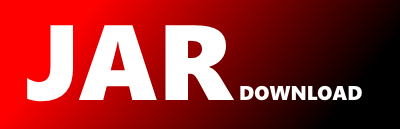
com.jk.data.vendors.mongo.JKMongoDataAccess Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.data.vendors.mongo;
import static com.mongodb.client.model.Filters.eq;
import java.util.List;
import java.util.Map;
import java.util.Vector;
import org.bson.Document;
import com.jk.core.util.JK;
import com.jk.core.util.JKObjectUtil;
import com.jk.data.dataaccess.JKDataAccessFactory;
import com.jk.data.dataaccess.nosql.JKNoSqlDataAccess;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.FindOneAndReplaceOptions;
import com.mongodb.client.model.ReturnDocument;
// TODO: Auto-generated Javadoc
/**
* The Class JKMongoDataAccess.
*/
public class JKMongoDataAccess implements JKNoSqlDataAccess {
/** The Constant ID_FIELD_NAME. */
private static final String ID_FIELD_NAME = "id";
/**
* Gets the collection.
*
* @param the generic type
* @param clas the clas
* @return the collection
*/
protected MongoCollection getCollection(Class> clas) {
clas = (Class) clas;
MongoDatabase db = JKDataAccessFactory.getNoSqlDataSource().getDatabase();
MongoCollection collection = db.getCollection(getDocumentName(clas), (Class) clas);
return collection;
}
/**
* Gets the document name.
*
* @param clas the clas
* @return the document name
*/
protected String getDocumentName(Class> clas) {
return clas.getSimpleName().toLowerCase();
}
/**
* Insert.
*
* @param the generic type
* @param obj the obj
* @return the t
*/
@Override
public T insert(T obj) {
MongoCollection collection = getCollection(obj.getClass());
collection.insertOne(obj);
return obj;
}
/**
* Gets the list.
*
* @param the generic type
* @param clas the clas
* @return the list
*/
@Override
public List getList(Class clas) {
MongoCollection collection = getCollection(clas);
List list = new Vector();
for (T t : collection.find(clas)) {
list.add(t);
}
return list;
}
/**
* Find by field name.
*
* @param the generic type
* @param clas the clas
* @param fieldName the field name
* @param value the value
* @return the list
*/
@Override
public List findByFieldName(Class clas, String fieldName, Object value) {
return getList(clas, JK.toMap(fieldName, value));
}
/**
* Gets the list.
*
* @param the generic type
* @param clas the clas
* @param filter the filter
* @return the list
*/
@Override
public List getList(Class clas, Map filter) {
MongoCollection collection = getCollection(clas);
Document document = new Document(filter);
List list = new Vector();
for (T t : collection.find(document, clas)) {
list.add(t);
}
return list;
}
/**
* Find.
*
* @param the generic type
* @param clas the clas
* @param id the id
* @return the t
*/
@Override
public T find(Class clas, Object id) {
MongoCollection collection = getCollection(clas);
return collection.find(eq("_id", id), clas).first();
}
/**
* Delete.
*
* @param the generic type
* @param clas the clas
* @param id the id
* @return the t
*/
@Override
public T delete(Class clas, Object id) {
MongoCollection collection = getCollection(clas);
return collection.findOneAndDelete(eq("_id", id));
}
/**
* Delete.
*
* @param the generic type
* @param obj the obj
* @return the t
*/
@Override
public T delete(T obj) {
MongoCollection collection = getCollection(obj.getClass());
Object fieldValue = JKObjectUtil.getFieldValue(obj, ID_FIELD_NAME);
return collection.findOneAndDelete(eq("_id", fieldValue));
}
/**
* Update.
*
* @param the generic type
* @param obj the obj
* @return the t
*/
@Override
public T update(T obj) {
return update(JKObjectUtil.getFieldValue(obj, ID_FIELD_NAME), obj);
}
/**
* Update.
*
* @param the generic type
* @param id the id
* @param obj the obj
* @return the t
*/
public T update(Object id, T obj) {
MongoCollection collection = getCollection(obj.getClass());
Document doc = new Document("_id", id);
// return record after update
FindOneAndReplaceOptions options = new FindOneAndReplaceOptions().returnDocument(ReturnDocument.AFTER);
return collection.findOneAndReplace(doc, obj, options);
}
/**
* Find one by field name.
*
* @param the generic type
* @param clas the clas
* @param fieldName the field name
* @param fieldValue the field value
* @return the t
*/
@Override
public T findOneByFieldName(Class clas, String fieldName, Object fieldValue) {
List list = getList(clas, JK.toMap(fieldName, fieldValue));
return JK.first(list);
}
/**
* Execute query.
*
* @param the generic type
* @param clas the clas
* @param queryString the query string
* @param paramters the paramters
* @return the list
*/
@Override
public List executeQuery(Class clas, String queryString, Object... paramters) {
JK.implementMe("TBD");
return null;
}
/**
* Insert or update.
*
* @param the generic type
* @param object the object
* @return the t
*/
@Override
public T insertOrUpdate(T object) {
return null;
}
/**
* Sets the max results.
*
* @param maxResults the new max results
*/
@Override
public void setMaxResults(int maxResults) {
JK.implementMe("No needed");
}
/**
* Detach.
*
* @param the generic type
* @param model the model
*/
@Override
public void detach(T model) {
JK.implementMe("No needed");
}
/**
* Clone.
*
* @param the generic type
* @param model the model
* @return the t
*/
@Override
public T clone(T model) {
JK.implementMe("No needed");
return null;
}
/**
* Start transaction.
*/
@Override
public void startTransaction() {
// TODO Auto-generated method stub
}
/**
* Close transaction.
*
* @param commit the commit
*/
@Override
public void closeTransaction(boolean commit) {
// TODO Auto-generated method stub
}
/**
* Gets the list and cache.
*
* @param the generic type
* @param clas the clas
* @return the list and cache
*/
@Override
public List getListAndCache(Class clas) {
JK.implementMe();
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy