((Element)node);
}
// 默认语种
if (node.getNodeName().equals("i18n-default")) {
log.info("读取默认语种i18n-default节点:"+node.getNodeName()+".");
String value = node.getTextContent()!=null?node.getTextContent().trim():"";
value = value.replaceAll(" ","").replaceAll("\r","").replaceAll("\n","").replaceAll("\t","");
value = getCharset(value);
if(!value.equals(""))
new I18n(context,"/conf/i18n/"+value+".properties");
}
}
}
/**
* 初始化拦截器
* @param element
*/
private void initInterceptors(Element parentElement) {
NodeList nodeList = parentElement.getChildNodes();
for(int i=0;i refClass;
try {
refClass = Class.forName(ref);
interceptor.setRefClass(refClass);
Session.interceptors.add(interceptor);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
}
/**
* 初始化数据源
* @param element
*/
private void initDataSource(Element element){
String maxActive = get(element,"maxActive").trim();
String maxIdle = get(element,"maxIdle").trim();
String maxWait = get(element,"maxWait").trim();
String initialSize = get(element,"initialSize").trim();
String name = get(element,"name");
String validationQuery = get(element,"validationQuery");
DataSourceBean dataSourceBean = new DataSourceBean();
dataSourceBean.setDriverClassName(get(element,"driverClassName"));
dataSourceBean.setMaxActive(maxActive.matches("\\d+")?Integer.valueOf(maxActive):0);
dataSourceBean.setMaxIdle(maxIdle.matches("\\d+")?Integer.valueOf(maxIdle):0);
dataSourceBean.setMaxWait(maxWait.matches("\\d+")?Long.valueOf(maxWait):0);
dataSourceBean.setInitialSize(initialSize.matches("\\d+")?Integer.valueOf(initialSize):0);
dataSourceBean.setName(name);
dataSourceBean.setPassword(get(element,"password"));
dataSourceBean.setUrl(get(element,"url"));
dataSourceBean.setUsername(get(element,"username"));
if(validationQuery!=null)
dataSourceBean.setValidationQuery(validationQuery);
Session.dataSourceBean = dataSourceBean;
Session.dataSourceBeanMap.put(name,dataSourceBean);
}
/**
* 初始化ActionsPackages
* @param element
*/
private void initActionsPackages(Node node){
String mValue = node.getTextContent()!=null?node.getTextContent().trim():"";
mValue = mValue.replaceAll(" ","").replaceAll("\r","").replaceAll("\n","").replaceAll("\t","");
String[] args = mValue.split(",");
Set set = new HashSet();
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy