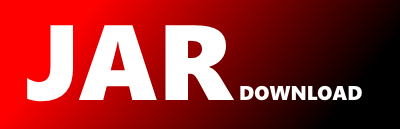
com.jk.webstack.controllers.JKWebAppBaseController Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.webstack.controllers;
import org.keycloak.KeycloakPrincipal;
import org.keycloak.KeycloakSecurityContext;
import org.keycloak.representations.IDToken;
import com.jk.core.util.JK;
import com.jk.web.faces.controllers.JKWebController;
import com.jk.webstack.services.email.EmailService;
import com.jk.webstack.services.logging.ActionLogsService;
// TODO: Auto-generated Javadoc
/**
* The Class JKAppBaseManagedBean.
*/
public class JKWebAppBaseController extends JKWebController {
/**
* Gets the user name.
*
* @return the user name
*/
public String getUserName() {
IDToken idToken = getIdToken();
if (idToken == null) {
return null;
}
return idToken.getPreferredUsername();
}
/**
* Gets the first name.
*
* @return the first name
*/
public String getFirstName() {
IDToken idToken = getIdToken();
if (idToken == null) {
return null;
}
return idToken.getGivenName();
}
/**
* Gets the family name.
*
* @return the family name
*/
public String getFamilyName() {
IDToken idToken = getIdToken();
if (idToken == null) {
return null;
}
return idToken.getFamilyName();
}
/**
* Gets the email.
*
* @return the email
*/
public String getEmail() {
IDToken idToken = getIdToken();
if (idToken == null) {
return null;
}
return idToken.getEmail();
}
/**
* Gets the id token.
*
* @return the id token
*/
public IDToken getIdToken() {
KeycloakPrincipal userPrincipal = (KeycloakPrincipal) request().getUserPrincipal();
if (userPrincipal == null) {
return null;
}
KeycloakPrincipal kp = (KeycloakPrincipal) userPrincipal;
IDToken idToken = kp.getKeycloakSecurityContext().getIdToken();
return idToken;
}
/**
* Checks if is user logged in.
*
* @return true, if is user logged in
*/
public boolean isUserLoggedIn() {
return getUserName() != null;
}
/**
* Log action.
*
* @param logType the log type
*/
public void logAction(String logType) {
String className = getActionLogName();
String actionName = logType;
boolean userLoggedIn = isUserLoggedIn();
String userName = getUserName();
Runnable command = () -> {
logger.info("Adding new logAction(type)");
getActionLogService().logAction(actionName);
if (userLoggedIn) {
getActionLogService().logUserAction(className, actionName, userName);
}
};
executeAsyc(command);
}
/**
* Gets the action log name.
*
* @return the action log name
*/
protected String getActionLogName() {
return getClass().getSimpleName();
}
/**
* Gets the action log service.
*
* @return the action log service
*/
protected ActionLogsService getActionLogService() {
return new ActionLogsService();
}
/**
* Gets the email service.
*
* @return the email service
*/
protected EmailService getEmailService() {
return new EmailService();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy