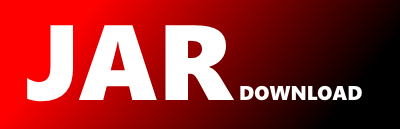
com.jk.db.dynamic.oracle.OracleDynamicDao Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2002-2016 Jalal Kiswani.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.db.dynamic.oracle;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Date;
import com.jk.db.dataaccess.oracle.OracleAbstractDao;
import com.jk.db.dataaccess.plain.JKUpdater;
import com.jk.db.dynamic.dataaccess.DynamicDataAccess;
import com.jk.db.util.JdbcUtil;
import com.jk.metadata.db.meta.EntityRecord;
import com.jk.metadata.db.meta.EntityMeta;
/**
* The Class OracleDynamicDao.
*
* @author Jalal Kiswani
*/
public class OracleDynamicDao extends DynamicDataAccess {
private final OracleAbstractDao oracleDao = new OracleAbstractDao();
/**
* Instantiates a new oracle dynamic dao.
*
* @param tableMetaName
* the table meta name
*/
// /////////////////////////////////////////////////////////////////
public OracleDynamicDao(final String tableMetaName) {
super(tableMetaName);
}
/**
* Instantiates a new oracle dynamic dao.
*
* @param meta
* the meta
*/
public OracleDynamicDao(EntityMeta meta) {
super(meta);
}
// ////////////////////////////////////////////////////////////////////////////
@Override
protected int getGeneratedKeys(final PreparedStatement ps) throws SQLException {
// this method should be fixed to support the last insert id in smarter
// way , since its not supported by oracle
// return 0;
throw new IllegalStateException("getGeneratedKeys not implemented on oracle DBMS");
}
/*
* (non-Javadoc)
*
* @see com.jk.db.dataaccess.plain.JKAbstractPlainDataAccess#getSystemDate()
*/
@Override
public Date getSystemDate() {
return this.oracleDao.getSystemDate();
}
/*
* (non-Javadoc)
*
* @see
* com.jk.db.dynamic.DynamicDao#insertRecord(com.jk.metadata.db.meta.Record)
*/
// //////////////////////////////////////////////////////////////
@Override
public String insertRecord(final EntityRecord record) {
if (record.getIdValue() == null) {
final long nextId = this.oracleDao.getNextId(this.tableMeta.getTableName(), record.getIdField().getFieldName());
record.setIdValue(nextId);
}
callBeforeAddEventOnTriggers(record);
final JKUpdater updater = new JKUpdater() {
@Override
public String getQuery() {
return OracleDynamicDao.this.sqlBuilder.buildInsert(record);
}
@Override
public void setParamters(final PreparedStatement ps) throws SQLException {
OracleDynamicDao.this.setParamters(record, ps, true);
}
};
executeUpdate(updater);
callAfterAddEventOnTriggers(record);
// addInsertAudit(record);
return record.getIdValue().toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy