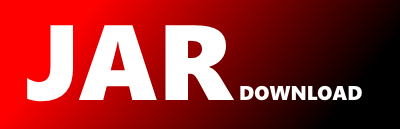
com.jk.services.crosscutting.logging.JKLogCrossCuttingService Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.services.crosscutting.logging;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Vector;
import com.jk.core.context.JKContextFactory;
import com.jk.core.http.JKHttpStatus;
import com.jk.core.logging.JKLog;
import com.jk.core.logging.JKLogger;
import com.jk.core.logging.JKLoggerFactory;
import com.jk.services.server.JKAbstractRestController;
import jakarta.inject.Singleton;
import jakarta.ws.rs.GET;
import jakarta.ws.rs.POST;
import jakarta.ws.rs.Path;
import jakarta.ws.rs.PathParam;
import jakarta.ws.rs.core.Response;
// TODO: Auto-generated Javadoc
/**
* Make this endpoint protected The Class HiService.
*/
@Path("/logging")
@Singleton
public class JKLogCrossCuttingService extends JKAbstractRestController {
/** The all logs. */
Map> allLogs = new HashMap<>();
/**
* Adds the.
*
* @param model the model
* @return the response
*/
@POST
public Response add(JKLog model) {
String remoteAppName = JKContextFactory.getCurrentContext().getRemoteAppName();
JKLogger logger = JKLoggerFactory.getLogger(model.getName());
switch (model.getSeverity()) {
case JKLog.TRACE:
logger.trace(model.toLogMessage());
break;
case JKLog.DEBUG:
logger.debug(model.toLogMessage());
break;
case JKLog.INFO:
logger.info(model.toLogMessage());
break;
case JKLog.WARN:
cacheLog(remoteAppName, model);
logger.warn(model.toLogMessage());
break;
case JKLog.ERROR:
cacheLog(remoteAppName, model);
logger.error(model.toLogMessage());
break;
default:
logger.info(model.toLogMessage());
}
return Response.status(JKHttpStatus.CREATED.value()).entity(model).build();
}
/**
* Gets the logs count.
*
* @param appName the app name
* @param resetCounter the reset counter
* @return the logs count
*/
@GET
@Path("/logs/count/{app-name}/{reset-counter}")
public Response getLogsCount(@PathParam("app-name") String appName, @PathParam("reset-counter") boolean resetCounter) {
List list = allLogs.get(appName);
int count = 0;
if (list != null) {
count = list.size();
if (resetCounter) {
allLogs.remove(appName);
}
}
return Response.ok(count).build();
}
/**
* Gets the logs.
*
* @param appName the app name
* @param resetCounter the reset counter
* @return the logs
*/
@GET
@Path("/logs/{app-name}/{reset-counter}")
public Response getLogs(@PathParam("app-name") String appName, @PathParam("reset-counter") boolean resetCounter) {
List list = allLogs.get(appName);
if (list != null) {
if (resetCounter) {
allLogs.remove(appName);
}
} else {
list = Collections.EMPTY_LIST;
}
return Response.ok(list).build();
}
/**
* Cache log.
*
* @param remoteAppName the remote app name
* @param model the model
*/
protected void cacheLog(String remoteAppName, JKLog model) {
if (remoteAppName == null) {
return;
}
List logs = allLogs.get(remoteAppName);
if (logs == null) {
logs = new Vector();
allLogs.put(remoteAppName, logs);
}
logs.add(model);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy