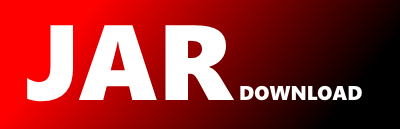
com.j2mvc.framework.dispatcher.reader.BaseReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of j2mvc-framework-web Show documentation
Show all versions of j2mvc-framework-web Show documentation
强烈建议使用J2mvc 2.1以后的版本。
version 2.1.01
1.优化路径跳转,Servlet和Filter方式的路径设置
2.优化内存销毁
3.更换JSON依赖包
4.优化接收方法RequestMethod,封装不同ContentType格式提交
封装JSON,XML数据提交模块
JSON请求示例
{
"id":"JSON134851",
"title":"JSON提交的标题",
"price":65.1,
"stock":100
}
XML请求示例
<!DOCTYPE root [
<!ELEMENT root ANY>
<!ATTLIST Product SSN ID #REQUIRED>]>
<root>
<Product SSN='id'>XMLID12354</Product>
<Product SSN='title'>XML提交的标题 </Product>
<Product SSN='price'>55 </Product>
<Product SSN='stock'>32 </Product>
</root>
version 2.1.02
1.解决URL无后缀情况无法加载静态资源,解决无法渲染CSS文件。
version 2.1.03
1.移除com.j2mvc.StringUtils.getUtf8()方法调用
更改为getCharset()
version 2.1.04
1.去除Servlet和Filter的全局变量销毁,只交给Listener处理。
version 2.1.05,2.1.06,2.1.07
1.完善POST提交的JSON数据
支持接收基础数据类型、任意对象类型、任意数组类型。
不支持接收参数为集合类型或Map类型,但可以定义为接收对象类型的元素。
version 2.1.05,2.1.06,2.1.07
1.修改连接池变量
version 2.1.09
增加上传功能,修改RequestMethod,ContentType设置方式
version 2.1.10,2.1.11
更改上传文件名格式为UUID格式,移除JSON映射类,更改接收多文件上传。
version 2.1.12
删除文件列有的空对象
version 2.1.13
增加配置文件目录/conf,加载上传功能配置/conf/upload.properties
version 2.1.18
拦截器也能获取ActionBean
version 2.1.20
添加上传文件只读权限
version 2.1.21
支持同时接收文件和文本数据
version 2.1.22
增加文件接收类型media
version 2.1.23
删除upload类printJson方法
The newest version!
package com.j2mvc.framework.dispatcher.reader;
import java.beans.IntrospectionException;
import java.beans.PropertyDescriptor;
import java.io.UnsupportedEncodingException;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import org.apache.log4j.Logger;
import org.w3c.dom.Document;
import com.alibaba.fastjson.JSONObject;
import com.j2mvc.framework.Session;
import com.j2mvc.framework.dao.DaoSupport;
import com.j2mvc.framework.mapping.Column;
import com.j2mvc.framework.mapping.Foreign;
import com.j2mvc.framework.mapping.PrimaryKey;
import com.j2mvc.framework.mapping.Sql;
import com.j2mvc.framework.util.FieldUtil;
import javassist.ClassClassPath;
import javassist.ClassPool;
import javassist.CtClass;
import javassist.CtMethod;
import javassist.Modifier;
import javassist.NotFoundException;
import javassist.bytecode.CodeAttribute;
import javassist.bytecode.LocalVariableAttribute;
import javassist.bytecode.MethodInfo;
/**
* 读取请求数据
*
* @author 杨朔
*
*/
public abstract class BaseReader {
static final Logger log = Logger.getLogger(BaseReader.class);
protected HttpServletRequest request;
// 当前返回Action对象
protected Object object;
// 当前方法
protected Method method;
// 当前class泛型对象
protected Class> clazz ;
// 参数类型数组
protected Class>[] types;
// 参数名称数组
protected String[] names;
// 参数值数组
protected Object[] values;
// 数据流字符串
protected String requestBody;
// JSON数据
protected JSONObject jsonData;
// XML数据
protected Document xmlData;
public BaseReader(HttpServletRequest request, Method method, Object object) {
super();
this.request = request;
this.method = method;
this.object = object;
}
/**
* 返回反射对象
* @return
*/
public abstract Object result();
/**
* 获取参数值
* @param type
* @param name
*/
protected abstract Object getParameterValue(Class> type,String name);
public JSONObject getJsonData() {
return jsonData;
}
public Document getXmlData() {
return xmlData;
}
public String getRequestBody() {
return requestBody;
}
/**
* 获取参数名
* @param methodName
* @param length
*
* @throws NotFoundException
*/
protected String[] getParameterNames(Class> clazz,String methodName,int length){
ClassPool pool = ClassPool.getDefault();
CtClass cc;
try {
// web下必须注册类路径,否则找不到类文件
pool.insertClassPath(new ClassClassPath(clazz));
cc = pool.getCtClass(clazz.getName());
CtMethod cm = cc.getDeclaredMethod(methodName);
// 使用javaassist的反射方法获取方法的参数名
MethodInfo methodInfo = cm.getMethodInfo();
CodeAttribute codeAttribute = methodInfo.getCodeAttribute();
LocalVariableAttribute attr = (LocalVariableAttribute) codeAttribute.getAttribute(LocalVariableAttribute.tag);
if (attr != null) {
// exception
String[] paramNames = new String[length];
int pos = Modifier.isStatic(cm.getModifiers()) ? 0 : 1;
for (int i = 0; i < length; i++) {
paramNames[i] = attr.variableName(i + pos);
}
return paramNames;
}
} catch (NotFoundException e) {
log.error(e.getMessage());
}
return null;
}
/**
* 查询指定主键实体对象
* @param fields
* @param clazz
*
*/
protected Object getObjectByPrimaryKey(List fields,Class> clazz){
/* 主键 */
PrimaryKey primaryKey = clazz.getAnnotation(PrimaryKey.class);
int size = fields.size();
for(int i=0;i fields,Object obj){
int size = fields.size();
for(int i=0;i type,String name);
/**
* 写入字段值
* @param field
* @param obj
* @param rs
*/
protected void invoke(Field field,Object obj) {
/* 注释字段,对应数据表字段 */
Column column = field.getAnnotation(Column.class);
/* 注释字段,对应关联对象字段 */
Foreign foreign = field.getAnnotation(Foreign.class);
/* 注释字段,根据SQL语句查询值,仅返回基础数据类型 */
Sql sql = field.getAnnotation(Sql.class);
if(column == null){
return;
}
String name = field.getName();
/* 数据表数据类型 */
Class> type = field.getType();
try {
Object value = null;
if(foreign!=null){
// 外键对象之主键属性数据类型
type = FieldUtil.getForeignKey(type).getType();
}
value = getValue(type,name);
if(foreign!=null){
// 外键,字段为对象,获取类主键,并实例
try {
DaoSupport daoSupport = new DaoSupport(field.getType());
value = daoSupport.get(value);
} catch (Exception e) {
log.error(e.getMessage());
}
}else if(sql!=null){
// 根据SQL语句查询值
try {
DaoSupport daoSupport = new DaoSupport(field.getType());
value = daoSupport.get(sql.value());
} catch (Exception e) {
log.error(e.getMessage());
}
}
// 执行写入操作
PropertyDescriptor pd = new PropertyDescriptor(name, obj.getClass());
Method wm = pd.getWriteMethod();
if(value!=null && !value.equals(""))
wm.invoke(obj, value);
} catch (IntrospectionException e) {
log.error(e.getMessage());
}catch (IllegalArgumentException e) {
log.error(e.getMessage());
}catch (IllegalAccessException e) {
log.error(e.getMessage());
}catch (InvocationTargetException e) {
log.error(e.getMessage());
}
}
/**
* 获取UTF8格式
* @param value
*
*/
protected String getCharset(String value){
if(value == null)
return null;
try {
if(java.nio.charset.Charset.forName("ISO-8859-1").newEncoder().canEncode(value)){
value = new String(value.getBytes("ISO-8859-1"),Session.encoding);
}else if(java.nio.charset.Charset.forName("UTF-8").newEncoder().canEncode(value)){
}else if(java.nio.charset.Charset.forName("GBK").newEncoder().canEncode(value)){
value = new String(value.getBytes("GBK"),"UTF-8");
}else if(java.nio.charset.Charset.forName("GB2312").newEncoder().canEncode(value)){
value = new String(value.getBytes("GB2312"),"UTF-8");
}
} catch (UnsupportedEncodingException e) {
}
return value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy