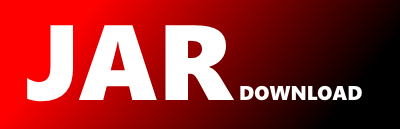
com.jk.data.dynamic.query.MetaDataSqlBuilder Maven / Gradle / Ivy
package com.jk.data.dynamic.query;
///*
// * Copyright 2002-2018 Jalal Kiswani.
// * E-mail: [email protected]
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//package com.jk.db.dynamic.query;
//
//
//import java.util.ArrayList;
//import java.util.HashMap;
//import java.util.List;
//import java.util.Map;
//import java.util.Set;
//import java.util.Vector;
//
//import com.jk.metadata.core.Field;
//import com.jk.metadata.core.EntityMetadata;
//import com.jk.metadata.core.FieldMetadata;
//import com.jk.util.JK;
//import com.jk.util.JKCollectionUtil;
//import com.jk.util.logging.JKLogger;
//import com.jk.util.logging.JKLoggerFactory;
//
//// TODO: Auto-generated Javadoc
///**
// * The Class MetaSqlBuilder.
// *
// * @author Jalal Kiswani
// */
//public class MetaDataSqlBuilder {
// static Map queryCache = new HashMap<>();
//
// /** The logger. */
// JKLogger logger = JKLoggerFactory.getLogger(getClass());
//
// /** The table meta. */
// private final EntityMetadata entityMeta;
//
// /**
// * Instantiates a new meta sql builder.
// *
// * @param entityMeta the table meta
// */
// // ////////////////////////////////////////////////////////////////////////////////////////////////
// public MetaDataSqlBuilder(final EntityMetadata entityMeta) {
// this.entityMeta = entityMeta;
// }
//
// /**
// * Builds the default report sql.
// *
// * @return the string
// */
// // ///////////////////////////////////////////////////////////////
// public String buildSelect() {
// if (this.entityMeta.isSingleRecord()) {
// return "SELECT * FROM " + this.entityMeta.getName();
// }
// boolean firstField = true;
// final StringBuffer buffer = new StringBuffer("SELECT ");
// if (this.entityMeta.getIdField() != null) {
// buffer.append(JK.NEW_LINE + this.entityMeta.getIdField().getFullQualifiedName());
// firstField = false;
// }
// final Set list = this.entityMeta.getFields();
// // Build fields
// // TODO : add support for multiple values from same table()alias support
// int foriegnKeyFieldCount = 0;
// for (final FieldMetadata fieldMetadata : list) {
//// if (fieldMetadata.isForiegnKeyField()) {
//// final ForiegnKeyFieldMetaData foriegnKeyFieldMetaData = null;//fieldMetadata;
////// foriegnKeyFieldMetaData.setAliasNamePostFix(foriegnKeyFieldCount++);
//// final String str = buildSqlFields(foriegnKeyFieldMetaData);
//// buffer.append(str);
//// } else {
//// if (firstField) {
//// firstField = false;
//// } else {
//// buffer.append(",");
//// }
//// // normal field
//// buffer.append(JK.NEW_LINE + fieldMetadata.getFullQualifiedName());
//// }
// }
// buffer.append(JK.NEW_LINE);
// // from
// buffer.append("FROM " + this.entityMeta.getName());
// // inner joins
//// final List foriegnKeyFields = this.entityMeta.getDetailFields();
//// for (final ForiegnKeyFieldMetaData foriegnKeyFieldMetaData : foriegnKeyFields) {
//// JK.implementMe();
//// //buffer.append(JK.NEW_LINE + foriegnKeyFieldMetaData.getLeftJoinStatement());
//// }
// buffer.append(";");
// return buffer.toString();
// }
//
// /**
// * Builds the default short sql.
// *
// * @return the string
// */
// // ///////////////////////////////////////////////////////////////
// public String buildShortSql() {
// JK.implementMe();
// return null;
//// final Query q = new Query();
//// q.addComponent(Keyword.SELECT);
//// final List fieldList = this.entityMeta.getFieldList();
//// q.addComponent(this.entityMeta.getIdField());
//// for (final FieldMeta fieldMeta : fieldList) {
//// q.addComponent(Operator.COMMA);
//// q.addComponent(fieldMeta);
//// }
//// q.addComponent(Keyword.FROM);
//// q.addComponent(this.entityMeta);
//// return q.compile();
// }
//
// // //
// // /////////////////////////////////////////////////////////////////////////////////////////
// // private void buildValues(StringBuffer sql, Record values) {
// // ArrayList fieldList = tableMeta.getFieldList();
// // sql.append("(");
// // if (!tableMeta.getIdField().isAutoIncrement()) {
// // sql.append(values == null ? "?" :
// // values.getIdField().getDatabaseString());
// // if (fieldList.size() > 0) {
// // sql.append(" , ");
// // }
// // }
// // for (int i = 0; i < fieldList.size(); i++) {
// // if (i > 0) {
// // sql.append(" ,");
// // }
// // sql.append(values == null ? "?" :
// // values.getField(i).getDatabaseString());
// // }
// // sql.append(" )\n");
// // }
// //
// // public String buildFatInsert(ArrayList records) {
// // StringBuffer buf = new StringBuffer();
// // buildInsert(buf);
// // for (int i = 0; i < records.size(); i++) {
// // Record record = records.get(i);
// // buildValues(buf, record);
// // if (i < records.size() - 1) {
// // buf.append(",");
// // }
// // }
// // return buf.toString();
// // }
//
// /**
// * Builds the delete.
// *
// * @return the string
// */
// // ///////////////////////////////////////////////////////////////
// public String buildDelete() {
// final StringBuffer sql = new StringBuffer();
// sql.append("DELETE FROM " + this.entityMeta.getLowerCaseNameWithUnderScores()).append(";");
// return sql.toString();
// }
//
// /**
// * Builds the delete.
// *
// * @param field the field
// * @return the string
// */
// // ///////////////////////////////////////////////////////////////
// public String buildDelete(final Field field) {
// final StringBuffer sql = new StringBuffer();
// sql.append(buildDelete());
// sql.append(" WHERE " + field.getSqlEquality());
// return sql.toString();
// }
//
// /**
// * Builds the fat insert.
// *
// * @param records the records
// * @return the string
// */
// // ////////////////////////////////////////////////////////////////////////////////////////////////
// public String buildFatInsert(final List records) {
// logger.debug("buildFatInsert()");
// final Query query = new Query();
// query.addComponent(Keyword.INSERT).addComponent(Keyword.INTO).addComponent(new TableName(this.entityMeta.getName()));
// final List fields = getFieldsInInsert(this.entityMeta.createEmptyRecord());
// query.addComponents(fields, Keyword.COMMA, true).addComponent(Keyword.VALUES);
//
// int i = 0;
// for (final Record record : records) {
// if (i++ > 0) {
// query.addComponent(Keyword.COMMA);
// }
// final IdFieldMetadata idField = this.entityMeta.getIdField();
// if (idField.isParticpateInInsert()) {
// query.addValue(record.getIdValue());
// }
// query.addValues(record.getFieldValues(), Keyword.COMMA, true);
// }
// String compiledQuery = query.compile();
// logger.debug("Query : ", compiledQuery);
// return compiledQuery;
// }
//
// /**
// * Builds the find by filter.
// *
// * @param filter the filter
// * @return the string
// */
// public String buildFindByFilter(final Record filter) {
// final StringBuffer buffer = new StringBuffer();
// buffer.append("SELECT * FROM " + this.entityMeta.getName());
// buffer.append(JK.NEW_LINE).append("WHERE 1=1 ");
// for (int i = 0; i < filter.getFieldsCount(); i++) {
// final Field field = filter.getField(i);
// if (field.getValue() != null) {
// buffer.append(" AND " + field.toSqlEquality());
// }
// }
// // +tableMeta.getIdField().getName()+" = '"+id + "'");
// return buffer.toString();
// }
//
// /**
// * Builds the find by id.
// *
// * @param id the id
// * @return the string
// */
// public String buildFindById(final String id) {
// final StringBuffer buffer = new StringBuffer();
// buffer.append("SELECT * FROM " + this.entityMeta.getName());
// buffer.append(JK.NEW_LINE).append("WHERE " + this.entityMeta.getIdField().getName() + " = '" + id + "'");
// return buffer.toString();
// }
//
// /**
// * Builds the insert.
// *
// * @param record the record
// * @return the string
// */
// // ////////////////////////////////////////////////////////////////////////////////////////////////
// public String buildInsert(final Record record) {
//// String statement = queryCache.get(record.getEntityMetaData().getName() + "-insert");
//// if (statement == null) {
// final Query query = new Query();
// query.addComponent(Keyword.INSERT);
// query.addComponent(Keyword.INTO);
// query.addComponent(new TableName(this.entityMeta.getLowerCaseNameWithUnderScores()));
//
// final List fields = getFieldsInInsert(record);
//
// query.addComponents(fields, Keyword.COMMA, true);
// query.addComponent(Keyword.VALUES);
// query.addComponent(Keyword.VARIABLE, fields.size(), Keyword.COMMA, true);
// String statement = query.compile();
//// queryCache.put(record.getEntityMetaData().getName() + "-insert", statement);
//// }
// return statement;
// }
//
// /**
// * Builds the sql fields.
// *
// * @param fieldMeta the field meta
// * @return the string
// */
// // ///////////////////////////////////////////////////////////////
// protected String buildSqlFields(final ForiegnKeyFieldMetaData fieldMeta) {
// JK.implementMe();
// return null;
//// final Vector summaryFields = fieldMeta.getReferenceTableMeta().lstSummaryFields();
//// if (summaryFields.size() == 1) {
//// return "," + JK.NEW_LINE + summaryFields.get(0).getFullQualifiedName(fieldMeta.getAliasNamePostFix());
//// }
//// // TODO: make this portable across DBMS other than mysql
//// final StringBuffer buf = new StringBuffer(",CONCAT_WS(' '");
//// for (final FieldMetadata summaryField : summaryFields) {
//// buf.append("," + summaryField.getFullQualifiedName(fieldMeta.getAliasNamePostFix()));
//// }
//// buf.append(") ");
//// return buf.toString();
// }
//
// /**
// * Builds the update.
// *
// * @param record the record
// * @return the string
// */
// public String buildUpdate(final Record record) {
//// String statement = queryCache.get(record.getEntityMetaData().getName() + "-update");
//// if (statement == null) {
// final StringBuffer sql = new StringBuffer();
// sql.append("Update " + this.entityMeta.getLowerCaseNameWithUnderScores() + JK.NEW_LINE + "SET \n ");
// for (int i = 0; i < record.getFieldsCount(); i++) {
// FieldMetadata meta = record.getField(i).getMeta();
//// if (meta.isIncludeInPersistence()) {
// if (i > 0) {
// sql.append(",").append(JK.NEW_LINE);
// }
// sql.append(escapeField(meta.getLowerCaseNameWithUnderScores()) + "=?");
//// }
// }
// sql.append(" \n WHERE " + record.getIdField().getMeta().getLowerCaseNameWithUnderScores()).append("=?;");
//// statement = sql.toString();
// return sql.toString();
//// queryCache.put(record.getEntityMetaData().getName() + "-update", statement);
//// }
//// return statement;
// }
//
// /**
// * Escape field.
// *
// * @param typeName the typeName
// * @return the string
// */
// protected String escapeField(final String typeName) {
// String scape = "";
// if (System.getProperty(JKCollectionUtil.fixPropertyKey("DB_ESCAPE_FIELDS"), "true").equals("true")) {
// scape = "`";
// }
// return scape + typeName + scape;
// }
//
// /**
// * Gets the fields in insert.
// *
// * @param record the record
// * @return the fields in insert
// */
// // ////////////////////////////////////////////////////////////////////////////////////////////////
// protected List getFieldsInInsert(final Record record) {
// final List fields = new ArrayList();
//
// final IdFieldMetadata idField = this.entityMeta.getIdField();
// if (record.getIdValueAsInteger() != 0) {
// fields.add(new FieldName(idField.getName()));
// }
//
// List fieldList = this.entityMeta.getFields();
// for (FieldMetadata fieldMetadata : fieldList) {
//// if (fieldMetadata.isIncludeInPersistence()) {
// fields.add(new FieldName(fieldMetadata.getLowerCaseNameWithUnderScores()));
//// }
// }
//
// return fields;
// }
//
// /**
// * Builds the create.
// */
// public void buildCreate() {
// // TODO Auto-generated method stub
//
// }
//
// /**
// * Builds the find by filter.
// *
// * @param filter the filter
// * @return the string
// */
// public String buildFindByFilter(List filter) {
// final StringBuffer buffer = new StringBuffer();
// buffer.append("SELECT * FROM " + this.entityMeta.getName());
// buffer.append(JK.NEW_LINE).append("WHERE 1=1 ");
// for (int i = 0; i < filter.size(); i++) {
// buffer.append(" AND " + filter.get(i));
// }
// return buffer.toString();
//
// }
//
//}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy