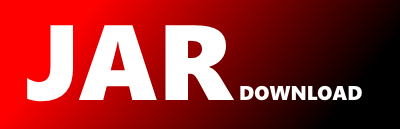
com.jaeksoft.searchlib.cache.FieldCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opensearchserver Show documentation
Show all versions of opensearchserver Show documentation
OpenSearchServer is a powerful, enterprise-class, search engine program. Using the web user interface,
the crawlers (web, file, database, ...) and the REST/RESTFul API you will be able to integrate quickly and
easily advanced full-text search capabilities in your application. OpenSearchServer runs on Windows and
Linux/Unix/BSD.
/**
* License Agreement for OpenSearchServer
*
* Copyright (C) 2008-2012 Emmanuel Keller / Jaeksoft
*
* http://www.open-search-server.com
*
* This file is part of OpenSearchServer.
*
* OpenSearchServer is free software: you can redistribute it and/or
* modify it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* OpenSearchServer is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with OpenSearchServer.
* If not, see .
**/
package com.jaeksoft.searchlib.cache;
import java.io.IOException;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import java.util.TreeSet;
import org.apache.lucene.document.Document;
import org.apache.lucene.document.Fieldable;
import org.apache.lucene.index.TermFreqVector;
import com.jaeksoft.searchlib.function.expression.SyntaxError;
import com.jaeksoft.searchlib.index.FieldCacheIndex;
import com.jaeksoft.searchlib.index.FieldContentCacheKey;
import com.jaeksoft.searchlib.index.IndexConfig;
import com.jaeksoft.searchlib.index.ReaderLocal;
import com.jaeksoft.searchlib.query.ParseException;
import com.jaeksoft.searchlib.schema.FieldValue;
import com.jaeksoft.searchlib.schema.FieldValueItem;
import com.jaeksoft.searchlib.schema.FieldValueOriginEnum;
import com.jaeksoft.searchlib.util.Timer;
public class FieldCache extends
LRUCache {
private IndexConfig indexConfig;
public FieldCache(IndexConfig indexConfig) {
super("Field cache", indexConfig.getFieldCache());
this.indexConfig = indexConfig;
}
final public Map get(final ReaderLocal reader,
final int docId, final Set fieldNameSet, final Timer timer)
throws IOException, ParseException, SyntaxError {
Map documentFields = new TreeMap();
Set storeField = new TreeSet();
Set vectorField = null;
Set indexedField = null;
Set missingField = null;
Timer t = new Timer(timer, "Field from cache");
// Getting available fields in the cache
for (String fieldName : fieldNameSet) {
FieldContentCacheKey key = new FieldContentCacheKey(fieldName,
docId);
FieldValueItem[] values = getAndPromote(key);
if (values != null)
documentFields
.put(fieldName, new FieldValue(fieldName, values));
else
storeField.add(fieldName);
}
t.end(null);
t = new Timer(timer, "Field from store");
// Check missing fields from store
if (storeField != null && storeField.size() > 0) {
vectorField = new TreeSet();
Document document = reader.getDocFields(docId, storeField);
for (String fieldName : storeField) {
Fieldable[] fieldables = document.getFieldables(fieldName);
if (fieldables != null && fieldables.length > 0) {
FieldValueItem[] valueItems = FieldValueItem
.buildArray(fieldables);
put(documentFields, fieldName, docId, valueItems);
} else
vectorField.add(fieldName);
}
}
t.end(null);
t = new Timer(timer, "Field from vector");
// Check missing fields from vector
if (vectorField != null && vectorField.size() > 0) {
indexedField = new TreeSet();
for (String fieldName : vectorField) {
TermFreqVector tfv = reader.getTermFreqVector(docId, fieldName);
if (tfv != null) {
FieldValueItem[] valueItems = FieldValueItem.buildArray(
FieldValueOriginEnum.TERM_VECTOR, tfv.getTerms());
put(documentFields, fieldName, docId, valueItems);
} else
indexedField.add(fieldName);
}
}
t.end(null);
t = new Timer(timer, "Field from StringIndex");
// Check missing fields from StringIndex
if (indexedField != null && indexedField.size() > 0) {
missingField = new TreeSet();
for (String fieldName : indexedField) {
FieldCacheIndex stringIndex = reader.getStringIndex(fieldName);
if (stringIndex != null) {
String term = stringIndex.lookup[stringIndex.order[docId]];
if (term != null) {
FieldValueItem[] valueItems = FieldValueItem
.buildArray(FieldValueOriginEnum.STRING_INDEX,
term);
put(documentFields, fieldName, docId, valueItems);
continue;
}
}
missingField.add(fieldName);
}
}
t.end(null);
if (missingField != null && missingField.size() > 0)
for (String fieldName : missingField)
documentFields.put(fieldName, new FieldValue(fieldName));
return documentFields;
}
final private void put(final Map documentFields,
final String fieldName, final int docId,
final FieldValueItem[] valueItems) {
documentFields.put(fieldName, new FieldValue(fieldName, valueItems));
FieldContentCacheKey key = new FieldContentCacheKey(fieldName, docId);
put(key, valueItems);
}
@Override
public void setMaxSize(int newMaxSize) {
super.setMaxSize(newMaxSize);
indexConfig.setFieldCache(newMaxSize);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy