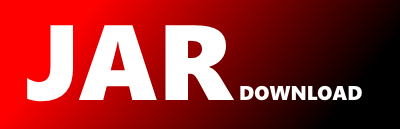
com.jakewharton.pingdom.entities.SummaryPerformance Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pingdom-java Show documentation
Show all versions of pingdom-java Show documentation
A Java wrapper around the Pingdom RESTful API and a simple DSL for easy interaction.
The newest version!
package com.jakewharton.pingdom.entities;
import java.util.Date;
import java.util.List;
import com.google.gson.annotations.Since;
import com.jakewharton.pingdom.PingdomEntity;
/**
* Represents a Pingdom summary performance object.
*/
public final class SummaryPerformance implements PingdomEntity {
private static final long serialVersionUID = 4741817235617335661L;
/**
* Represents a Pingdom summary performance hour object.
*/
public static final class Hour implements PingdomEntity {
private static final long serialVersionUID = 8901699621396348130L;
@Since(2.0) private Date startTime;
@Since(2.0) private Integer avgResponse;
@Since(2.0) private Integer uptime;
@Since(2.0) private Integer downtime;
@Since(2.0) private Integer unmonitored;
/**
* Hour interval start.
*
* @return Value.
* @since 2.0
*/
public Date getStartTime() {
return this.startTime;
}
/**
* Average response time for this interval in milliseconds.
*
* @return Value.
* @since 2.0
*/
public Integer getAverageResponse() {
return this.avgResponse;
}
/**
* Total uptime for this interval in seconds.
*
* @return Value.
* @since 2.0
*/
public Integer getUptime() {
return this.uptime;
}
/**
* Total downtime for this interval in seconds.
*
* @return Value.
* @since 2.0
*/
public Integer getDowntime() {
return this.downtime;
}
/**
* Total unmonitored time for this interval in seconds.
*
* @return Value.
* @since 2.0
*/
public Integer getUnmonitored() {
return this.unmonitored;
}
}
/**
* Represents a Pingdom summary performance day object.
*/
public static final class Day implements PingdomEntity {
private static final long serialVersionUID = 4373734505420295307L;
@Since(2.0) private Date startTime;
@Since(2.0) private Integer avgResponse;
@Since(2.0) private Integer uptime;
@Since(2.0) private Integer downtime;
@Since(2.0) private Integer unmonitored;
/**
* Day interval start.
*
* @return Value.
* @since 2.0
*/
public Date getStartTime() {
return this.startTime;
}
/**
* Average response time for this interval in milliseconds.
*
* @return Value.
* @since 2.0
*/
public Integer getAverageResponse() {
return this.avgResponse;
}
/**
* Total uptime for this interval in seconds.
*
* @return Value.
* @since 2.0
*/
public Integer getUptime() {
return this.uptime;
}
/**
* Total downtime for this interval in seconds.
*
* @return Value.
* @since 2.0
*/
public Integer getDowntime() {
return this.downtime;
}
/**
* Total unmonitored time for this interval in seconds.
*
* @return Value.
* @since 2.0
*/
public Integer getUnmonitored() {
return this.unmonitored;
}
}
/**
* Represents a Pingdom summary performance week object.
*/
public static final class Week implements PingdomEntity {
private static final long serialVersionUID = -3145455384315445757L;
@Since(2.0) private Date startTime;
@Since(2.0) private Integer avgResponse;
@Since(2.0) private Integer uptime;
@Since(2.0) private Integer downtime;
@Since(2.0) private Integer unmonitored;
/**
* Week interval start.
*
* @return Value.
* @since 2.0
*/
public Date getStartTime() {
return this.startTime;
}
/**
* Average response time for this interval in milliseconds.
*
* @return Value.
* @since 2.0
*/
public Integer getAverageResponse() {
return this.avgResponse;
}
/**
* Total uptime for this interval in seconds.
*
* @return Value.
* @since 2.0
*/
public Integer getUptime() {
return this.uptime;
}
/**
* Total downtime for this interval in seconds.
*
* @return Value.
* @since 2.0
*/
public Integer getDowntime() {
return this.downtime;
}
/**
* Total unmonitored time for this interval in seconds.
*
* @return Value.
* @since 2.0
*/
public Integer getUnmonitored() {
return this.unmonitored;
}
}
@Since(2.0) private List hours;
@Since(2.0) private List days;
@Since(2.0) private List weeks;
/**
* List of hours.
*
* @return List of values.
* @since 2.0
*/
public List getHours() {
return this.hours;
}
/**
* List of days.
*
* @return List of values.
* @since 2.0
*/
public List getDays() {
return this.days;
}
/**
* List of weeks.
*
* @return List of values.
* @since 2.0
*/
public List getWeeks() {
return this.weeks;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy