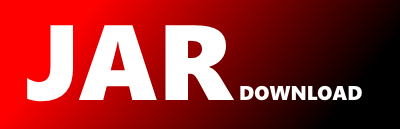
com.jk.db.dynamic.meta.AbstractEntityMetaFactory Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2002-2016 Jalal Kiswani.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.db.dynamic.meta;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Set;
import java.util.logging.Logger;
import com.jk.db.datasource.JKDataSource;
import com.jk.db.datasource.JKDataSourceFactory;
import com.jk.logging.JKLogger;
import com.jk.logging.JKLoggerFactory;
import com.jk.metadata.db.exceptions.TableMetaNotFoundException;
import com.jk.metadata.db.meta.EntityMeta;
/**
* A factory for creating AbstractTableMeta objects.
*/
public class AbstractEntityMetaFactory {
protected static AbstractEntityMetaFactory instance;
JKLogger logger = JKLoggerFactory.getLogger(AbstractEntityMetaFactory.class);
HashMap metaFactorys = new HashMap();
JKDataSource defaultDataSource = JKDataSourceFactory.getDefaultDataSource();
public static AbstractEntityMetaFactory getInstance() {
if (instance == null) {
instance = new AbstractEntityMetaFactory();
}
return instance;
}
public static void setInstance(AbstractEntityMetaFactory instance) {
AbstractEntityMetaFactory.instance = instance;
}
protected AbstractEntityMetaFactory() {
final EntityMetaFactory defaultFactory = new EntityMetaFactory(defaultDataSource);
metaFactorys.put(defaultDataSource, defaultFactory);
}
/**
* Adds the tables meta.
*
* @param connectionManager the connection manager
* @param newEntities the new tables
* @return the table meta factory
*/
// //////////////////////////////////////////////////////////////////////////////////////////////////////
public EntityMetaFactory addEntitiesMeta(final JKDataSource connectionManager,
final Hashtable newEntities) {
logger.debug("Add new entities...");
final EntityMetaFactory metaFactory = getEntityMetaFactory(connectionManager);
metaFactory.addEntitiesMeta(newEntities);
return metaFactory;
}
/**
* Gets the default meta factory.
*
* @return the default meta factory
*/
public EntityMetaFactory getDefaultEntityMetaFactory() {
return metaFactorys.get(defaultDataSource);
}
// ////////////////////////////////////////////////////////////////////////////////////////////////////////
// public static void loadMetaFiles(InputStream in) throws
// FileNotFoundException, JKXmlException {
// getDefaultMetaFactory().loadMetaFiles(in);
// }
/**
* Gets the meta factory.
*
* @param connectionManager the connection manager
* @return the meta factory
*/
// //////////////////////////////////////////////////////////////////////////////////////////////////////
public EntityMetaFactory getEntityMetaFactory(final JKDataSource connectionManager) {
EntityMetaFactory metaFactory = metaFactorys.get(connectionManager);
if (metaFactory == null) {
metaFactory = new EntityMetaFactory(connectionManager);
metaFactorys.put(connectionManager, metaFactory);
}
return metaFactory;
}
/**
* Gets the table meta.
*
* @param connectionManager the connection manager
* @param metaName the meta name
* @return the table meta
*/
// ////////////////////////////////////////////////////////////////////////////////////////////////
public EntityMeta getEntityMeta(final JKDataSource connectionManager, final String metaName) {
logger.debug("getTableMeta :" + metaName);
return metaFactorys.get(connectionManager).getEntityeMeta(metaName);
}
// /**
// * @return
// */
// public static Hashtable getTables() {
// return getDefaultMetaFactory().getTables();
// }
// /**
// *
// * @return
// */
// public static ArrayList getTablesAsArrayList() {
// return getDefaultMetaFactory().getTablesAsArrayList();
// }
// public static boolean isMetaExists(String metaName) {
// return getDefaultMetaFactory().isMetaExists(metaName);
// }
/**
* If if connectinManager is not defined , the system will start look inside
* other meta.
*
* @param tableName the table name
* @return the table meta
* @throws TableMetaNotFoundException the table meta not found exception
*/
public EntityMeta getEntityMeta(final String tableName) {
logger.debug("getTableMeta : " + tableName);
final EntityMetaFactory defaultMetaFactory = getDefaultEntityMetaFactory();
if (defaultMetaFactory.isEntityMetaExists(tableName)) {
logger.debug("get from default datasource");
return defaultMetaFactory.getEntityeMeta(tableName);
}
// look in other connections
logger.debug("not found inside default datasource , look into other connections");
final Set keys = metaFactorys.keySet();
for (JKDataSource key : keys) {
final EntityMetaFactory tableMetaFactory = metaFactorys.get(key);
if (defaultMetaFactory != tableMetaFactory) {
if (tableMetaFactory.isEntityMetaExists(tableName)) {
return tableMetaFactory.getEntityeMeta(tableName);
}
}
}
throw new TableMetaNotFoundException("TableMeta : " + tableName + " doesnot exists");
}
/**
* Register factory.
*
* @param manager the manager
* @param factory the factory
*/
public void registerFactory(final JKDataSource manager, final EntityMetaFactory factory) {
metaFactorys.put(manager, factory);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy