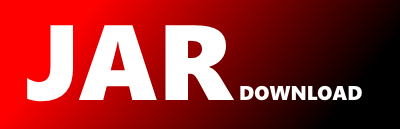
com.jk.data.vendors.oracle.JKOracleDataAccess Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.data.vendors.oracle;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Blob;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Timestamp;
import java.util.Map;
import com.jk.core.exceptions.JKDataAccessException;
import com.jk.core.util.JK;
import com.jk.data.dataaccess.core.JKDataAccessImpl;
import com.jk.data.dataaccess.core.JKFinder;
import com.jk.data.datasource.JKDataSource;
// TODO: Auto-generated Javadoc
/**
* The Class OraclDataAccess.
*/
public class JKOracleDataAccess extends JKDataAccessImpl {
/**
* Instantiates a new oracl data access.
*
* @param dataSource the data source
*/
public JKOracleDataAccess(JKDataSource dataSource) {
super(dataSource);
}
/**
* Blob to byte array.
*
* @param blob the blob
* @return the byte[]
* @throws IOException Signals that an I/O exception has occurred.
* @throws SQLException the SQL exception
*/
// /////////////////////////////////////////////////////////////////////
public static byte[] blobToByteArray(final Blob blob) throws IOException, SQLException {
final InputStream inputStream = blob.getBinaryStream();
int inByte;
byte[] returnBytes;
final ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
while ((inByte = inputStream.read()) != -1) {
byteArrayOutputStream.write(inByte);
}
returnBytes = byteArrayOutputStream.toByteArray();
return returnBytes;
}
// ///////////////////////////////////////////////////////////////////
// @Override
// public CachedRowSet executeQuery(final String query, final int fromRowIndex, final int toRowIndex) throws JKDataAccessException {
// final String sql = "SELECT * from ( select a.*, rownum r from ( " + query + ") a where rownum <= " + toRowIndex + " ) where r > "
// + fromRowIndex;
//
// return executeQuery(sql);
// }
/**
* Gets the next sequence.
*
* @param squenceName the squence name
* @return the next sequence
*/
public int getNextSequence(final String squenceName) {
final Object output = super.executeSingleOutputQuery("SELECT " + squenceName + ".NEXTVAL from DUAL");
if (output instanceof Integer || output instanceof Long) {
return new Integer(output.toString());
}
throw new JKDataAccessException(
"Unable to get sequence of sequence : " + squenceName + " , value returned " + output);
}
/**
* Gets the system date.
*
* @return the system date
*/
/*
* (non-Javadoc)
*
* @see com.jk.db.test.dataaccess.core.JKAbstractPlainDataAccess#getSystemDate()
*/
@Override
public Timestamp getSystemDate() {
final JKFinder finder = new JKFinder() {
@Override
public String getQuery() {
return "SELECT SYSTIMESTAMP FROM DUAL";
}
@Override
public Object populate(final ResultSet rs) throws SQLException {
final Timestamp date = rs.getTimestamp(1);
return date;
}
@Override
public void setParamters(final PreparedStatement ps) throws SQLException {
}
};
return (java.sql.Timestamp) findRecord(finder);
}
/**
* Checks if is duplicate key.
*
* @param e the e
* @return true, if is duplicate key
* @throws SQLException the SQL exception
*/
// /////////////////////////////////////////////////////////////////////
protected boolean isDuplicateKey(final SQLException e) throws SQLException {
return isDuplicateKey(e, true);
}
/**
* Checks if is duplicate key.
*
* @param e the e
* @param throwException the throw exception
* @return true, if is duplicate key
* @throws SQLException the SQL exception
*/
// /////////////////////////////////////////////////////////////////////
protected boolean isDuplicateKey(final SQLException e, final boolean throwException) throws SQLException {
if (e.getErrorCode() == 1) {
return true;
}
if (throwException) {
throw e;
}
return false;
}
/**
* Checks if is table exists.
*
* @param tableName the table name
* @return true, if is table exists
*/
@Override
public boolean isTableExists(String tableName) {
JK.fixMe("Find better way to do it");
try {
execute("SELECT 1 FROM ".concat(tableName));
return true;
} catch (Exception e) {
return false;
}
}
/**
* Describe table.
*
* @param name the name
* @return the map
*/
@Override
public Map describeTable(String name) {
JK.fixMe();
return super.describeTable(name);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy