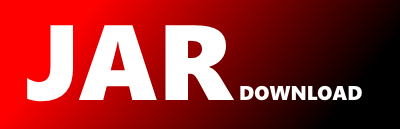
com.jk.webstack.controllers.JKWebControllerWithOrmSupport Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.webstack.controllers;
import java.util.List;
import com.jk.core.config.JKConstants;
import com.jk.core.factory.JKFactory;
import com.jk.core.test.JKMockUtil;
import com.jk.core.util.JK;
import com.jk.core.util.JKCollectionUtil;
import com.jk.core.util.JKObjectUtil;
import com.jk.data.dataaccess.orm.JKObjectDataAccess;
import com.jk.data.dataaccess.orm.JKObjectDataAccessImpl;
import com.jk.services.client.workflow.JKWorkflowServiceClient;
import com.jk.services.client.workflow.JKWorkflowServiceClient.UserAction;
import com.jk.services.client.workflow.JKWorkflowUtil;
import com.jk.services.client.workflow.models.NewPayloadRequestModel;
import com.jk.services.client.workflow.models.PayloadModel;
import com.jk.services.client.workflow.models.WorkflowEntityModel;
import com.jk.webstack.services.workflow.WorkflowController;
// TODO: Auto-generated Javadoc
/**
* The Class JKManagedBeanWithOrmSupport.
*
* @param the generic type
*/
public abstract class JKWebControllerWithOrmSupport extends JKWebControllerWithSqlDataAccess {
/** The model. */
private T model;
/** The oruginal model, which will be used in workflow. */
private T original;
/** The model class. */
private Class modelClass;
/** The model list. */
private List modelList;
/** The filter list. */
private List filterList;
/** In case required for another datatable. */
private List filterList2;
/** The always refresh list. */
private boolean alwaysRefreshList;
/** The mode. */
protected ControllerMode mode;
/** The confirm reeset. */
private boolean confirmReeset;
/** The edit tabular. */
boolean editTabular;
/** The client. */
JKWorkflowServiceClient client = new JKWorkflowServiceClient();
/** The workflow avialable. */
boolean workflowAvialable;
/** The payload. */
PayloadModel payload;
/** The payloads. */
List payloads;
/** The workflow entity. */
private WorkflowEntityModel workflowEntity;
/**
* Instantiates a new JK managed bean with orm support.
*/
public JKWebControllerWithOrmSupport() {
modelClass = JKFactory.type(JKObjectUtil.getGenericClassFromParent(this));
mode = ControllerMode.ADD;
workflowAvialable = JKWorkflowUtil.isWorkflowAvialable();
if (workflowAvialable) {
client.getSystem(JK.getAppName());// to sync App Name;
workflowEntity = client.getWorkflowEntity(JK.getAppName(), modelClass.getSimpleName(),
modelClass.getName());
}
}
/**
* Adds the.
*
* @return the string
*/
public String addToDatabase() {
if (model == null) {
throw new IllegalStateException("Model is null while calling merge");
}
beforeInsert();
T model = this.model;
getDataAccess().insert(model);
afterInsert();
modelList = null;
// reset();
find(getIdValue(model));
success("Added successfully", false);
return null;
}
/**
* Edits the.
*
* @return the string
*/
public String edit() {
mode = ControllerMode.EDIT;
return null;
}
/**
* Save.
*
* @return the string
*/
public String saveToDatabase() {
if (model == null) {
throw new IllegalStateException("Model is null while calling merge");
}
beforeUpdate();
T model = this.model;
getDataAccess().update(model);
reset();
afterUpdate();
find(getIdValue(model));
success("Updated successfully", true);
return null;
}
/**
* Before insert.
*/
protected void beforeInsert() {
}
/**
* After insert.
*/
protected void afterInsert() {
}
/**
* Before update.
*/
protected void beforeUpdate() {
}
/**
* After update.
*/
protected void afterUpdate() {
}
/**
* Before delete.
*/
protected void beforeDelete() {
}
/**
* Find.
*
* @param id the id
* @return the string
*/
public String find(int id) {
T model = (T) getDataAccess().find(getModelClass(), id);
setModel(model);
mode = ControllerMode.READONLY;
return null;
}
/**
* Gets the model class.
*
* @return the model class
*/
protected Class getModelClass() {
return modelClass;
}
/**
* Gets the model list.
*
* @return the model list
*/
public List getModelList() {
if (modelList == null) {
modelList = getDataAccess().getList(getModelClass());
}
return modelList;
}
/**
* Delete.
*
* @return the string
*/
// ///////////////////////////////////////////////////
public String deleteFromDatabase() {
beforeDelete();
getDataAccess().delete(getModelClass(), getIdValue());
afterDelete();
reset();
success("Deleted successfully", false);
return null;
}
/**
* After delete.
*/
protected void afterDelete() {
}
/**
* Gets the id value.
*
* @return the id value
*/
public Integer getIdValue() {
return getIdValue(getModel());
}
/**
* Gets the id value.
*
* @param model the model
* @return the id value
*/
protected Integer getIdValue(T model) {
return (Integer) JKObjectUtil.getFieldValue(model, JKConstants.Database.DEFAULT_PRIMARY_KEY_FIELD_NAME);
}
/**
* Gets the model.
*
* @return the model
*/
public T getModel() {
if (model == null) {
model = createEmptyModel();
}
return model;
}
/**
* Creates the empty model.
*
* @return the t
*/
protected T createEmptyModel() {
return JKObjectUtil.newInstance(getModelClass());
}
/**
* Sets the model.
*
* @param model the new model
*/
public void setModel(T model) {
// to avoid loosing any local views value
if (model != this.model) {
this.model = model;
if (model == null) {
mode = ControllerMode.ADD;
} else {
mode = ControllerMode.READONLY;
}
}
}
/**
* Gets the data access.
*
* @return the data access
*/
// ///////////////////////////////////////////////////
protected JKObjectDataAccess getDataAccess() {
return JKFactory.instance(JKObjectDataAccessImpl.class);
}
/**
* Reset.
*
* @return the string
*/
// ///////////////////////////////////////////////////
public String reset() {
this.model = null;
this.modelList = null;
payloads = null;
original = null;
mode = ControllerMode.ADD;
if (isWorkflowAvialable()) {
resetWorkFlow();
}
return null;
}
/**
* Reset work flow.
*/
protected void resetWorkFlow() {
String controllerName = "workflow";
WorkflowController workflow=getViewScopedManagedBean(controllerName);
if (workflow != null) {
workflow.reset();
updateUi("frmNotifications");
}
}
/**
* Checks if is always refresh list.
*
* @return true, if is always refresh list
*/
// ///////////////////////////////////////////////////
public boolean isAlwaysRefreshList() {
return alwaysRefreshList;
}
/**
* Sets the always refresh list.
*
* @param alwaysRefreshList the new always refresh list
*/
public void setAlwaysRefreshList(boolean alwaysRefreshList) {
this.alwaysRefreshList = alwaysRefreshList;
}
/**
* Sets the id value.
*
* @param value the new id value
*/
public void setIdValue(Object value) {
JKObjectUtil.setPeopertyValue(getModel(), JKConstants.Database.DEFAULT_PRIMARY_KEY_FIELD_NAME, value);
}
/**
* Duplicate.
*
* @return the string
*/
public String duplicate() {
model = getDataAccess().clone(getModel());
setIdValue(0);
mode = ControllerMode.ADD;
return null;
}
/**
* Gets the empty model.
*
* @return the empty model
*/
public T getEmptyModel() {
return JKFactory.instance(modelClass);
}
/**
* Fill.
*
* @return the string
*/
public String fill() {
JKMockUtil.fillFields(getModel());
return null;
}
/**
* Checks if is allow add.
*
* @return true, if is allow add
*/
public boolean isAllowAdd() {
return mode == ControllerMode.ADD;
}
/**
* Checks if is allow edit.
*
* @return true, if is allow edit
*/
public boolean isAllowEdit() {
return mode == ControllerMode.READONLY;
}
/**
* Checks if is allow save.
*
* @return true, if is allow save
*/
public boolean isAllowSave() {
return mode == ControllerMode.EDIT;
}
/**
* Checks if is allow delete.
*
* @return true, if is allow delete
*/
public boolean isAllowDelete() {
return mode == ControllerMode.EDIT;
}
/**
* Checks if is allow reset.
*
* @return true, if is allow reset
*/
public boolean isAllowReset() {
return getMode() != ControllerMode.WORKFLOW;
}
/**
* Checks if is allow fill.
*
* @return true, if is allow fill
*/
public boolean isAllowFill() {
return isAllowAdd() && isDevelopmentMode();
}
/**
* Checks if is edits the mode.
*
* @return true, if is edits the mode
*/
public boolean isEditMode() {
return mode == ControllerMode.EDIT;
}
/**
* Cancel edit.
*/
public void cancelEdit() {
mode = ControllerMode.READONLY;
}
/**
* Checks if is read only mode.
*
* @return true, if is read only mode
*/
public boolean isReadOnlyMode() {
return mode == ControllerMode.READONLY;
}
/**
* Gets the filter list.
*
* @return the filter list
*/
public List getFilterList() {
return filterList;
}
/**
* Sets the filter list 2.
*
* @param filterList2 the new filter list 2
*/
public void setFilterList2(List filterList2) {
this.filterList2 = filterList2;
}
/**
* Sets the filter list.
*
* @param filterList the new filter list
*/
public void setFilterList(List filterList) {
this.filterList = filterList;
}
/**
* Gets the filter list 2.
*
* @return the filter list 2
*/
public List getFilterList2() {
return filterList2;
}
/**
* Checks if is confirm reeset.
*
* @return true, if is confirm reeset
*/
public boolean isConfirmReeset() {
return confirmReeset;
}
/**
* Sets the confirm reeset.
*
* @param confirmReeset the new confirm reeset
*/
public void setConfirmReeset(boolean confirmReeset) {
this.confirmReeset = confirmReeset;
}
/**
* Gets the mode.
*
* @return the mode
*/
public ControllerMode getMode() {
return mode;
}
/**
* Checks if is edits the tabular.
*
* @return true, if is edits the tabular
*/
public boolean isEditTabular() {
return editTabular;
}
/**
* Sets the edits the tabular.
*
* @param editTabular the new edits the tabular
*/
public void setEditTabular(boolean editTabular) {
this.editTabular = editTabular;
}
/**
* Save all.
*/
public void saveAllToDatabase() {
List list = getModelList();
for (T model : list) {
getDataAccess().update(model);
}
success("Saved succesfully", false);
}
/**
* Adds the.
*
* @return the string
*/
public String add() {
if (isWorkflowAvialable()) {
// @formatter:off
client.insert(
new NewPayloadRequestModel().
withEntity(getWorkflowEntityName()).
withAction(UserAction.CREATE.toString()).
withBody(JKObjectUtil.toJson(getModel())).
withSystem(JK.getAppName()));
// @formatter:on
reset();
success("Record sent for the needed approvals");
} else {
return addToDatabase();
}
return null;
}
/**
* Save.
*
* @return the string
*/
public String save() {
if (isWorkflowAvialable()) {
// @formatter:off
client.insert(
new NewPayloadRequestModel().
withEntity(getWorkflowEntityName()).
withAction(UserAction.MODIFY.toString()).
withBody(JKObjectUtil.toJson(getModel())).
withSystem(JK.getAppName()));
// @formatter:on
reset();
success("Record sent for the needed approvals");
} else {
return saveToDatabase();
}
return null;
}
/**
* Save all.
*
* @return the string
*/
public String saveAll() {
if (isWorkflowAvialable()) {
List list = getModelList();
for (T model : list) {
// @formatter:off
client.insert(
new NewPayloadRequestModel().
withEntity(getWorkflowEntityName()).
withAction(UserAction.MODIFY.toString()).
withBody(JKObjectUtil.toJson(model)).
withSystem(JK.getAppName()));
// @formatter:on
}
reset();
success("Record sent for the needed approvals");
} else {
saveAllToDatabase();
setEditTabular(false);
}
return null;
}
/**
* Delete.
*
* @return the string
*/
public String delete() {
if (isWorkflowAvialable()) {
// @formatter:off
client.insert(
new NewPayloadRequestModel().
withEntity(getWorkflowEntityName()).
withAction(UserAction.DELETE.toString()).
withBody(JKObjectUtil.toJson(getModel())).
withSystem(JK.getAppName()));
// @formatter:on
reset();
success("Record sent for the needed approvals");
} else {
return deleteFromDatabase();
}
return null;
}
/**
* Gets the payloads.
*
* @return the payloads
*/
public List getPayloads() {
if (payloads == null) {
payloads = client.getPayLoads(JK.getAppName(), getWorkflowEntityName(),
JKCollectionUtil.toString(getRoles(), false));
}
return payloads;
}
/**
* Sets the payloads.
*
* @param payloads the new payloads
*/
public void setPayloads(List payloads) {
this.payloads = payloads;
}
/**
* Gets the workflow entity name.
*
* @return the workflow entity name
*/
protected String getWorkflowEntityName() {
return getModelClass().getSimpleName();
}
/**
* Checks if is workflow avialable.
*
* @return true, if is workflow avialable
*/
public boolean isWorkflowAvialable() {
return workflowAvialable && workflowEntity != null;
}
/**
* Gets the payload.
*
* @return the payload
*/
public PayloadModel getPayload() {
return payload;
}
/**
* Sets the payload.
*
* @param payload the new payload
*/
public void setPayload(PayloadModel payload) {
this.payload = payload;
}
/**
* Approve payload.
*
* @param id the id
* @return the string
*/
public String approvePayload(int id) {
PayloadModel payload = findPayload(id);
if (payload == null) {
JK.exception("Payload with id ({}) not found." + id);
}
String approveAction = client.approveWorkflowEntity(id);
if (approveAction.equals("INSERT")) {
setModel(JKObjectUtil.jsonToObject(payload.getPayload(), modelClass));
addToDatabase();
}
if (approveAction.equals("UPDATE")) {
setModel(JKObjectUtil.jsonToObject(payload.getPayload(), modelClass));
saveToDatabase();
}
if (approveAction.equals("DELETE")) {
setModel(JKObjectUtil.jsonToObject(payload.getPayload(), modelClass));
deleteFromDatabase();
}
reset();
return null;
}
/**
* Find payload.
*
* @param id the id
* @return the payload model
*/
public PayloadModel findPayload(int id) {
for (PayloadModel payload : getPayloads()) {
if (payload.getId().equals(id)) {
return payload;
}
}
return null;
}
/**
* Reject payload.
*
* @param id the id
* @return the string
*/
public String rejectPayload(int id) {
String rejectAction = client.rejectWorkflowEntity(id);
reset();
return null;
}
/**
* View payload.
*
* @param id the id
* @return the string
*/
public String viewPayload(int id) {
this.payload = findPayload(id);
mode = ControllerMode.WORKFLOW;
this.model = (T) payload.getObject();
return null;
}
/**
* Checks if is input disabled.
*
* @return true, if is input disabled
*/
public boolean isInputDisabled() {
return mode == ControllerMode.READONLY || mode == ControllerMode.WORKFLOW;
}
/**
* Gets the original.
*
* @return the original
*/
public T getOriginal() {
if (getPayload() != null && getPayload().getObject() != null) {
int index = getModelList().indexOf(getPayload().getObject());
if (index != -1) {
original = getModelList().get(index);
}
}
return original;
}
/**
* Sets the original.
*
* @param original the new original
*/
public void setOriginal(T original) {
this.original = original;
}
/**
* Checks if is field modified.
*
* @param name the name
* @return true, if is field modified
*/
public boolean isFieldModified(String name) {
if (getMode() != ControllerMode.ADD && getOriginal() != null) {
Object newValue = JKObjectUtil.getFieldValue(getModel(), name);
Object originalValue = JKObjectUtil.getFieldValue(getOriginal(), name);
if (newValue != null) {
return !newValue.equals(originalValue);
}
if (originalValue != null) {
return !originalValue.equals(newValue);
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy