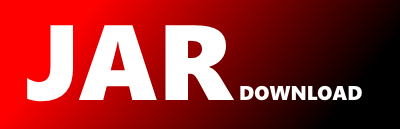
com.jk.data.dynamic.analyzer.DataBaseAnaylazer Maven / Gradle / Ivy
package com.jk.data.dynamic.analyzer;
///*
// * Copyright 2002-2018 Jalal Kiswani.
// * E-mail: [email protected]
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//package com.jk.db.test.dynamic.analyzer;
//
//import java.sql.Connection;
//import java.sql.DatabaseMetaData;
//import java.sql.ResultSet;
//import java.sql.SQLException;
//import java.util.ArrayList;
//import java.util.List;
//import java.util.Vector;
//
//import javax.annotation.PostConstruct;
//import javax.sql.rowset.CachedRowSet;
//
//import com.jk.db.test.dataaccess.core.JKDataAccessService;
//import com.jk.db.test.datasource.JKDataSource;
//import com.jk.db.test.datasource.JKDataSourceFactory;
//import com.jk.db.test.multitent.MultiTenantSupport;
//import com.jk.metadata.core.EntityMetadata;
//import com.jk.metadata.core.FieldMetadata;
//import com.jk.metadata.core.ForiegnKeyFieldMetaData;
//import com.jk.metadata.core.IdFieldMetadata;
//import com.jk.metadata.importers.DataBaseImporter;
//import com.jk.util.exceptions.JKDataAccessException;
//import com.jk.util.exceptions.handler.JKExceptionUtil;
//import com.jk.util.logging.JKLogger;
//import com.jk.util.logging.JKLoggerFactory;
//
//// TODO: Auto-generated Javadoc
///**
// * The Class AbstractDataBaseAnaylazer.
// *
// * @author Jalal Kiswani
// */
//public class DataBaseAnaylazer implements DataBaseImporter {
//
// /** The logger. */
// JKLogger logger = JKLoggerFactory.getLogger(getClass());
//
// /**
// * The main method.
// *
// * @param args the arguments
// * @throws JKDataAccessException the JK data access exception
// * @throws SQLException the SQL exception
// */
// public static void main(final String[] args) throws JKDataAccessException, SQLException {
// // final AbstractDataBaseAnaylazer a = (AbstractDataBaseAnaylazer)
// // JKDataSourceFactory.getDataSource().getDatabaseAnasyaler();
// }
//
// /** The meta. */
// private DatabaseMetaData meta;
//
// /** The data source. */
// private JKDataSource dataSource;
//
// /** The dao. */
// private JKDataAccessService dao;
//
// /**
// * Instantiates a new abstract data base anaylazer.
// */
// public DataBaseAnaylazer() {
// }
//
// @PostConstruct
// public void init() {
// this.dataSource=JKDataSourceFactory.getDefaultDataSource();
// final Connection connection = dataSource.getQueryConnection();
// try {
// this.meta = connection.getMetaData();
// this.dao = JKDataSourceFactory.getDataAccessService();
// this.dao.setEnableMultiTenant(false);
// } catch (Exception e) {
// JKExceptionUtil.handle(e);
// } finally {
// dataSource.close(connection);
// }
// }
//
// /**
// * Instantiates a new abstract data base anaylazer.
// *
// * @param dataSource the data source
// */
// public DataBaseAnaylazer(final JKDataSource dataSource) {
// this.dataSource = dataSource;
// init();
// }
//
// /**
// * Gets the catalogs typeName.
// *
// * @return the catalogs typeName
// */
// /*
// * (non-Javadoc)
// *
// * @see com.jk.db.test.dynamic.meta.generator.DataBaseAnalayzer#getCatalogsName()
// */
// public List getCatalogsName() {
// try {
// final ResultSet rs = this.meta.getCatalogs();
// final ArrayList catalogNames = new ArrayList();
// final int counter = 1;
// while (rs.next()) {
// // for (int i = 0; i < rs.getMetaData().getColumnCount(); i++) {
// catalogNames.add(rs.getString("TABLE_CAT"));
// // }
// }
// rs.close();
// return catalogNames;
// } catch (Exception e) {
// JKExceptionUtil.handle(e);
// return null;
// }
// }
//
// /*
// * (non-Javadoc)
// *
// * @see com.jk.db.test.dynamic.meta.generator.DataBaseAnalayzer#getDatabasesName()
// */
// /**
// * Gets the databases typeName.
// *
// * @return the databases typeName
// */
// // /////////////////////////////////////////////////////////////////////////////////////
// public List getDatabasesName() {
// return getCatalogsName();
// }
//
// /**
// * Gets the foriegn keys.
// *
// * @param databaseName the database typeName
// * @param tableName the table typeName
// * @return @
// * @throws SQLException the SQL exception
// */
// private List getForiegnKeys(final String databaseName, final String tableName) throws SQLException {
// final ResultSet rs = getImportedKeys(this.meta, databaseName, tableName);
// final List fields = new ArrayList();
// while (rs.next()) {
// final ForiegnKeyFieldMetaData field = new ForiegnKeyFieldMetaData();
// field.setName(rs.getString("FKCOLUMN_NAME"));
// field.setReferenceEntityName(rs.getString("PKTABLE_NAME"));
// field.setReferenceFieldName(rs.getString("PKCOLUMN_NAME"));
// fields.add(field);
// }
// rs.close();
// return fields;
// }
//
// /**
// * Gets the id field.
// *
// * @param databaseName the database typeName
// * @param tableName the table typeName
// * @return the id field
// * @throws JKDataAccessException the JK data access exception
// * @throws SQLException the SQL exception
// * @ @throws JKDataAccessException
// */
// private IdFieldMetadata getIdField(final String databaseName, final String tableName) throws JKDataAccessException, SQLException {
// final ResultSet rs = getPrimaryKeysFromMeta(this.meta, databaseName, tableName);
// try {
// if (rs.next()) {
// final IdFieldMetadata id = new IdFieldMetadata();
// id.setName(rs.getString("COLUMN_NAME"));
// final boolean autoIncrement = isAutoIncrement(databaseName, tableName);
// id.setAutoIncrement(autoIncrement);
// return id;
// }
// return null;
// } finally {
// rs.close();
// }
//
// }
//
// // ///////////////////////////////////////////////////////////////////////////////////////////////////////////
// /*
// * (non-Javadoc)
// *
// * @see com.fs.commons.dao.dynamic.meta.generator.DataBaseAnaylser1#
// * getDyanmicMeta (java.lang.String)
// */
// // @Override
// // public Hashtable getDyanmicMeta(String databaseName)
// // , DaoException {
// // ArrayList tables = getTablesMeta(databaseName);
// // Hashtable hash = new Hashtable();
// // for (TableMeta tableMeta : tables) {
// // hash.put(tableMeta.getTableName(), tableMeta);
// // }
// // return hash;
// // }
//
// /**
// * Gets the imported keys.
// *
// * @param meta the meta
// * @param databaseName the database typeName
// * @param tableName the table typeName
// * @return the imported keys
// * @throws SQLException the SQL exception
// */
// // //////////////////////////////////////////////////////////////////////////
// protected ResultSet getImportedKeys(final DatabaseMetaData meta, final String databaseName, final String tableName) throws SQLException {
// return meta.getImportedKeys(databaseName, null, tableName);
// }
//
// /**
// * Gets the primary keys from meta.
// *
// * @param meta the meta
// * @param databaseName the database typeName
// * @param tableName the table typeName
// * @return the primary keys from meta
// * @throws SQLException the SQL exception
// */
// // //////////////////////////////////////////////////////////////////////////////////////////
// protected ResultSet getPrimaryKeysFromMeta(final DatabaseMetaData meta, final String databaseName, final String tableName) throws SQLException {
// return meta.getPrimaryKeys(databaseName, null, tableName);
// }
//
// /*
// * (non-Javadoc) TODO : check me
// *
// * @see com.jk.db.test.dynamic.meta.generator.DataBaseAnalayzer#getSchemas()
// */
// /**
// * Gets the schemas.
// *
// * @return the schemas
// */
// // /////////////////////////////////////////////////////////////////////////////////////
// public List getSchemas() {
// try {
// final ResultSet rs = this.meta.getSchemas();
// final ArrayList schemaNames = new ArrayList();
// while (rs.next()) {
// // for (int i = 0; i < rs.getMetaData().getColumnCount(); i++) {
// schemaNames.add(rs.getString("TABLE_SCHEM"));
// // }
// }
// rs.close();
// return schemaNames;
// } catch (Exception e) {
// JKExceptionUtil.handle(e);
// return null;
// }
// }
//
// /**
// * Gets the table.
// *
// * @param databaseName the database typeName
// * @param tableName the table typeName
// * @return the table
// */
// // //////////////////////////////////////////////////////////////////////////////
// protected EntityMetadata getTable(final String databaseName, final String tableName) {
// logger.debug("getTable ({}) from database ({})", tableName, databaseName);
// if (isEntityExist(tableName)) {
// logger.debug("table ({}) was found", tableName);
// // System.out.println("Fetching table : " + tableName);
// final EntityMetadata meta = new EntityMetadata();
// meta.setName(tableName);
// // meta.setIdField(getIdField(databaseName, tableName));
// loadFields(databaseName, meta);
// return meta;
// }
// logger.debug("Table ({}) wasnt found in database ({}), returing null", tableName, databaseName);
// return null;
// }
//
// /**
// * Gets the table columns from meta.
// *
// * @param meta the meta
// * @param database the database
// * @param tableName the table typeName
// * @return the table columns from meta
// * @throws SQLException the SQL exception
// */
// protected ResultSet getTableColumnsFromMeta(final DatabaseMetaData meta, final String database, final String tableName) throws SQLException {
// return meta.getColumns(database, null, tableName, null);
// }
//
// /**
// * Gets the entities metadata.
// *
// * @return the entities metadata
// */
// /*
// * (non-Javadoc)
// *
// * @see com.jk.db.test.dynamic.meta.generator.DataBaseAnalayzer#getTablesMeta()
// */
// public List getEntitiesMetadata() {
// logger.debug("getEntitiesMetadata()");
// return getEntitiesMetadata(this.dataSource.getDatabaseName());
// }
//
// /**
// * Gets the entities metadata.
// *
// * @param databaseName the database typeName
// * @return the entities metadata
// */
// /*
// * (non-Javadoc)fv
// *
// * @see com.jk.db.test.dynamic.meta.generator.DataBaseAnalayzer#getTablesMeta(java.
// * lang.String)
// */
// public List getEntitiesMetadata(final String databaseName) {
// logger.debug("getEntitiesMetadata for database ({})", databaseName);
// try {
// final ResultSet rs = loadTableNamesFromMeta(this.meta, databaseName);
// final List tables = new Vector();
//
// while (rs.next()) {
// final String tableType = rs.getString("TABLE_TYPE");
// if (tableType.toUpperCase().equals("TABLE")) {
// final String tableName = rs.getString("TABLE_NAME");
// final EntityMetadata meta = getTable(databaseName, tableName);
// tables.add(meta);
// }
// }
// rs.close();
// return tables;
// } catch (Exception e) {
// JKExceptionUtil.handle(e);
// return null;
// }
// }
//
// /**
// * Checks if is auto increment.
// *
// * @param databaseName the database typeName
// * @param tableName the table typeName
// * @return true, if is auto increment
// * @throws JKDataAccessException the JK data access exception
// * @throws SQLException the SQL exception
// */
// // //////////////////////////////////////////////////////////////////////////////////////
// protected boolean isAutoIncrement(final String databaseName, final String tableName) throws JKDataAccessException, SQLException {
// final String emptyRowQuery = buildEmptyRowQuery(databaseName, tableName);
// logger.trace("Executing : (" + emptyRowQuery + ") to check isAutoIncrement...");
// final CachedRowSet rowSet = this.dao.executeQueryAsCachedRowSet(emptyRowQuery);
// final boolean autoIncrement = rowSet.getMetaData().isAutoIncrement(1);
// return autoIncrement;
// }
//
// /*
// * (non-Javadoc)
// *
// * @see
// * com.jk.db.test.dynamic.meta.generator.DataBaseAnalayzer#isTableExist(java.lang
// * .String)
// */
// /**
// * Checks if is entity exist.
// *
// * @param tableName the table typeName
// * @return true, if is entity exist
// */
// // /////////////////////////////////////////////////////////////////////////////////////
// public boolean isEntityExist(final String tableName) {
// return dao.isTableExists(tableName);
//// final List tables = getEntitiesMetadata(this.dataSource.getDatabaseName());
//// for (final EntityMetadata tableMeta : tables) {
//// if (tableMeta.getName().trim().equalsIgnoreCase(tableName)) {
//// return true;
//// }
//// }
//// return false;
// }
//
// // ///////////////////////////////////////////////////////////////////////////////////////////////////////////
// /*
// * (non-Javadoc)
// *
// * @see com.fs.commons.dao.dynamic.meta.generator.DataBaseAnaylser1#getFields
// * (java.lang.String, java.lang.String)
// */
// /**
// * Load fields.
// *
// * @param database the database
// * @param tableMeta the table meta
// */
// // @Override
// protected void loadFields(final String database, final EntityMetadata tableMeta) {
// logger.debug("Import fields for entity ({})", tableMeta.getName());
// try {
// IdFieldMetadata idField = getIdField(database, tableMeta.getName());
// tableMeta.setIdField(idField);
// final List foriegnFields = getForiegnKeys(database, tableMeta.getName());
// for (final ForiegnKeyFieldMetaData foriegnKeyFieldMetaData : foriegnFields) {
// foriegnKeyFieldMetaData.setParentEntity(tableMeta);
// }
//
// final ResultSet rs = getTableColumnsFromMeta(this.meta, database, tableMeta.getName());
// while (rs.next()) {
// final String fieldName = rs.getString("COLUMN_NAME");
// if (isFieldNameAllowed(fieldName)) {
// // System.out.println("Processing field : "+fieldName);
// FieldMetadata fieldMetadata;
// boolean newField = true;
// // the following check is used for primary keys and foreign keys
// // which is already added to the list
// if ((fieldMetadata = tableMeta.getField(fieldName, true)) != null) {
// newField = false;
// } else {
// if (idField == null) {
// idField = new IdFieldMetadata();
// idField.setAutoIncrement(true);
// tableMeta.setIdField(idField);
// fieldMetadata = idField;
// newField = false;
// } else {
// fieldMetadata = new FieldMetadata();
// }
// fieldMetadata.setName(fieldName);
// fieldMetadata.setParentEntity(tableMeta);
// int index;
// if ((index = foriegnFields.indexOf(fieldMetadata)) != -1) {
// fieldMetadata = foriegnFields.get(index);
// }
// }
// final int type = rs.getInt("DATA_TYPE");
// final int nullable = rs.getInt("NULLABLE");
// final String defaultValue = rs.getString("COLUMN_DEF");
// final int maxLength = rs.getInt("COLUMN_SIZE");
// // int decimalDigits=rs.getInt("DECIMAL_DIGITS");
//
// fieldMetadata.setMaxLength(maxLength);
// fieldMetadata.setType(type);
// fieldMetadata.setRequired(nullable == 0 ? true : false);
// if (defaultValue != null && !defaultValue.equals("null")) {
// fieldMetadata.setDefaultValue(defaultValue);
// }
// if (newField) {
// tableMeta.addField(fieldMetadata);
// logger.debug("\t({})", fieldMetadata);
// }
// }
// }
// rs.close();
// } catch (Exception e) {
// JKExceptionUtil.handle(e);
// }
// }
//
// /**
// *
// * @param fieldName
// * @return
// */
// public boolean isFieldNameAllowed(String fieldName) {
// return !fieldName.equals(MultiTenantSupport.TENANT_FIELD_NAME) ;
// }
//
// /**
// * Load table names from meta.
// *
// * @param meta the meta
// * @param dbName the db typeName
// * @return the result set
// * @throws SQLException the SQL exception
// */
// // /////////////////////////////////////////////////////////////////////////////////////
// protected ResultSet loadTableNamesFromMeta(final DatabaseMetaData meta, final String dbName) throws SQLException {
// return meta.getTables(dbName, null, null, new String[] { "TABLE" });
// }
//
// /**
// * Gets the default catalog.
// *
// * @return the default catalog
// */
// /*
// * (non-Javadoc)
// *
// * @see com.jk.db.test.dynamic.meta.generator.DataBaseAnalayzer#getDefaultCatalog()
// */
// public String getDefaultCatalog() {
// try {
// return dataSource.getQueryConnection().getCatalog();
// } catch (JKDataAccessException | SQLException e) {
// JKExceptionUtil.handle(e);
// return null;
// }
// }
//
// /**
// * Builds the empty row query.
// *
// * @param catalogName the catalog typeName
// * @param tableName the table typeName
// * @return the string
// */
// protected String buildEmptyRowQuery(final String catalogName, final String tableName) {
// // TODO: check it , it has been updated it to work with H2 DB for
// // testing since h2 database schema definition is different from oracle and
// // mysql
// // and it named "public" . and i still don't know why??
// return "select * from ".concat(tableName).concat(" limit 1");
// }
//
// /*
// * (non-Javadoc)
// *
// * @see
// * com.jk.metadata.importers.MetaDataImporter#importEntity(java.lang.Object)
// */
// @Override
// public EntityMetadata importEntity(String tableName) {
// logger.debug("Importing entity ({})", tableName);
// return getTable(this.dataSource.getDatabaseName(), tableName);
// }
//
// /*
// * (non-Javadoc)
// *
// * @see
// * com.jk.metadata.importers.MetaDataImporter#importEntities(java.lang.Object,
// * java.lang.String)
// */
// @Override
// public List importEntities(Object allEntitiesSource, String sourceName) {
// return getEntitiesMetadata();
// }
//
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy