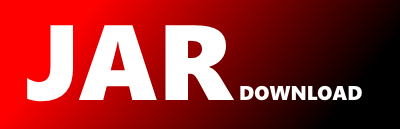
com.jk.application.generators.TableMetaXMLGenerator Maven / Gradle / Ivy
/*
* Copyright 2002-2016 Jalal Kiswani.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.application.generators;
import java.util.List;
import com.jk.logging.JKLogger;
import com.jk.logging.JKLoggerFactory;
import com.jk.metadata.db.constraints.Constraint;
import com.jk.metadata.db.constraints.DataRangeConstraint;
import com.jk.metadata.db.meta.FieldMeta;
import com.jk.metadata.db.meta.ForiegnKeyFieldMeta;
import com.jk.metadata.db.meta.IdFieldMeta;
import com.jk.metadata.db.meta.TableMeta;
/**
* The Class XMLGenerator.
*
* @author Jalal Kiswani
*/
public class TableMetaXMLGenerator {
private JKLogger logger=JKLoggerFactory.getLogger(getClass());
/**
* Instantiates a new XML generator.
*/
public TableMetaXMLGenerator() {
}
/**
*
* @param constraint
* @return
*/
protected Object buildConstraint(final Constraint constraint) {
final StringBuffer buf = new StringBuffer();
buf.append("");
for (int i = 0; i < constraint.getFields().size(); i++) {
if (constraint.getFields().get(i) != null) {
buf.append(" ");
}
}
buf.append(" ");
return buf;
}
/**
*
* @param fieldMeta
* @param buf
*/
protected void buildFieldsAttributes(final FieldMeta fieldMeta, final StringBuffer buf) {
buf.append(" name='" + fieldMeta.getName() + "' ");
if (fieldMeta.getType() != FieldMeta.FIELD_TYPE) {
buf.append(" type='" + fieldMeta.getType() + "' ");
}
if (fieldMeta.getMaxLength() != FieldMeta.MAX_LENGHT) {
buf.append(" max-length='" + fieldMeta.getMaxLength() + "' ");
}
if (fieldMeta.isRequired() != FieldMeta.REQUIRED) {
buf.append(" required='" + fieldMeta.isRequired() + "' ");
}
if (fieldMeta.isAllowUpdate() != FieldMeta.ALLOW_UPDATE) {
buf.append(" allow-update='" + fieldMeta.isAllowUpdate() + "' ");
}
if (fieldMeta.isVisible() != FieldMeta.VISIBLE) {
buf.append(" visible='" + fieldMeta.isVisible() + "' ");
}
if (fieldMeta.isEnabled() != FieldMeta.ENABLED) {
buf.append(" enabled='" + fieldMeta.isEnabled() + "'");
}
if (fieldMeta.getDefaultValue() != null && !fieldMeta.getDefaultValue().equals("")) {
buf.append(" default-value='" + fieldMeta.getDefaultValue() + "'");
}
if (fieldMeta.isSummaryField()) {
buf.append(" summary-field='" + fieldMeta.isSummaryField() + "'");
}
}
/**
* @param fieldMeta
* @return
*/
protected String buildFieldXml(final FieldMeta fieldMeta) {
if (fieldMeta instanceof ForiegnKeyFieldMeta) {
return buildForiegnKeyFieldXML((ForiegnKeyFieldMeta) fieldMeta);
}
final StringBuffer buf = new StringBuffer();
buf.append(" \n");
return buf.toString();
}
/**
*
* @param fieldMeta
* @return
*/
protected String buildForiegnKeyFieldXML(final ForiegnKeyFieldMeta fieldMeta) {
final StringBuffer buf = new StringBuffer();
buf.append(" \n");
return buf.toString();
}
/**
*
* @param tableMeta
* @return
*/
protected String buildIdFieldXML(final TableMeta tableMeta) {
final StringBuffer buf = new StringBuffer();
final IdFieldMeta id = tableMeta.getIdField();
buf.append(" \n");
return buf.toString();
}
/**
*
* @param trigger
* @return
*/
protected String buildTrigger(final String triggerName) {
final StringBuffer buf = new StringBuffer();
buf.append("");
buf.append(triggerName);
buf.append(" ");
return buf.toString();
}
/**
*
* @param tableMeta
* @return
*/
protected String generateTableMetaXML(final TableMeta tableMeta) {
logger.info("Generating tableMeta for table : " + tableMeta.getTableName());
final StringBuffer buf = new StringBuffer();
buf.append(" 0) {
buf.append(" filter-indices='" + tableMeta.getFiltersAsString() + "'");
}
if (tableMeta.getPanelClassName() != null && !tableMeta.getPanelClassName().equals("")) {
buf.append(" panel-class='" + tableMeta.getPanelClassName() + "'");
}
if (!tableMeta.isAllowAdd()) {
buf.append(" allow-add='" + tableMeta.isAllowAdd() + "' ");
}
if (!tableMeta.isAllowDelete()) {
buf.append(" allow-delete='" + tableMeta.isAllowDelete() + "' ");
}
if (!tableMeta.isAllowUpdate()) {
buf.append(" allow-update='" + tableMeta.isAllowUpdate() + "' ");
}
if (tableMeta.getDefaultUIRowCount() != TableMeta.UI_COLUMN_COUNT) {
buf.append(" ui-colunm-count='" + tableMeta.getDefaultUIRowCount() + "' ");
}
buf.append(">\n");
buf.append(buildIdFieldXML(tableMeta));
for (int i = 0; i < tableMeta.getFieldList().size(); i++) {
buf.append(buildFieldXml(tableMeta.getFieldList().get(i)));
}
if (tableMeta.getConstraints().size() > 0) {
buf.append("\n");
for (int i = 0; i < tableMeta.getConstraints().size(); i++) {
buf.append(buildConstraint(tableMeta.getConstraints().get(i)));
}
buf.append(" \n");
}
if (tableMeta.getTriggerNames().size() > 0) {
buf.append("\n");
for (final String triggerName : tableMeta.getTriggerNames()) {
buf.append(buildTrigger(triggerName) + "\n");
}
buf.append(" \n");
}
if (!tableMeta.isReportSqlNull()) {
buf.append("\n");
buf.append(tableMeta.getReportSql() + "\n");
buf.append(" \n");
}
if (!tableMeta.isListSqlNull()) {
buf.append("\n");
buf.append(tableMeta.getListSql() + "\n");
buf.append(" \n");
}
if (!tableMeta.isShortSqlNull()) {
buf.append("\n");
buf.append(tableMeta.getShortReportSql() + "\n");
buf.append(" \n");
}
buf.append("
\n");
return buf.toString();
}
/**
* Generate tables meta xml.
*
* @param tables
* the tables
* @return the string
*/
public String generateTablesMetaXml(final List tables) {
final StringBuffer buf = new StringBuffer();
buf.append("\n");
for (int i = 0; i < tables.size(); i++) {
final TableMeta tableMeta = tables.get(i);
buf.append("\n");
buf.append("\n");
buf.append("\n");
buf.append(generateTableMetaXML(tableMeta));
}
buf.append(" ");
return buf.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy