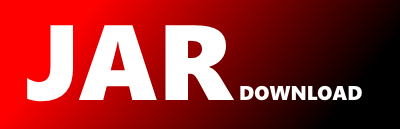
com.jk.webstack.security.controllers.JKAccountWebController Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.webstack.security.controllers;
import com.jk.data.exceptions.JKRecordNotFoundException;
import com.jk.web.faces.controllers.JKWebController;
import com.jk.webstack.services.account.AccountAlreadyExistsException;
import com.jk.webstack.services.account.AccountServices;
import jakarta.enterprise.context.RequestScoped;
import jakarta.inject.Named;
import jakarta.servlet.ServletException;
/**
* The Class MB_Account.
*/
@Named("accountController")
@RequestScoped
public class JKAccountWebController extends JKWebController{
/** The email. */
String email;
/** The first name. */
String firstName;
/** The last name. */
String lastName;
/** The password. */
String password;
/** The created. */
boolean created;
/** The suggest reset. */
boolean suggestReset;
/** The service. */
AccountServices service = new AccountServices();
/**
* Gets the email.
*
* @return the email
*/
public String getEmail() {
return email;
}
/**
* Sets the email.
*
* @param email the new email
*/
public void setEmail(String email) {
this.email = email;
}
/**
* Gets the password.
*
* @return the password
*/
public String getPassword() {
return password;
}
/**
* Sets the password.
*
* @param password the new password
*/
public void setPassword(String password) {
this.password = password;
}
/**
* Gets the first name.
*
* @return the first name
*/
public String getFirstName() {
return firstName;
}
/**
* Sets the first name.
*
* @param firstName the new first name
*/
public void setFirstName(String firstName) {
this.firstName = firstName;
}
/**
* Gets the last name.
*
* @return the last name
*/
public String getLastName() {
return lastName;
}
/**
* Sets the last name.
*
* @param lastName the new last name
*/
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
* Creates the.
*
* @return the string
* @throws ServletException the servlet exception
*/
public String create() throws ServletException {
logAction("Create Account");
try {
service.createAccount(email, firstName, lastName,password);
request().login(email, password);
created = true;
redirect("/");
// getEmailService().addEmailToQueue("[email protected]", "Clowiz New Account", firstName+" "+lastName+","+email, true);
} catch (AccountAlreadyExistsException e) {
suggestReset=true;
error("Account already exists, Please reset password!");
}
// success("Thank you. Please check your Email inbox (including the Spam folder).", false);
return null;
}
/**
* Reset account.
*
* @return the string
*/
public String resetAccount() {
logAction("Reset Account");
try {
service.resetAccount(email);
success("Thank you. Please check your Email inbox (including the spam folder).", false);
request().setAttribute("resetSucc", true);
} catch (JKRecordNotFoundException e) {
error("We cant find this Email in our records.",false);
request().setAttribute("createAccount", true);
}
return null;
}
/**
* Checks if is created.
*
* @return true, if is created
*/
public boolean isCreated() {
return created;
}
/**
* Checks if is suggest reset.
*
* @return true, if is suggest reset
*/
public boolean isSuggestReset() {
return suggestReset;
}
/**
* Sets the suggest reset.
*
* @param suggestReset the new suggest reset
*/
public void setSuggestReset(boolean suggestReset) {
this.suggestReset = suggestReset;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy