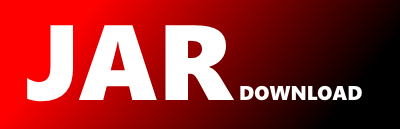
com.jk.services.server.commons.models.ServiceInfo Maven / Gradle / Ivy
/*
* Copyright 2002-2023 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://j-framework.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.services.server.commons.models;
import java.util.List;
import java.util.Vector;
/**
* This class represents information about a service, including its name, path,
* and a list of end-points.
*
* @author Dr. Jalal H. Kiswani
* @version 1.0
*/
public class ServiceInfo {
/**
* Represents the name of the service.
*/
String name;
/**
* Represents the path at which the service is accessible.
*/
String path;
/**
* Represents a list of end-points associated with the service.
*/
List endPoints;
/**
* This method retrieves the name of the service.
*
* @return the name of the service.
*/
public String getName() {
return name;
}
/**
* This method sets the name of the service to the provided value.
*
* @param name Specifies the new name of the service.
*/
public void setName(String name) {
this.name = name;
}
/**
* This method retrieves the list of end-points associated with the service.
*
* @return the list of end-points associated with the service.
*/
public List getEndPoints() {
if (endPoints == null) {
endPoints = new Vector<>();
}
return endPoints;
}
/**
* This method sets the list of end-points associated with the service to the
* provided list.
*
* @param endPoints Specifies the new list of end-points associated with the
* service.
*/
public void setEndPoints(List endPoints) {
this.endPoints = endPoints;
}
/**
* This method retrieves the path at which the service is accessible.
*
* @return the path at which the service is accessible.
*/
public String getPath() {
return path;
}
/**
* This method sets the path at which the service is accessible to the provided
* value.
*
* @param path Specifies the new path at which the service is accessible.
*/
public void setPath(String path) {
this.path = path;
}
/**
* This method adds an end-point to the list of end-points associated with the
* service.
*
* @param endpoint Represents the end-point to be added.
*/
public void addEndPoint(EndPoint endpoint) {
getEndPoints().add(endpoint);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy