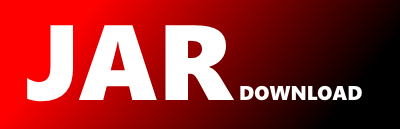
com.jk.data.datasource.JKDataSourceFactory Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.data.datasource;
import java.sql.Driver;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.Collection;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import com.jk.core.config.JKConfig;
import com.jk.core.config.JKConfigEvent;
import com.jk.core.config.JKConfigListener;
import com.jk.core.config.JKConstants;
import com.jk.core.logging.JKLogger;
import com.jk.core.logging.JKLoggerFactory;
import com.jk.core.util.JK;
import com.jk.data.datasource.impl.JKHibernateDataSource;
import com.jk.data.datasource.impl.JKPlainDataSource;
// TODO: Auto-generated Javadoc
/**
* A factory for creating JKDataSource objects.
*/
public class JKDataSourceFactory {
/** The Constant DEFAULT_PREFIX. */
private static final String DEFAULT_PREFIX = "default";
/** The logger. */
static JKLogger logger = JKLoggerFactory.getLogger(JKDataSourceFactory.class);
/** The datasources. */
static Map datasources = new HashMap<>();
static {
JKConfig.addListner(new JKConfigListener() {
@Override
public void reloadConfig(JKConfigEvent event) {
close();
}
@Override
public void closeConfig(JKConfigEvent event) {
close();
}
});
}
/**
* Creates a new JKDataSource object.
*
* @return the JK data source
*/
public static JKDataSource getDefaultDataSource() {
return getDataSource(DEFAULT_PREFIX);
}
/**
* Creates a new JKDataSource object.
*
* @param prefix the prefix
* @return the JK data source
*/
public static synchronized JKDataSource getDataSource(String prefix) {
logger.debug("getDataSource for prefix({})", prefix);
JKDataSource datasource = datasources.get(prefix);
if (datasource == null) {
logger.info("datasource ({}) not found, create new one", prefix);
Properties properties = JKConfig.get().toProperties();
if (!prefix.equals(DEFAULT_PREFIX)) {
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy