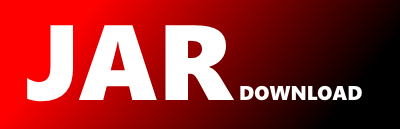
com.jaeksoft.searchlib.render.AbstractRenderDocumentsXml Maven / Gradle / Ivy
/**
* License Agreement for OpenSearchServer
*
* Copyright (C) 2012 Emmanuel Keller / Jaeksoft
*
* http://www.open-search-server.com
*
* This file is part of OpenSearchServer.
*
* OpenSearchServer is free software: you can redistribute it and/or
* modify it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* OpenSearchServer is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with OpenSearchServer.
* If not, see .
**/
package com.jaeksoft.searchlib.render;
import java.io.IOException;
import java.util.List;
import java.util.Map;
import org.apache.commons.collections.CollectionUtils;
import com.jaeksoft.searchlib.SearchLibException;
import com.jaeksoft.searchlib.function.expression.SyntaxError;
import com.jaeksoft.searchlib.query.ParseException;
import com.jaeksoft.searchlib.request.AbstractRequest;
import com.jaeksoft.searchlib.request.AbstractSearchRequest;
import com.jaeksoft.searchlib.request.RequestInterfaces;
import com.jaeksoft.searchlib.request.ReturnField;
import com.jaeksoft.searchlib.request.ReturnFieldList;
import com.jaeksoft.searchlib.result.AbstractResult;
import com.jaeksoft.searchlib.result.ResultDocument;
import com.jaeksoft.searchlib.result.ResultDocumentsInterface;
import com.jaeksoft.searchlib.schema.FieldValue;
import com.jaeksoft.searchlib.schema.FieldValueItem;
import com.jaeksoft.searchlib.snippet.SnippetField;
import com.jaeksoft.searchlib.snippet.SnippetFieldList;
import com.jaeksoft.searchlib.snippet.SnippetFieldValue;
public abstract class AbstractRenderDocumentsXml>
extends AbstractRenderXml {
private final ResultDocumentsInterface> resultDocs;
protected AbstractRenderDocumentsXml(T2 result) {
super(result);
resultDocs = result instanceof ResultDocumentsInterface ? (ResultDocumentsInterface>) result
: null;
}
final protected void renderDocuments() throws IOException, ParseException,
SyntaxError, SearchLibException {
int start = resultDocs.getRequestStart();
int end = resultDocs.getDocumentCount() + start;
writer.print("");
for (int i = start; i < end; i++) {
ResultDocument doc = resultDocs.getDocument(i, renderingTimer);
this.renderDocument(i, doc);
}
writer.println(" ");
}
final protected void renderDocument(AbstractRequest abstractRequest,
ResultDocument doc) throws IOException {
if (doc == null)
return;
ReturnFieldList returnFieldList = null;
SnippetFieldList snippetFieldList = null;
if (abstractRequest instanceof AbstractSearchRequest) {
returnFieldList = ((AbstractSearchRequest) abstractRequest)
.getReturnFieldList();
snippetFieldList = ((AbstractSearchRequest) abstractRequest)
.getSnippetFieldList();
} else if (abstractRequest instanceof RequestInterfaces.ReturnedFieldInterface) {
returnFieldList = ((RequestInterfaces.ReturnedFieldInterface) abstractRequest)
.getReturnFieldList();
}
if (returnFieldList != null)
for (ReturnField field : returnFieldList)
renderField(doc, field.getName());
if (snippetFieldList != null)
for (SnippetField field : snippetFieldList)
renderSnippetValue(doc, field.getName());
}
final protected void renderDocument(ResultDocument doc) throws IOException {
Map returnFields = doc.getReturnFields();
if (returnFields != null)
for (String fieldName : returnFields.keySet())
renderField(doc, fieldName);
Map snippetFields = doc.getSnippetFields();
if (snippetFields != null)
for (String fieldName : snippetFields.keySet())
renderSnippetValue(doc, fieldName);
}
final protected void renderDocumentPrefix(int pos, ResultDocument doc) {
writer.print("\t");
}
final protected void renderDocumentSuffix() {
writer.println("\t ");
}
final protected void renderDocumentContent(int pos, ResultDocument doc)
throws SearchLibException, IOException {
renderDocument(request, doc);
int cc = resultDocs.getCollapseCount(pos);
if (cc > 0) {
writer.print("\t\t");
writer.print(cc);
writer.println(" ");
}
}
protected void renderDocument(int pos, ResultDocument doc)
throws IOException, ParseException, SyntaxError, SearchLibException {
renderDocumentPrefix(pos, doc);
renderDocumentContent(pos, doc);
renderDocumentSuffix();
}
private void renderField(ResultDocument doc, String fieldName)
throws IOException {
List values = doc.getValues(fieldName);
if (CollectionUtils.isEmpty(values)) {
writer.print("\t\t ");
return;
}
for (FieldValueItem v : values) {
writer.print("\t\t');
writer.print(xmlTextRender(v.getValue()));
writer.println(" ");
}
}
private void renderSnippetValue(ResultDocument doc, String fieldName)
throws IOException {
List snippets = doc.getSnippetValues(fieldName);
if (CollectionUtils.isEmpty(snippets)) {
writer.print("\t\t ");
return;
}
boolean highlighted = doc.isHighlighted(fieldName);
for (FieldValueItem snippet : snippets) {
writer.print("\t\t');
writer.print(xmlTextRender(snippet.getValue()));
writer.println("\t\t ");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy