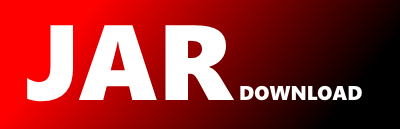
com.jk.services.client.logging.JKLogServiceClient Maven / Gradle / Ivy
/*
*
*/
package com.jk.services.client.logging;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.Executor;
import java.util.concurrent.Executors;
import com.jk.core.config.JKConfigEvent;
import com.jk.core.config.JKConfig;
import com.jk.core.config.JKConfigListener;
import com.jk.core.config.JKConstants;
import com.jk.core.logging.JKLog;
import com.jk.core.logging.JKLogger;
import com.jk.core.logging.JKLoggerFactory;
import com.jk.core.util.JK;
import com.jk.core.util.JKValidationUtil;
import com.jk.services.client.JKServiceClient;
import junit.framework.Assert;
// TODO: Auto-generated Javadoc
/**
* The Class JKLogServiceClient.
*/
public class JKLogServiceClient {
static {
JKConfig.addListner(new JKConfigListener() {
@Override
public void reloadConfig(JKConfigEvent event) {
enabled=null;
runAsync=null;
}
@Override
public void closeConfig(JKConfigEvent event) {
}
});
}
/** The enabled. */
static Boolean enabled;
/** The run async. */
static Boolean runAsync;
/** The logger. */
JKLogger logger = JKLoggerFactory.getLogger(getClass());
/** The log client. */
JKServiceClient logClient;
/**
* Instantiates a new JK log service client.
*/
public JKLogServiceClient() {
String base = JK.getProperty(JKConstants.Microservices.LOG_SERVICE_BASE);
if (isEnabled() && JKValidationUtil.isAnyEmpty(base)) {
JK.exception("Please set the logging service base: " + JKConstants.Microservices.LOG_SERVICE_BASE);
}
logClient = new JKServiceClient<>(base);
logClient.setEnableRemoteLogging(false);//to avoid recursion
logClient.setModelClass(JKLog.class);
}
/**
* Call trace.
*
* @param message the message
*/
public void callTrace(String message) {
if (isEnabled())
callAddLog(message, JKLog.TRACE);
}
/**
* Call debug.
*
* @param message the message
*/
public void callDebug(String message) {
if (isEnabled())
callAddLog(message, JKLog.DEBUG);
}
/**
* Call info.
*
* @param message the message
*/
public void callInfo(String message) {
if (isEnabled())
callAddLog(message, JKLog.INFO);
}
/**
* Call warn.
*
* @param message the message
*/
public void callWarn(String message) {
if (isEnabled())
callAddLog(message, JKLog.WARN);
}
/**
* Call error.
*
* @param message the message
*/
public void callError(String message) {
if (isEnabled())
callAddLog(message, JKLog.ERROR);
}
/**
* Call add log.
*
* @param message the message
* @param severity the severity
*/
public void callAddLog(String message, String severity) {
if (isEnabled()) {
//it should be here to be able to get the context information
JKLog log = new JKLog(JK.getAppName(), message, severity);
Runnable runnable = () -> {
try {
logClient.callJsonWithPost("crosscutting/logging", log);
} catch (Exception e) {
e.printStackTrace();
logger.error("Unable to log to the Logging Microservice for the following reason:" + e.getMessage());
}
};
if (getRunAsync()) {
JK.runAsync(runnable);
} else {
runnable.run();
}
} else {
logger.info("Log Service Client is not enabled, you can enable it by by setting ({}) variable",
JKConstants.Microservices.LOG_SERVICE_ENABLED);
}
}
/**
* Gets the app log count.
*
* @param appName the app name
* @param reset the reset
* @return the app log count
*/
public Integer getAppLogCount(String appName, boolean reset) {
JK.fixMe("secure me");
if (isEnabled()) {
String response = logClient.callJsonAsString(String.format("crosscutting/logging/logs/count/%s/%s", appName, reset));
return new Integer(response);
}
return null;
}
/**
* Gets the app logs.
*
* @param appName the app name
* @param reset the reset
* @return the app logs
*/
public List getAppLogs(String appName, boolean reset) {
JK.fixMe("secure me");
if (isEnabled()) {
List response = logClient.callJsonAsListOfObjects(String.format("crosscutting/logging/logs/%s/%s", appName, reset));
return response;
}
return Collections.EMPTY_LIST;
}
/**
* Checks if is enabled.
*
* @return true, if is enabled
*/
public static boolean isEnabled() {
if (enabled == null) {
enabled = JKConfig.get().getPropertyAsBoolean(JKConstants.Microservices.LOG_SERVICE_ENABLED, false);
}
return enabled;
}
/**
* Gets the run async.
*
* @return the run async
*/
public static Boolean getRunAsync() {
if (runAsync == null) {
runAsync = JKConfig.get().getPropertyAsBoolean(JKConstants.Microservices.LOG_SERVICE_RUN_ASYNC, true);
}
return runAsync;
}
/**
* Sets the run async.
*
* @param runAsync the new run async
*/
public static void setRunAsync(Boolean runAsync) {
JKLogServiceClient.runAsync = runAsync;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy