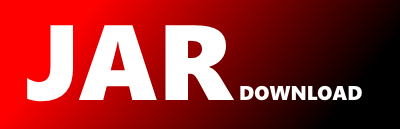
com.jaeksoft.searchlib.ClientFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opensearchserver Show documentation
Show all versions of opensearchserver Show documentation
OpenSearchServer is a powerful, enterprise-class, search engine program. Using the web user interface,
the crawlers (web, file, database, ...) and the REST/RESTFul API you will be able to integrate quickly and
easily advanced full-text search capabilities in your application. OpenSearchServer runs on Windows and
Linux/Unix/BSD.
/**
* License Agreement for OpenSearchServer
*
* Copyright (C) 2010-2013 Emmanuel Keller / Jaeksoft
*
* http://www.open-search-server.com
*
* This file is part of OpenSearchServer.
*
* OpenSearchServer is free software: you can redistribute it and/or
* modify it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* OpenSearchServer is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with OpenSearchServer.
* If not, see .
**/
package com.jaeksoft.searchlib;
import java.io.File;
import java.io.IOException;
import java.net.URISyntaxException;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.xpath.XPathExpressionException;
import org.apache.lucene.search.BooleanQuery;
import org.xml.sax.SAXException;
import com.jaeksoft.searchlib.crawler.web.browser.BrowserDriverEnum;
import com.jaeksoft.searchlib.util.FileUtils;
import com.jaeksoft.searchlib.util.Sequence;
import com.jaeksoft.searchlib.util.properties.PropertyItem;
import com.jaeksoft.searchlib.util.properties.PropertyItemListener;
import com.jaeksoft.searchlib.util.properties.PropertyManager;
import com.jaeksoft.searchlib.web.StartStopListener;
public class ClientFactory implements PropertyItemListener {
public static ClientFactory INSTANCE = null;
public final InstanceProperties properties;
private final Sequence globalSequence;
private PropertyItem booleanQueryMaxClauseCount;
private PropertyItem defaultWebBrowserDriver;
private PropertyItem soapActive;
private PropertyItem externalParser;
private PropertyItem logFullTrace;
private PropertyItem smtpHostname;
private PropertyItem smtpPort;
private PropertyItem smtpUseSsl;
private PropertyItem smtpUseTls;
private PropertyItem smtpSenderEmail;
private PropertyItem smtpSenderName;
private PropertyItem smtpUsername;
private PropertyItem smtpPassword;
private PropertyItem schedulerThreadPoolSize;
private PropertyItem clusterInstanceId;
private PropertyManager advancedProperties;
public ClientFactory() throws SearchLibException {
try {
globalSequence = new Sequence(new File(
StartStopListener.OPENSEARCHSERVER_DATA_FILE,
"globalSequence.txt"), 36);
properties = new InstanceProperties(new File(
StartStopListener.OPENSEARCHSERVER_DATA_FILE,
"properties.xml"));
File advPropFile = new File(
StartStopListener.OPENSEARCHSERVER_DATA_FILE,
"advanced.xml");
advancedProperties = new PropertyManager(advPropFile);
booleanQueryMaxClauseCount = advancedProperties.newIntegerProperty(
"booleanQueryMaxClauseCount", 1024, null, null);
hasBeenSet(booleanQueryMaxClauseCount);
booleanQueryMaxClauseCount.addListener(this);
defaultWebBrowserDriver = advancedProperties.newStringProperty(
"defaultWebBrowserDriver",
BrowserDriverEnum.FIREFOX.getName());
defaultWebBrowserDriver.addListener(this);
soapActive = advancedProperties.newBooleanProperty("soapActive",
false);
soapActive.addListener(this);
externalParser = advancedProperties.newBooleanProperty(
"externalParser", false);
externalParser.addListener(this);
logFullTrace = advancedProperties.newBooleanProperty(
"logFullTrace", false);
hasBeenSet(logFullTrace);
logFullTrace.addListener(this);
smtpHostname = advancedProperties.newStringProperty("smtpHostname",
"localhost");
smtpHostname.addListener(this);
smtpPort = advancedProperties.newIntegerProperty("smtpPort", 25, 1,
65536);
smtpPort.addListener(this);
smtpUseSsl = advancedProperties.newBooleanProperty("smtpUseSsl",
false);
smtpUseSsl.addListener(this);
smtpUseTls = advancedProperties.newBooleanProperty("smtpUseTls",
false);
smtpUseTls.addListener(this);
smtpSenderEmail = advancedProperties.newStringProperty(
"smtpSenderEmail", "[email protected]");
smtpSenderEmail.addListener(this);
smtpSenderName = advancedProperties.newStringProperty(
"smtpSenderName", "");
smtpSenderName.addListener(this);
smtpUsername = advancedProperties.newStringProperty("smtpUsername",
"");
smtpUsername.addListener(this);
smtpPassword = advancedProperties.newStringProperty("smtpPassword",
"");
smtpPassword.addListener(this);
schedulerThreadPoolSize = advancedProperties.newIntegerProperty(
"schedulerThreadPoolSize", 20, 1, 200);
schedulerThreadPoolSize.addListener(this);
clusterInstanceId = advancedProperties.newIntegerProperty(
"clusterInstanceId", null, 0, 131072);
clusterInstanceId.addListener(this);
if (clusterInstanceId.getValue() == null)
clusterInstanceId
.setValue((int) System.currentTimeMillis() % 131072);
} catch (XPathExpressionException e) {
throw new SearchLibException(e);
} catch (ParserConfigurationException e) {
throw new SearchLibException(e);
} catch (SAXException e) {
throw new SearchLibException(e);
} catch (IOException e) {
throw new SearchLibException(e);
} catch (URISyntaxException e) {
throw new SearchLibException(e);
}
}
protected Client newClient(File initFileOrDir,
boolean createIndexIfNotExists, boolean disableCrawler)
throws SearchLibException {
return new Client(initFileOrDir, createIndexIfNotExists, disableCrawler);
}
final public Client getNewClient(File initFileOrDir,
boolean createIndexIfNotExists, boolean disableCrawler)
throws SearchLibException {
try {
if (!FileUtils
.isSubDirectory(
StartStopListener.OPENSEARCHSERVER_DATA_FILE,
initFileOrDir))
throw new SearchLibException("Security alert: " + initFileOrDir
+ " is outside OPENSEARCHSERVER_DATA ("
+ StartStopListener.OPENSEARCHSERVER_DATA_FILE + ")");
} catch (IOException e) {
throw new SearchLibException(e);
}
return newClient(initFileOrDir, createIndexIfNotExists, disableCrawler);
}
public static void setInstance(ClientFactory cf) {
INSTANCE = cf;
}
public PropertyItem getBooleanQueryMaxClauseCount() {
return booleanQueryMaxClauseCount;
}
public PropertyItem getDefaultWebBrowserDriver() {
return defaultWebBrowserDriver;
}
public PropertyItem getSoapActive() {
return soapActive;
}
public PropertyItem getExternalParser() {
return externalParser;
}
public PropertyItem getLogFullTrace() {
return logFullTrace;
}
public PropertyItem getSmtpHostname() {
return smtpHostname;
}
public PropertyItem getSmtpPort() {
return smtpPort;
}
public PropertyItem getSmtpUseSsl() {
return smtpUseSsl;
}
public PropertyItem getSmtpUseTls() {
return smtpUseTls;
}
public PropertyItem getSmtpSenderEmail() {
return smtpSenderEmail;
}
public PropertyItem getSmtpSenderName() {
return smtpSenderName;
}
public PropertyItem getSmtpUsername() {
return smtpUsername;
}
public PropertyItem getSmtpPassword() {
return smtpPassword;
}
public PropertyItem getSchedulerThreadPoolSize() {
return schedulerThreadPoolSize;
}
public PropertyItem getClusterInstanceId() {
return clusterInstanceId;
}
@Override
public void hasBeenSet(PropertyItem> prop) throws SearchLibException {
if (prop == booleanQueryMaxClauseCount)
BooleanQuery.setMaxClauseCount(booleanQueryMaxClauseCount
.getValue());
else if (prop == logFullTrace)
Logging.setShowStackTrace(logFullTrace.isValue());
try {
advancedProperties.save();
} catch (IOException e) {
throw new SearchLibException(e);
}
}
public Sequence getGlobalSequence() {
return globalSequence;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy