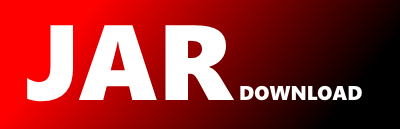
com.jk.services.server.commons.models.EndPoint Maven / Gradle / Ivy
Show all versions of j-framework-service Show documentation
/*
* Copyright 2002-2023 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://j-framework.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.services.server.commons.models;
/**
* This class represents an end-point definition.
*
* It contains information about the path, HTTP method, method name, accepted
* MIME type, returned MIME type, and parameters associated with the end-point.
*
* @author Dr. Jalal H. Kiswani
* @version 1.0
*/
public class EndPoint {
/**
* Represents the path at which the end-point is accessible.
*/
String path;
/**
* Represents the HTTP method used to access the end-point.
*/
String httpMethod;
/**
* Represents the method name associated with the end-point.
*/
String method;
/**
* Represents the accepted MIME type for incoming requests to the end-point.
*/
String acceptMimeType;
/**
* Represents the MIME type for the response returned by the end-point.
*/
String returnMimeType;
/**
* Represents the parameters associated with the end-point.
*/
String params;
/**
* This method retrieves the path at which the end-point is accessible.
*
* @return the path at which the end-point is accessible.
*/
public String getPath() {
return path;
}
/**
* This method sets the path at which the end-point is accessible to the
* provided value.
*
* @param path Specifies the new path at which the end-point is accessible.
*/
public void setPath(String path) {
this.path = path;
}
/**
* This method retrieves the method name associated with the end-point.
*
* @return the method name associated with the end-point.
*/
public String getMethod() {
return method;
}
/**
* This method sets the method name associated with the end-point to the
* provided value.
*
* @param method Specifies the new method name associated with the end-point.
*/
public void setMethod(String method) {
this.method = method;
}
/**
* This method retrieves the accepted MIME type for incoming requests to the
* end-point.
*
* @return the accepted MIME type for incoming requests to the end-point
*/
public String getAcceptMimeType() {
return acceptMimeType;
}
/**
* This method sets the accepted MIME type for incoming requests to the
* end-point to the provided value.
*
* @param acceptMimeType Specifies the new accepted MIME type for incoming
* requests to the end-point.
*/
public void setAcceptMimeType(String acceptMimeType) {
this.acceptMimeType = acceptMimeType;
}
/**
* This method retrieves the MIME type for the response returned by the
* end-point.
*
* @return the MIME type for the response returned by the end-point.
*/
public String getReturnMimeType() {
return returnMimeType;
}
/**
* This method sets the MIME type for the response returned by the end-point to
* the provided value.
*
* @param returnMimeType Specifies the new MIME type for the response returned
* by the end-point.
*/
public void setReturnMimeType(String returnMimeType) {
this.returnMimeType = returnMimeType;
}
/**
* This method retrieves the parameters associated with the end-point.
*
* @return the parameters associated with the end-point.
*/
public String getParams() {
return params;
}
/**
* This method sets the parameters associated with the end-point to the provided
* value.
*
* @param params Specifies the new parameters associated with the end-point.
*/
public void setParams(String params) {
this.params = params;
}
/**
* This method retrieves the HTTP method used to access the end-point.
*
* @return the HTTP method used to access the end-point.
*/
public String getHttpMethod() {
return httpMethod;
}
/**
* This method sets the HTTP method used to access the end-point to the provided
* value.
*
* @param httpMethod Specifies the new HTTP method used to access the end-point.
*/
public void setHttpMethod(String httpMethod) {
this.httpMethod = httpMethod;
}
}