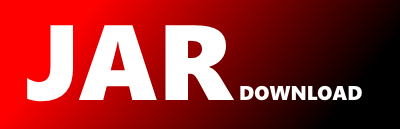
com.jk.webstack.security.JKWebSecurityConfig Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.webstack.security;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationProvider;
import org.springframework.security.authentication.dao.DaoAuthenticationProvider;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.security.web.SecurityFilterChain;
import org.springframework.security.web.firewall.DefaultHttpFirewall;
import org.springframework.security.web.firewall.HttpFirewall;
import org.springframework.security.web.session.HttpSessionEventPublisher;
import com.jk.core.config.JKConfig;
import com.jk.core.config.JKConstants;
import com.jk.core.logging.JKLogger;
import com.jk.core.logging.JKLoggerFactory;
import com.jk.webstack.security.services.SecurityService;
import jakarta.servlet.ServletContext;
/**
* The Class WebSecurityConfig.
*/
@EnableWebSecurity
@Configuration
public class JKWebSecurityConfig {
/** The logger. */
JKLogger logger = JKLoggerFactory.getLogger(getClass());
/** The enabled. */
private boolean enabled = JKConfig.getDefaultInstance().getProperty(JKConstants.App.ENABLE_SECURITY, "false")
.equals("true");
/** The context. */
@Autowired
ServletContext context;
/** The public urls. */
private String[] publicUrls = JKConfig.get().getProperty(JKConstants.WEB.SECURITY_PUBLIC_URL, JKConstants.WEB.DEFAULT_PUBLIC_URLS)
.split(",");
/**
* Configure.
*
* @param http the http
* @throws Exception the exception
*/
@Bean
public SecurityFilterChain configure(HttpSecurity http) throws Exception {
logger.info(enabled ? "WebSecurity is Enabled" : "WebSecurity is Disabled");
if (enabled) {
// @formatter:off
http.csrf().disable()
.authorizeHttpRequests()
.requestMatchers(publicUrls).permitAll()
.requestMatchers("/admin/**").hasAnyAuthority("admin")
.requestMatchers("/*/components/**").denyAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginProcessingUrl("/login")
.loginPage("/login/index.xhtml")
.defaultSuccessUrl("/")
.failureUrl("/login/")
.permitAll()
.and()
.logout()
.logoutUrl("/logout")
.logoutSuccessUrl("/")
.deleteCookies("JSESSIONID")
.permitAll()
.and()
.exceptionHandling()
.accessDeniedPage("/error/403.xhtml");
// @formatter:on
} else {
http.csrf().disable().authorizeHttpRequests().requestMatchers("**").permitAll();
}
return http.build();
}
/**
* Configure global.
*
* @param auth the auth
* @throws Exception the exception
*/
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
if (enabled)
auth.authenticationProvider(authenticationProvider());
}
/**
* Authentication provider.
*
* @return the authentication provider
*/
@Bean
public AuthenticationProvider authenticationProvider() {
if (enabled) {
DaoAuthenticationProvider authProvider = new DaoAuthenticationProvider();
SecurityService service = new SecurityService();
authProvider.setUserDetailsService(service);
authProvider.setPasswordEncoder(encoder());
//authProvider.setAuthoritiesMapper(new MyAuthMapper());
return authProvider;
} else {
return new NullAuthenticationProvider();
}
}
/**
* Encoder.
*
* @return the password encoder
*/
@Bean
public PasswordEncoder encoder() {
return new BCryptPasswordEncoder();
}
/**
* Default http firewall.
*
* @return the http firewall
*/
@Bean
public HttpFirewall defaultHttpFirewall() {
return new DefaultHttpFirewall();
}
/**
* Gets the servlet context.
*
* @return the servlet context
*/
@Bean
public ServletContext getServletContext() {
return context;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy