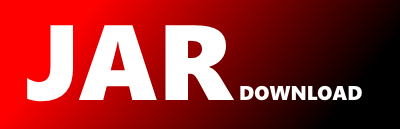
com.jk.webstack.security.controllers.JKSecurityWebController Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.webstack.security.controllers;
/*
* Copyright 2002-2018 Jalal Kiswani.
* E-mail: [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.userdetails.UserDetails;
import com.jk.web.faces.controllers.JKWebController;
import com.jk.webstack.security.User;
import com.jk.webstack.security.UserRole;
import com.jk.webstack.security.services.SecurityService;
import jakarta.enterprise.context.RequestScoped;
import jakarta.inject.Named;
/**
* The Class MB_Login.
*/
@Named("securityController")
@RequestScoped
public class JKSecurityWebController extends JKWebController {
/** The user. */
private User user;
/** The current password. */
String currentPassword;
/** The new password. */
String newPassword;
/**
* Gets the user name.
*
* @return the user name
*/
public String getMessage() {
Object attribute = session().getAttribute("SPRING_SECURITY_LAST_EXCEPTION");
if (attribute == null) {
return null;
}
// session().removeAttribute("SPRING_SECURITY_LAST_EXCEPTION");
if (attribute.toString().contains("BadCredentialsException")) {
return "Invalid username or password";
}
return attribute.toString();
}
/**
* Return account object for current logged in user.
*
* @return the user
*/
public User getUser() {
if (isUserLoggedIn()) {
if (this.user == null) {
this.user = (User) getService().loadUserByUsername(getUserName());
}
return user;
}
return null;
}
/**
* Gets the user name.
*
* @return the user name
*/
public String getUserName() {
Object principal = SecurityContextHolder.getContext().getAuthentication().getPrincipal();
if (principal instanceof UserDetails) {
String username = ((UserDetails) principal).getUsername();
return username;
}
return null;
}
/**
* Checks if is user logged in.
*
* @return true, if is user logged in
*/
public boolean isUserLoggedIn() {
return getUserName()!=null;
}
/**
* Gets the service.
*
* @return the service
*/
protected SecurityService getService() {
return new SecurityService();
}
/**
* Gets the current password.
*
* @return the current password
*/
public String getCurrentPassword() {
return currentPassword;
}
/**
* Sets the current password.
*
* @param currentPassword the new current password
*/
public void setCurrentPassword(String currentPassword) {
this.currentPassword = currentPassword;
}
/**
* Gets the new password.
*
* @return the new password
*/
public String getNewPassword() {
return newPassword;
}
/**
* Sets the new password.
*
* @param newPassword the new new password
*/
public void setNewPassword(String newPassword) {
this.newPassword = newPassword;
}
/**
* Change password.
*
* @return the string
*/
public String changePassword() {
try {
getService().changePassword(getUserName(), currentPassword, newPassword);
success("Password changed successfully");
return null;
} catch (AssertionError e) {
error("Unable to change password, please check your current password");
return null;
}
}
/**
* Checks if is admin.
*
* @return true, if is admin
*/
public boolean isAdmin() {
UserRole role = getRole();
if (role != null) {
return role.getName().equals(UserRole.ADMIN);
}
return false;
}
/**
* Gets the role.
*
* @return the role
*/
private UserRole getRole() {
User user = getUser();
if (user != null) {
return user.getRole();
}
return null;
}
/**
*
* @param role
* @return
*/
public boolean isAuthorized(String role) {
if(getRole()==null) {
return true;
}
if(role==null) {
return false;
}
return getRole().getName().equals(role);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy