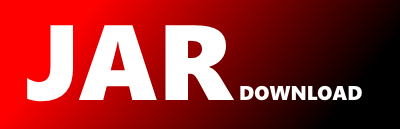
com.jk.services.client.JKServiceClientException Maven / Gradle / Ivy
/*
* Copyright 2002-2021 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.services.client;
import com.jk.core.config.JKConfig;
import com.jk.core.config.JKConstants;
import com.jk.core.exceptions.JKException;
import com.jk.core.http.JKHttpStatus;
import com.jk.core.util.JK;
// TODO: Auto-generated Javadoc
/**
* The Class JKServiceClientException.
*/
public class JKServiceClientException extends JKException {
/** The service name. */
String serviceName;
/** The error code. */
String errorCode;
/** The error message. */
String errorMessage;
/** The status. */
private JKHttpStatus status;
/** The url. */
private String url;
/** The response message. */
private String responseMessage;
/**
* Instantiates a new JK service client exception.
*/
public JKServiceClientException() {
super();
}
/**
* Instantiates a new JK service client exception.
*
* @param message the message
* @param cause the cause
* @param enableSuppression the enable suppression
* @param writableStackTrace the writable stack trace
*/
public JKServiceClientException(String message, Throwable cause, boolean enableSuppression,
boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
}
/**
* Instantiates a new JK service client exception.
*
* @param message the message
* @param cause the cause
*/
public JKServiceClientException(String message, Throwable cause) {
super(message, cause);
}
/**
* Instantiates a new JK service client exception.
*
* @param message the message
*/
public JKServiceClientException(String message) {
super(message);
}
/**
* Instantiates a new JK service client exception.
*
* @param cause the cause
*/
public JKServiceClientException(Throwable cause) {
super(cause);
}
/**
* Instantiates a new JK service client exception.
*
* @param status the status
*/
public JKServiceClientException(JKHttpStatus status) {
this.status = status;
}
/**
* Instantiates a new JK service client exception.
*
* @param serviceName the service name
* @param url the url
* @param status the status
* @param responseMessage the response message
* @param cause the cause
*/
public JKServiceClientException(String serviceName, String url, JKHttpStatus status, String responseMessage,Throwable cause) {
super(cause);
this.serviceName = serviceName;
this.url = url;
this.status = status;
this.responseMessage = responseMessage;
}
/**
* Instantiates a new JK service client exception.
*
* @param serviceName the service name
* @param url the url
* @param status the status
* @param responseMessage the response message
*/
public JKServiceClientException(String serviceName, String url, JKHttpStatus status, String responseMessage) {
this.serviceName = serviceName;
this.url = url;
this.status = status;
this.responseMessage = responseMessage;
}
/**
* Gets the message.
*
* @return the message
*/
@Override
public String getMessage() {
StringBuffer buffer = new StringBuffer();
if (serviceName != null) {
buffer.append("Service: ").append(serviceName).append("\t");
}
if (status != null) {
buffer.append("Status: ").append(status).append("\t");
}
if (url != null && JKConfig.get().getPropertyAsBoolean(JKConstants.Microservices.SHOW_URL_ON_ERROR, true)) {
buffer.append(" URL : ").append(url);
}
if (responseMessage != null) {
buffer.append(JK.NEW_LINE).append("Message: ").append(JK.NEW_LINE).append(responseMessage).append("\t");
}
// if(responseMessage!=null) {
// buffer.append(" Response Message: ").append(responseMessage);
// }
if(buffer.length()>0) {
return buffer.toString();
}
return super.getMessage();
}
/**
* Gets the service name.
*
* @return the service name
*/
public String getServiceName() {
return serviceName;
}
/**
* Sets the service name.
*
* @param serviceName the new service name
*/
public void setServiceName(String serviceName) {
this.serviceName = serviceName;
}
/**
* Gets the error code.
*
* @return the error code
*/
public String getErrorCode() {
return errorCode;
}
/**
* Sets the error code.
*
* @param errorCode the new error code
*/
public void setErrorCode(String errorCode) {
this.errorCode = errorCode;
}
/**
* Gets the error message.
*
* @return the error message
*/
public String getErrorMessage() {
return errorMessage;
}
/**
* Sets the error message.
*
* @param errorMessage the new error message
*/
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
/**
* Gets the status.
*
* @return the status
*/
public JKHttpStatus getStatus() {
return status;
}
/**
* Sets the status.
*
* @param status the new status
*/
public void setStatus(JKHttpStatus status) {
this.status = status;
}
/**
* Gets the url.
*
* @return the url
*/
public String getUrl() {
return url;
}
/**
* Sets the url.
*
* @param url the new url
*/
public void setUrl(String url) {
this.url = url;
}
/**
* Gets the response message.
*
* @return the response message
*/
public String getResponseMessage() {
return responseMessage;
}
/**
* Sets the response message.
*
* @param responseMessage the new response message
*/
public void setResponseMessage(String responseMessage) {
this.responseMessage = responseMessage;
}
public boolean isNotFound() {
return getStatus()==JKHttpStatus.NOT_FOUND;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy