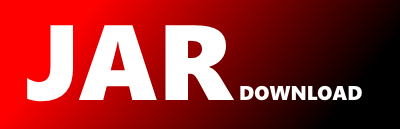
com.jk.services.server.commons.models.ServiceServerInfo Maven / Gradle / Ivy
Show all versions of j-framework-service Show documentation
/*
* Copyright 2002-2023 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://j-framework.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.services.server.commons.models;
import java.util.List;
import java.util.Vector;
/**
* This class represents information about a service server, including its name,
* start time, build time, associated services, and application name.
*
* @author Dr. Jalal H. Kiswani
* @version 1.0
*/
public class ServiceServerInfo {
/**
* Represents the name of the service server.
*/
String name;
/**
* Represents the application name associated with the service server.
*
* This should be a unique name for the whole JVM instance.
*/
private String appName;
// this shall be q unique name for the whole JVM instance, in smart-cloud, it
// should be app.name property
/**
* Represents the start time of the service server as a string.
*/
String startTime;
/**
* Represents the build time of the service server as a string.
*/
String buildTime;
/**
* Represents the list of service information objects representing the service
* hosted on the service server.
*/
List services;
/**
* This method retrieves the start time of the service server as a string.
*
* @return the start time of the service server as a string.
*/
public String getStartTime() {
return startTime;
}
/**
* This method sets the start time of the service server to the provided value.
*
* @param startTime Specifies the new start time of the service server as a
* string.
*/
public void setStartTime(String startTime) {
this.startTime = startTime;
}
/**
* This method retrieves the build time of the service server as a string.
*
* @return the build time of the service server as a string.
*/
public String getBuildTime() {
return buildTime;
}
/**
* This method sets the build time of the service server to the provided value..
*
* @param buildTime Specifies the new build time of the service server as a
* string.
*/
public void setBuildTime(String buildTime) {
this.buildTime = buildTime;
}
/**
* This method retrieves the name of the service server.
*
* @return the name of the service server.
*/
public String getName() {
return name;
}
/**
* This method sets the name of the service server to the provided value.
*
* @param name Specifies the new name of the service server.
*/
public void setName(String name) {
this.name = name;
}
/**
* This method retrieves the list of service information objects representing
* the service hosted on the service server.
*
* @return the list of service information objects representing the service
* hosted on the service server.
*/
public List getServices() {
if (services == null) {
services = new Vector();
}
return services;
}
/**
* This method sets the list of service information objects representing the
* service hosted on the service server to the provided list.
*
* @param services Specifies the new list of service information objects
* representing the service hosted on the service server.
*/
public void setServices(List services) {
this.services = services;
}
/**
* This method adds a service to the list of service information objects
* representing the service hosted on the service server.
*
* @param serverInfo Specifies the service to be added.
*/
public void addService(ServiceInfo serverInfo) {
getServices().add(serverInfo);
}
/**
* This method retrieves the application name associated with the service
* server.
*
* @return the application name associated with the service server.
*/
public String getAppName() {
return appName;
}
/**
* This method sets the application name associated with the service server to
* the provided value.
*
* @param appName Specifies the new application name associated with the service
* server.
*/
public void setAppName(String appName) {
this.appName = appName;
}
}