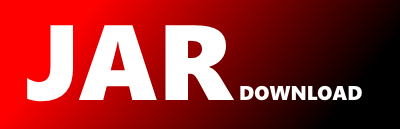
com.jk.webstack.security.User Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.webstack.security;
import java.io.Serializable;
import java.util.Collection;
import java.util.Vector;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.userdetails.UserDetails;
import jakarta.persistence.Column;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import jakarta.persistence.JoinColumn;
import jakarta.persistence.Table;
import jakarta.persistence.Transient;
import lombok.Data;
// TODO: Auto-generated Javadoc
/**
* The Class User.
*/
@Entity
@Table(name = "sec_users")
/**
* Instantiates a new user.
*/
/**
* Instantiates a new user.
*/
/**
* Instantiates a new user.
*/
/**
* Instantiates a new user.
*/
/**
* Instantiates a new user.
*/
/**
* Instantiates a new user.
*/
/**
* Instantiates a new user.
*/
@Data
public class User implements UserDetails, Serializable {
/** The id. */
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
Integer id;
/** The username. */
String username;
/** The firstname. */
String firstname;
/** The lastname. */
String lastname;
/** The password. */
String password;
/** The verified email. */
@Column(name = "verified_email")
boolean verifiedEmail;
/** The expired. */
boolean expired;
/** The locked. */
boolean locked;
/** The role. */
@JoinColumn(name = "role_id")
UserRole role;
/** The temp. */
@Transient
private String temp;
/**
* Creates the.
*
* @return the user
*/
public static User create() {
return new User();
}
/**
* Username.
*
* @param username the username
* @return the user
*/
public User username(String username) {
setUsername(username);
return this;
}
/**
* Firstname.
*
* @param firstname the firstname
* @return the user
*/
public User firstname(String firstname) {
setFirstname(firstname);
return this;
}
/**
* Lastname.
*
* @param lastname the lastname
* @return the user
*/
public User lastname(String lastname) {
setLastname(lastname);
return this;
}
/**
* Gets the authorities.
*
* @return the authorities
*/
@Override
public Collection extends GrantedAuthority> getAuthorities() {
if(getRole()==null) {
return null;
}
Vector roles = new Vector<>();
roles.add(getRole());
return roles;
}
/**
* Gets the username.
*
* @return the username
*/
@Override
public String getUsername() {
return username;
}
/**
* Checks if is account non expired.
*
* @return true, if is account non expired
*/
@Override
public boolean isAccountNonExpired() {
return !expired;
}
/**
* Checks if is account non locked.
*
* @return true, if is account non locked
*/
@Override
public boolean isAccountNonLocked() {
return !locked;
}
/**
* Checks if is credentials non expired.
*
* @return true, if is credentials non expired
*/
@Override
public boolean isCredentialsNonExpired() {
return true;
}
/**
* Checks if is enabled.
*
* @return true, if is enabled
*/
@Override
public boolean isEnabled() {
return !locked;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy