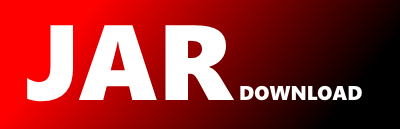
com.jk.data.dataaccess.orm.JKEntity Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.data.dataaccess.orm;
import java.io.Serializable;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.util.List;
import java.util.Vector;
import com.jk.core.util.JKObjectUtil;
import com.jk.data.dataaccess.orm.meta.JKColumnWrapper;
import com.jk.data.dataaccess.orm.meta.JKSortDirection;
import com.jk.data.dataaccess.orm.meta.JKSortInfo;
import jakarta.persistence.Column;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import jakarta.persistence.Table;
// TODO: Auto-generated Javadoc
/**
* The Class JKEntity.
*/
public class JKEntity implements Serializable {
/** The id. */
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
/** The table name. */
String tableName;
/**
* Instantiates a new JK entity.
*/
public JKEntity() {
}
/**
* Gets the table name.
*
* @return the table name
*/
public String getTableName() {
Table annotation = getClass().getAnnotation(Table.class);
return annotation.name();
}
/**
* Gets the id value.
*
* @param the generic type
* @return the id value
*/
public T getIdValue() {
return JKObjectUtil.getPropertyValue(this, this.getIdColumn().getFieldName());
}
/**
* Gets the first non id column.
*
* @return the first non id column
*/
// ///////////////////////////////////////////////
public Column getFirstNonIdColumn() {
Field[] declaredFields = getClass().getDeclaredFields();
for (Field field : declaredFields) {
if (field.getAnnotation(Id.class) == null) {
Column column = field.getAnnotation(Column.class);
if (column != null) {
return new JKColumnWrapper(field, column);
}
}
}
return null;
}
/**
* Gets the columns.
*
* @param clas the clas
* @return the columns
*/
// /////////////////////////////////////////////////////////////////
public static List getColumns(Class extends JKEntity> clas) {
Field[] declaredFields = clas.getDeclaredFields();
Vector columns = new Vector<>();
for (Field field : declaredFields) {
Column column = field.getAnnotation(Column.class);
Id id = field.getAnnotation(Id.class);
JKSortInfo sortInfo = field.getAnnotation(JKSortInfo.class);
if (column != null) {
JKColumnWrapper wrapper = new JKColumnWrapper(field, column);
wrapper.setId(id);
wrapper.setSortInfo(addColumnToSortInfo(wrapper, sortInfo));
columns.add(wrapper);
}
}
return columns;
}
/**
* Adds the column to sort info.
*
* @param wrapper the wrapper
* @param sortInfo the sort info
* @return the JK sort info
*/
// ///////////////////////////////////////////////////
private static JKSortInfo addColumnToSortInfo(final JKColumnWrapper wrapper, final JKSortInfo sortInfo) {
if (sortInfo == null) {
return null;
}
JKSortInfo info = new JKSortInfo() {
@Override
public Class extends Annotation> annotationType() {
return sortInfo.annotationType();
}
@Override
public JKSortDirection sortOrder() {
return sortInfo.sortOrder();
}
@Override
public Column column() {
return wrapper;
}
};
return info;
}
/**
* Gets the id column.
*
* @return the id column
*/
// ///////////////////////////////////////////////////
public JKColumnWrapper getIdColumn() {
return getIdColumn(this.getClass());
}
/**
* Gets the id column.
*
* @param clas the clas
* @return the id column
*/
// ///////////////////////////////////////////////////
public static JKColumnWrapper getIdColumn(Class extends JKEntity> clas) {
for (JKColumnWrapper col : getColumns(clas)) {
if (col.isId()) {
return col;
}
}
return null;
}
/**
* Prints the fields annotations.
*/
// ///////////////////////////////////////////////
public void printFieldsAnnotations() {
Field[] declaredFields = getClass().getDeclaredFields();
for (Field field : declaredFields) {
printFieldAnnotations(field);
}
}
/**
* Prints the field annotations.
*
* @param field the field
*/
// ///////////////////////////////////////////////
public static void printFieldAnnotations(Field field) {
Annotation[] annotations = field.getAnnotations();
for (Annotation annotation : annotations) {
System.out.println(annotation);
}
}
/**
* To string.
*
* @return the string
*/
/*
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
List columns = getColumns(getClass());
StringBuffer buf = new StringBuffer();
buf.append(getAliasName());
buf.append("[");
int i = 0;
for (JKColumnWrapper column : columns) {
if (!column.getFieldName().toLowerCase().contains("password")) {
if (i++ > 0) {
buf.append(",");
}
buf.append(column.name());
buf.append("=");
buf.append(JKObjectUtil.getPropertyValue(this, column.getFieldName()).toString());
}
}
buf.append("]\n");
return buf.toString();
}
/**
* Gets the sort info.
*
* @param clas the clas
* @return the sort info
*/
// ///////////////////////////////////////////////
public static JKSortInfo getSortInfo(Class extends JKEntity> clas) {
for (JKColumnWrapper column : getColumns(clas)) {
if (column.getSortInfo() != null) {
return column.getSortInfo();
}
}
return null;
}
/**
* Equals.
*
* @param obj the obj
* @return true, if successful
*/
/*
* (non-Javadoc)
*
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (!(obj instanceof JKEntity)) {
return false;
}
if (obj == this) {
return true;
}
// TODO : fix this to support equality before persist the entity to db
// since
JKEntity that = (JKEntity) obj;
if (this.getTableName().equals(that.getTableName())) {
if (this.getIdValue() != null && this.getIdValue().equals(that.getIdValue())) {
return true;
}
}
return false;
}
/**
* Hash code.
*
* @return the int
*/
/*
* (non-Javadoc)
*
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode() {
int hashcode = getIdValue().hashCode();
return hashcode;
}
/**
* Gets the alias name.
*
* @return the alias name
*/
public String getAliasName() {
return getClass().getSimpleName();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy