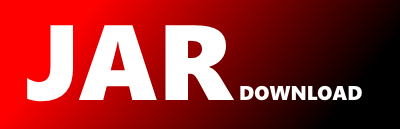
com.jk.data.datasource.DefaultPhysicalNamingStrategy Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.data.datasource;
import java.io.Serializable;
import java.util.Collections;
import java.util.List;
import org.hibernate.boot.model.naming.Identifier;
import org.hibernate.boot.model.naming.PhysicalNamingStrategy;
import org.hibernate.engine.jdbc.env.spi.JdbcEnvironment;
// TODO: Auto-generated Javadoc
/**
* The Class DefaultPhysicalNamingStrategy.
*/
public class DefaultPhysicalNamingStrategy implements PhysicalNamingStrategy, Serializable {
/**
* The Enum NamePattern.
*/
public enum NamePattern {
/** The lower case. */
LOWER_CASE,
/** The upper case. */
UPPER_CASE,
/** The as is with underscores. */
AS_IS_WITH_UNDERSCORES,
/** The as is. */
AS_IS;
}
/**
* To physical catalog name.
*
* @param name the name
* @param jdbcEnvironment the jdbc environment
* @return the identifier
*/
@Override
public Identifier toPhysicalCatalogName(Identifier name, JdbcEnvironment jdbcEnvironment) {
return convertToFinalFormat(name);
}
/**
* To physical schema name.
*
* @param name the name
* @param jdbcEnvironment the jdbc environment
* @return the identifier
*/
@Override
public Identifier toPhysicalSchemaName(Identifier name, JdbcEnvironment jdbcEnvironment) {
return convertToFinalFormat(name);
}
/**
* To physical table name.
*
* @param name the name
* @param jdbcEnvironment the jdbc environment
* @return the identifier
*/
@Override
public Identifier toPhysicalTableName(Identifier name, JdbcEnvironment jdbcEnvironment) {
return convertToFinalFormat(name);
}
/**
* To physical sequence name.
*
* @param name the name
* @param jdbcEnvironment the jdbc environment
* @return the identifier
*/
@Override
public Identifier toPhysicalSequenceName(Identifier name, JdbcEnvironment jdbcEnvironment) {
return convertToFinalFormat(name);
}
/**
* To physical column name.
*
* @param name the name
* @param jdbcEnvironment the jdbc environment
* @return the identifier
*/
@Override
public Identifier toPhysicalColumnName(Identifier name, JdbcEnvironment jdbcEnvironment) {
return convertToFinalFormat(name);
}
/**
* Convert to snake case.
*
* @param identifier the identifier
* @return the identifier
*/
private Identifier convertToFinalFormat(final Identifier identifier) {
if (identifier == null) {
return null;
}
if (getPattern() == NamePattern.AS_IS) {
return identifier;
}
String regex = "([a-z])([A-Z])";
String replacement = "$1_$2";
String newName = identifier.getText().replaceAll(regex, replacement);
if (getKeywords().contains(newName.toUpperCase())) {
newName = newName + "_";
}
switch (getPattern()) {
case LOWER_CASE:
newName = newName.toLowerCase();
break;
case UPPER_CASE:
newName = newName.toUpperCase();
}
return Identifier.toIdentifier(newName, identifier.isQuoted());
}
/**
* Gets the pattern.
*
* @return the pattern
*/
public NamePattern getPattern() {
return NamePattern.LOWER_CASE;
}
/**
* Gets the keywords.
*
* @return the keywords
*/
public List getKeywords() {
return Collections.EMPTY_LIST;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy