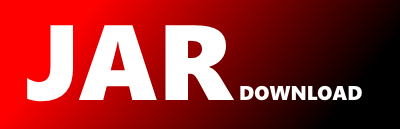
com.jk.services.server.JKServiceUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of j-framework-service Show documentation
Show all versions of j-framework-service Show documentation
A set wrappers, filters, and API's that enables faster development in microservices in Java
The newest version!
/*
* Copyright 2002-2023 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://j-framework.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.services.server;
import com.jk.core.config.JKConfig;
import com.jk.core.config.JKConstants;
import com.jk.core.context.JKContext;
import com.jk.core.context.JKContextFactory;
import com.jk.core.exceptions.JKSecurityException;
import com.jk.core.logging.JKLogger;
import com.jk.core.logging.JKLoggerFactory;
import com.jk.core.util.JK;
/**
* This class provides utility methods for working with service-related
* information.
*
* @author Dr. Jalal H. Kiswani
* @version 1.0
*/
public class JKServiceUtil {
/**
* Represents the class logger.
*/
static JKLogger logger = JKLoggerFactory.getLogger(JKServiceUtil.class);
/**
* This method retrieves the remote IP address of the client making the service
* request.
*
* @return the remote IP address as a string.
*/
public static String getRemoteIp() {
JKContext ctx = JKContextFactory.getCurrentContext();
String ip = ctx.getForwardHost();
if (ip == null) {
ip = ctx.getRealIP();
if (ip == null) {
ip = ctx.getRemoteIP();
}
}
if (ip != null) {
ip = ip.split(",")[0];// to get the first IP if there is more than one int he field, such as
// x-forward-for field
}
return ip;
}
/**
* This method retrieves the remote user associated with the service request.
*
* @return the remote user's name as a string.
*/
public static String getRemoteUser() {
String user = JKContextFactory.getCurrentContext().getUserName();
if (user == null) {
user = JKContextFactory.getCurrentContext().getRemoteUser();
}
return user;
}
/**
* This method retrieves the remote role associated with the service request.
*
* @return the remote role as a string.
*/
public static String getRemoteRole() {
String role = JKContextFactory.getCurrentContext().getRole();
if (role == null) {
role = JKContextFactory.getCurrentContext().getRemoteRole();
}
return role;
}
/**
* This method checks if the remote host making the service request is
* authorized based on its IP address.
*
* @return true, if the host is authorized, false otherwise.
*/
public static boolean isAuthorizedHost() {
JK.fixMe("Enhance for performance");
String remoteIp = getRemoteIp();
String ip = JKConfig.get().getProperty(JKConstants.Microservices.ALLOWED_IP);
if (ip == null) {
logger.debug("All IP's are allowed to call this service");
return true;
}
String[] allowedIps = ip.split(",");
for (String host : allowedIps) {
if (host.equals(remoteIp)) {
logger.debug("IP ({}) is configued to call this service", remoteIp);
return true;
}
}
logger.error("IP ({}) is not allowed to call this service", remoteIp);
return false;
}
/**
* This method checks if the remote host making the service request is
* authorized based on its IP address and throws an exception if not authorized.
*/
public static void checkAuthorizedHost() {
if (!isAuthorizedHost()) {
JK.throww(new JKSecurityException("Host not authorized ".concat(getRemoteIp())));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy