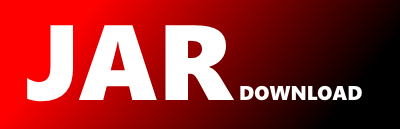
com.jk.application.api.MenuItem Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2002-2016 Jalal Kiswani.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.application.api;
import java.util.Properties;
import com.jk.security.JKPrivilige;
/**
* The Interface MenuItem.
*
* @author Jalal Kiswani
*/
public interface MenuItem {
/**
* Clear.
*
* @see java.util.Hashtable#clear()
*/
void clear();
/**
* Gets the full qualified path.
*
* @return the full qualified path
*/
String getFullQualifiedPath();
/**
* Gets the icon name.
*
* @return the iconName
*/
String getIconName();
/**
* Gets the name.
*
* @return the name
*/
String getName();
/**
* Gets the parent menu.
*
* @return the parentMenu
*/
Menu getParentMenu();
/**
* Gets the privilige.
*
* @return the privilige
*/
//////////////////////////////////////////////////////////////////////////////
JKPrivilige getPrivilige();
/**
* Gets the privilige id.
*
* @return the priviligeId
*/
int getPriviligeId();
/**
* Gets the properties.
*
* @return the properties
*/
Properties getProperties();
/**
* Gets the property.
*
* @param key
* the key
* @return the property
* @see java.util.Properties#getProperty(java.lang.String)
*/
String getProperty(String key);
/**
* Gets the property.
*
* @param key
* the key
* @param defaultValue
* the default value
* @return the property
* @see java.util.Properties#getProperty(java.lang.String, java.lang.String)
*/
String getProperty(String key, String defaultValue);
/**
* Inits the.
*/
void init();
/**
* Checks if is cache panel.
*
* @return the cachePanel
*/
boolean isCachePanel();
/**
* Checks if is dynamic table meta.
*
* @return true, if is dynamic table meta
*/
//////////////////////////////////////////////////////////////////////////////
boolean isDynamicTableMeta();
/**
* Checks if is empty.
*
* @return true, if is empty
* @see java.util.Hashtable#isEmpty()
*/
boolean isEmpty();
/**
* Checks if is executor.
*
* @return true, if is executor
*/
//////////////////////////////////////////////////////////////////////////////
boolean isExecutor();
/**
* Checks if is checks for detail tables.
*
* @return true, if is checks for detail tables
*/
//////////////////////////////////////////////////////////////////////////////
boolean isHasDetailTables();
/**
* Removes the.
*
* @param key
* the key
* @return the object
* @see java.util.Hashtable#remove(java.lang.Object)
*/
Object remove(Object key);
/**
* @param cachePanel
* the cachePanel to set
*/
void setCachePanel(boolean cachePanel);
/**
* Sets the icon name.
*
* @param iconName
* the iconName to set
*/
void setIconName(String iconName);
/**
* Sets the name.
*
* @param name
* the name to set
*/
void setName(String name);
/**
* Sets the parent menu.
*
* @param parentMenu
* the parentMenu to set
*/
void setParentMenu(Menu parentMenu);
/**
* Sets the privilige id.
*
* @param priviligeId
* the priviligeId to set
*/
void setPriviligeId(int priviligeId);
/**
* Sets the properties.
*
* @param properties
* the properties to set
*/
void setProperties(Properties properties);
/**
* Sets the property.
*
* @param key
* the key
* @param value
* the value
* @return the object
* @see java.util.Properties#setProperty(java.lang.String, java.lang.String)
*/
Object setProperty(String key, String value);
/**
* Size.
*
* @return the int
* @see java.util.Hashtable#size()
*/
int size();
/**
* Gets the full title.
*
* @return the full title
*/
String getFullTitle();
void setIndex(int index);
int getIndex();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy