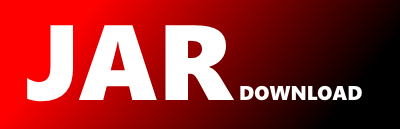
com.jk.application.api.AbstractMenuItem Maven / Gradle / Ivy
/*
* Copyright 2002-2016 Jalal Kiswani.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.application.api;
import java.util.Properties;
import com.jk.locale.JKMessage;
import com.jk.security.JKPrivilige;
import com.jk.security.JKSecurityManager;
/**
* The Class MenuItem.
*
* @author u087
*/
public abstract class AbstractMenuItem implements MenuItem {
String name;
Properties properties = new Properties();
boolean cachePanel = Boolean.valueOf(System.getProperty("fs.panel.cache", "false"));
String iconName;
int priviligeId;
// boolean cacheAtStartup =
// Boolean.valueOf(System.getProperty("fs.panel.cacheAtStartup", "true"));
// UIWidgetFactory factory =
// ApplicationFactory.getUIWidgetFactorty().creareUIViewFactory();
Menu parentMenu;
private boolean iniitialized;
private int index;
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#clear()
*/
@Override
public void clear() {
this.properties.clear();
}
// /**
// * Creates the panel.
// *
// * @return the UI view
// * @throws UIOPanelCreationException
// * the UIO panel creation exception
// */
// public UIView createPanel() throws UIOPanelCreationException {
// return createPanel(!this.cachePanel);
// }
// /**
// * Creates the panel.
// *
// * @param createNew
// * the create new
// * @return the UI view
// * @throws UIOPanelCreationException
// * the UIO panel creation exception
// */
// public UIView createPanel(final boolean createNew) throws
// UIOPanelCreationException {
// return createView(getProperties(), createNew);
// // return factory.createPanel(getProperties());
// }
/*
* (non-Javadoc)
*
* @see com.jk.metadata.application.ui.UIViewFactory#createView(java.util.
* Properties, boolean)
*/
// @Override
// public UIView createView(final Properties prop, final boolean createNew)
// throws UIOPanelCreationException {
// final UIView pnl = this.factory.createView(getProperties(), createNew);
// if (pnl != null) {
// pnl.setName(getName());
//// if (pnl instanceof DaoComponent) {
//// ((DaoComponent)
// pnl).setDataSource(getParentMenu().getParentModule().getDataSource());
//// }
// }
// return pnl;
// }
// //////////////////////////////////////////////////////////////////////////////
// public Vector getDetailTables() {
// if (!isHasDetailTables()) {
// throw new IllegalStateException("getTableMeta() is only allowed on
// table-meta");
// }
// final String[] tablesName =
// this.properties.getProperty("detail-tables").split(",");
// final Vector tables = new Vector();
// for (final String tableMetaName : tablesName) {
// tables.add(AbstractTableMetaFactory.getTableMeta(tableMetaName));
// }
// return tables;
// }
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getFullQualifiedPath()
*/
@Override
public String getFullQualifiedPath() {
final StringBuffer buf = new StringBuffer();
buf.append(getParentMenu().getFullQualifiedPath());
buf.append("-->");
buf.append(JKMessage.get(getName(), true));
return buf.toString();
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getIconName()
*/
@Override
public String getIconName() {
return this.iconName;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getName()
*/
@Override
public String getName() {
return this.name;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getParentMenu()
*/
@Override
public Menu getParentMenu() {
return this.parentMenu;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getPrivilige()
*/
//////////////////////////////////////////////////////////////////////////////
@Override
public JKPrivilige getPrivilige() {
// final int privId = (getParentMenu().getParentModule().toString() +
// getParentMenu().getName() + getName()).hashCode();
return JKSecurityManager.createPrivilige(JKMessage.get(getName(), true), getParentMenu().getPrivilige());
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getPriviligeId()
*/
@Override
public int getPriviligeId() {
return this.priviligeId;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getProperties()
*/
@Override
public Properties getProperties() {
return this.properties;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getProperty(java.lang.String)
*/
@Override
public String getProperty(final String key) {
return this.properties.getProperty(key);
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getProperty(java.lang.String, java.lang.String)
*/
@Override
public String getProperty(final String key, final String defaultValue) {
return this.properties.getProperty(key, defaultValue);
}
// //////////////////////////////////////////////////////////////////////////////
// public TableMeta getTableMeta() {
// if (!isDynamicTableMeta()) {
// throw new IllegalStateException("getTableMeta() is only allowed on
// table-meta");
// }
// return
// AbstractTableMetaFactory.getTableMeta(this.properties.getProperty("table-meta"));
// }
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#init()
*/
@Override
public void init() {
if (this.iniitialized) {
return;
}
this.iniitialized = true;
// UIPanelFactoryImpl factory = new UIPanelFactoryImpl();
// to avoid executing this executor at startup
if (this.properties.getProperty("executor") == null) {
// if (isCachePanel()) {
// try {
// factory.createPanel(properties, true);
// } catch (UIOPanelCreationException e) {
// throw new RuntimeException(e);
// }
// }
}
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#isCachePanel()
*/
@Override
public boolean isCachePanel() {
return this.cachePanel;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#isDynamicTableMeta()
*/
//////////////////////////////////////////////////////////////////////////////
@Override
public boolean isDynamicTableMeta() {
return this.properties.getProperty("table-meta") != null;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#isEmpty()
*/
@Override
public boolean isEmpty() {
return this.properties.isEmpty();
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#isExecutor()
*/
//////////////////////////////////////////////////////////////////////////////
@Override
public boolean isExecutor() {
return this.properties.getProperty("executor") != null;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#isHasDetailTables()
*/
//////////////////////////////////////////////////////////////////////////////
@Override
public boolean isHasDetailTables() {
return !this.properties.getProperty("detail-tables", "").equals("");
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#remove(java.lang.Object)
*/
@Override
public Object remove(final Object key) {
return this.properties.remove(key);
}
/**
* Sets the cache panel.
*
* @param cachePanel
* the new cache panel
* @throws ViewCreationException
* the UIO panel creation exception
*/
// public JKPanel createPanel(boolean createNew) throws
// UIOPanelCreationException {
// // if (pnl == null || createNew) {
// // this could be happend in executor classes
//
// JKPanel pnl = (JKPanel) factory.createPanel(getProperties(),createNew);
// if (pnl != null) {
// pnl.setName(getName());
// }
// // }
// return pnl;
// }
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#setCachePanel(boolean)
*/
@Override
public void setCachePanel(final boolean cachePanel) {
this.cachePanel = cachePanel;
}
// /**
// * @return the cacheAtStartup
// */
// public boolean isCacheAtStartup() {
// return cacheAtStartup;
// }
// /**
// * @param cacheAtStartup
// * the cacheAtStartup to set
// */
// public void setCacheAtStartup(boolean cacheAtStartup) {
// this.cacheAtStartup = cacheAtStartup;
// setCachePanel(cacheAtStartup);
// }
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#setIconName(java.lang.String)
*/
@Override
public void setIconName(final String iconName) {
this.iconName = iconName;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#setName(java.lang.String)
*/
@Override
public void setName(final String name) {
this.name = name;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#setParentMenu(com.jk.metadata.application.api.Menu)
*/
@Override
public void setParentMenu(final Menu parentMenu) {
this.parentMenu = parentMenu;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#setPriviligeId(int)
*/
@Override
public void setPriviligeId(final int priviligeId) {
this.priviligeId = priviligeId;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#setProperties(java.util.Properties)
*/
@Override
public void setProperties(final Properties properties) {
this.properties = properties;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#setProperty(java.lang.String, java.lang.String)
*/
@Override
public Object setProperty(final String key, final String value) {
return this.properties.setProperty(key, value);
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#size()
*/
@Override
public int size() {
return this.properties.size();
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getFullTitle()
*/
@Override
public String getFullTitle() {
return getParentMenu().getFullTitle().concat(" > ").concat(JKMessage.get(getName(), true));
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#setIndex(int)
*/
@Override
public void setIndex(int index) {
this.index = index;
}
/* (non-Javadoc)
* @see com.jk.metadata.application.MenuItem#getIndex()
*/
@Override
public int getIndex() {
return index;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy