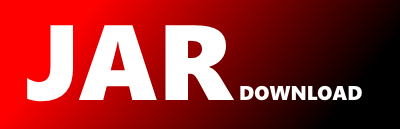
com.jk.data.dynamic.paging.DataBasePager Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.data.dynamic.paging;
import javax.sql.rowset.CachedRowSet;
import com.jk.core.util.JKDebugUtil;
import com.jk.data.dataaccess.JKDataAccessFactory;
import com.jk.data.dataaccess.core.JKDataAccessService;
import com.jk.data.datasource.JKDataSource;
// TODO: Auto-generated Javadoc
/**
* The Class DataBasePager.
*/
public class DataBasePager implements DataPager {
/**
* The main method.
*
* @param args the arguments
*/
// /////////////////////////////////////////////////////
public static void main(final String[] args) {
final DataBasePager p = new DataBasePager();
p.setQuery("SELECT * FROM GEN_NATIONAL_NUMBERS");
JKDebugUtil.printCurrentTime(1);
System.out.println(p.getAllRowsCount());
JKDebugUtil.printCurrentTime(2);
System.out.println(p.getPagesCount());
JKDebugUtil.printCurrentTime(3);
p.moveToFirstPage();
JKDebugUtil.printCurrentTime(4);
p.moveToNextPage();
JKDebugUtil.printCurrentTime(5);
p.moveToNextPage();
JKDebugUtil.printCurrentTime(6);
p.moveToNextPage();
JKDebugUtil.printCurrentTime(7);
// p.moveToNextPage();
// p.moveToNextPage();
// p.moveToNextPage();
// p.moveToLastPage();
}
/** The query. */
private String query;
/** The page rows count. */
private int pageRowsCount = -1;
/** The pages count. */
private int pagesCount;
/** The current page. */
private int currentPage;
/** The all rows count. */
private int allRowsCount;
/** The datasource. */
JKDataSource datasource;
/** The data access. */
JKDataAccessService dataAccess = JKDataAccessFactory.getDataAccessService();
/** The result set. */
private CachedRowSet resultSet;
/**
* Instantiates a new data base pager.
*/
// /////////////////////////////////////////////////////
public DataBasePager() {
}
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dynamic.paging.DataPager#getAllRowsCount()
*/
/**
* Gets the all rows count.
*
* @return the all rows count
*/
// /////////////////////////////////////////////////////
@Override
public int getAllRowsCount() {
return this.allRowsCount;
}
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dynamic.paging.DataPager#getCurrentPage()
*/
/**
* Gets the current page.
*
* @return the current page
*/
// /////////////////////////////////////////////////////
@Override
public int getCurrentPage() {
return this.currentPage;
}
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dynamic.paging.DataPager#getPageRowsCount()
*/
/**
* Gets the page rows count.
*
* @return the page rows count
*/
// /////////////////////////////////////////////////////
@Override
public int getPageRowsCount() {
return this.pageRowsCount == -1 ? JKDataAccessFactory.getDefaultDataSource().getRowsLimit() : this.pageRowsCount;
}
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dynamic.paging.DataPager#getPagesCount()
*/
/**
* Gets the pages count.
*
* @return the pages count
*/
// /////////////////////////////////////////////////////
@Override
public int getPagesCount() {
return this.pagesCount;
}
/**
* Gets the query.
*
* @return the query
*/
// /////////////////////////////////////////////////////
public String getQuery() {
return this.query;
}
/**
* Gets the result set.
*
* @return the result set
*/
public CachedRowSet getResultSet() {
return this.resultSet;
}
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dynamic.paging.DataPager#moveToFirstPage()
*/
/**
* Move to first page.
*
* @throws PagingException the paging exception
*/
// /////////////////////////////////////////////////////
@Override
public void moveToFirstPage() throws PagingException {
final int currentPage = 0;
moveToPage(currentPage);
}
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dynamic.paging.DataPager#moveToLastPage()
*/
/**
* Move to last page.
*
* @throws PagingException the paging exception
*/
// /////////////////////////////////////////////////////
@Override
public void moveToLastPage() throws PagingException {
moveToPage(getPagesCount() - 1);
}
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dynamic.paging.DataPager#moveToNextPage()
*/
/**
* Move to next page.
*
* @throws PagingException the paging exception
*/
// /////////////////////////////////////////////////////
@Override
public void moveToNextPage() throws PagingException {
moveToPage(this.currentPage + 1);
}
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dynamic.paging.DataPager#moveToPage(int)
*/
/**
* Move to page.
*
* @param page the page
*/
// /////////////////////////////////////////////////////
@Override
public void moveToPage(final int page) {
if (page >= 0 && page < getPagesCount()) {
try {
if (this.pagesCount == 1) {
this.resultSet = dataAccess.executeQueryAsCachedRowSet(this.query);
} else {
this.resultSet = dataAccess.executeQueryAsCachedRowSet(this.query, page * getPageRowsCount(),
page * getPageRowsCount() + getPageRowsCount());
}
this.currentPage = page;
// dataAccess.printRecordResultSet(resultSet);
} catch (final Exception e) {
throw new PagingException(e);
}
} else {
this.resultSet = null;
}
}
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dynamic.paging.DataPager#moveToPreviousePage()
*/
/**
* Move to previouse page.
*
* @throws PagingException the paging exception
*/
// /////////////////////////////////////////////////////
@Override
public void moveToPreviousePage() throws PagingException {
moveToPage(this.currentPage - 1);
}
/**
* Sets the datasource.
*
* @param datasource the new datasource
*/
// /////////////////////////////////////////////////////
public void setDatasource(final JKDataSource datasource) {
this.datasource = datasource;
}
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dynamic.paging.DataPager#setPageRowsCount(int)
*/
/**
* Sets the page rows count.
*
* @param pageRowsCount the new page rows count
*/
// /////////////////////////////////////////////////////
@Override
public void setPageRowsCount(final int pageRowsCount) {
this.pageRowsCount = pageRowsCount;
}
/**
* Sets the query.
*
* @param query the new query
*/
// /////////////////////////////////////////////////////
public void setQuery(final String query) {
this.query = query;
if (getPageRowsCount() == 0) {
this.pagesCount = 1;
} else {
this.allRowsCount = dataAccess.getRowsCount(query);
if (this.allRowsCount <= getPageRowsCount()) {
this.pagesCount = 1;
} else {
this.pagesCount = this.allRowsCount / getPageRowsCount();
if (this.allRowsCount % getPageRowsCount() > 0) {
this.pagesCount++;
}
}
}
moveToPage(getCurrentPage());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy