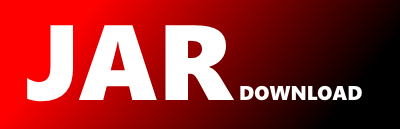
com.jk.application.parsers.ApplicationXmlParser Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2002-2016 Jalal Kiswani.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.application.parsers;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
import java.util.Vector;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import com.jk.application.api.Application;
import com.jk.application.api.ApplicationSuperFactory;
import com.jk.application.api.Module;
import com.jk.application.listener.ApplicationListener;
import com.jk.db.datasource.JKDataSourceFactory;
import com.jk.exceptions.JKXmlException;
import com.jk.util.JK;
import com.jk.util.JKConversionUtil;
/**
* The Class ApplicationXmlParser.
*
* @author Jalal Kiswani
*/
public class ApplicationXmlParser {
private static int moduleIndex = 1;
/**
* Parses the application.
*
* @param in
* the in
* @return the application
* @throws JKXmlException
* the JK xml exception
*/
public Application parseApplication(final InputStream in) throws JKXmlException {
final DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder;
try {
builder = factory.newDocumentBuilder();
final Document doc = builder.parse(in);
final Node root = doc.getFirstChild();
final NodeList list = root.getChildNodes();
final Application application = ApplicationSuperFactory.getInstance().createApplication();
final List modules = new Vector();
for (int i = 0; i < list.getLength(); i++) {
final Node node = list.item(i);
final String text = node.getTextContent().trim();
if (node.getNodeName().equals("name")) {
application.setApplicationName(text);
} else if (node.getNodeName().equals("id")) {
application.setApplicationId(Integer.parseInt(text));
} else if (node.getNodeName().equals("config-file")) {
application.setConfigFileName(text);
} else if (node.getNodeName().equals("splash-img")) {
application.setSplashImage(text);
// }else if (node.getNodeName().equals("date-format")) {
// JKDate.setDefaultDateFormat(text);
} else if (node.getNodeName().equals("view-modules")) {
application.setViewModules(Boolean.parseBoolean(text));
} else if (node.getNodeName().equals("home-img")) {
application.setHomeImage(text);
} else if (node.getNodeName().equals("background-img")) {
application.setBackgroundImage(text);
} else if (node.getNodeName().equals("locale")) {
application.setLocale(text, true);
} else if (node.getNodeName().equals("auto-logout-interval")) {
application.setSessionTimeout(JKConversionUtil.toInteger(text));
} else if (node.getNodeName().equals("module")) {
final Module module = parseModule(list.item(i));
module.setApplication(application);
modules.add(module);
} else if (node.getNodeName().equals("listener")) {
final ApplicationListener listener = parseListener(list.item(i));
application.addListener(listener);
}
}
application.setModules(modules);
return application;
} catch (final Exception e) {
throw new JKXmlException(e);
}
}
/**
*
* @param item
* @return
* @throws ClassNotFoundException
* @throws IllegalAccessException
* @throws InstantiationException
*/
private ApplicationListener parseListener(final Node item) throws Exception {
final Element e = (Element) item;
ApplicationListener listener = null;
if (!e.getAttribute("class").equals("")) {
final String className = e.getAttribute("class");
listener = JK.newInstance(className);
}
return listener;
}
/**
*
* @param item
* @return
* @throws ClassNotFoundException
* @throws IllegalAccessException
* @throws InstantiationException
* @throws IOException
*/
protected Module parseModule(final Node item) throws Exception {
final Element e = (Element) item;
Module module = null;
if (!e.getAttribute("class").equals("")) {
final String className = e.getAttribute("class");
module = (Module) Class.forName(className).newInstance();
} else if (!e.getAttribute("config-path").equals("")) {
final Module defaultModule = ApplicationSuperFactory.getInstance().createModule();
defaultModule.setConfigPath(e.getAttribute("config-path"));
module = defaultModule;
}
module.setIndex(moduleIndex++);
if (!e.getAttribute("id").equals("")) {
module.setModuleId(Integer.parseInt(e.getAttribute("id")));
}
if (!e.getAttribute("default").trim().equals("")) {
module.setDefault(Boolean.parseBoolean(e.getAttribute("default")));
}
if (!e.getAttribute("datasource-config").trim().equals("")) {
module.setDataSource(JKDataSourceFactory.createByConfigFile(e.getAttribute("datasource-config")));
}
module.setModuleName(e.getAttribute("name"));
final NodeList list = item.getChildNodes();
for (int i = 0; i < list.getLength(); i++) {
if (list.item(i).getNodeName().equals("paramters")) {
}
}
return module;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy