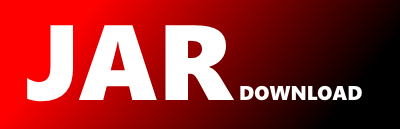
com.jk.webstack.services.account.Account Maven / Gradle / Ivy
/*
* Copyright 2002-2022 Dr. Jalal Kiswani.
* Email: [email protected]
* Check out https://smart-api.com for more details
*
* All the opensource projects of Dr. Jalal Kiswani are free for personal and academic use only,
* for commercial usage and support, please contact the author.
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.webstack.services.account;
import java.io.Serializable;
import java.util.Collection;
import java.util.Date;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.userdetails.UserDetails;
import com.jk.core.jpa.BaseEntity;
import com.jk.core.util.JKDateTimeUtil;
import jakarta.persistence.Column;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import jakarta.persistence.PrePersist;
import jakarta.persistence.PreUpdate;
import jakarta.persistence.Table;
import jakarta.persistence.Transient;
import lombok.Data;
// TODO: Auto-generated Javadoc
/**
* The Class Account.
*/
@Entity
@Table(name = "app_accounts")
/**
* Instantiates a new account.
*/
/**
* Instantiates a new account.
*/
/**
* Instantiates a new account.
*/
/**
* Instantiates a new account.
*/
/**
* Instantiates a new account.
*/
/**
* Instantiates a new account.
*/
/**
* Instantiates a new account.
*/
@Data
public class Account extends BaseEntity implements UserDetails, Serializable {
/** The Constant TOKEN_TIME_OUT. */
private static final int TOKEN_TIME_OUT = 30;
/** The id. */
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
Integer id;
/** The email. */
String email;
/** The firstname. */
String firstname;
/** The lastname. */
String lastname;
/** The password. */
String password;
/** The verified email. */
@Column(name = "verified_email")
boolean verifiedEmail;
/** The expired. */
boolean expired;
/** The locked. */
boolean locked;
/** The one time token. */
@Column(name = "token")
String oneTimeToken;
/** The one token time. */
@Column(name = "token_time")
Date oneTokenTime;
/** The temp. */
@Transient
private String temp;
/**
* Creates the.
*
* @return the account
*/
public static Account create() {
return new Account();
}
/**
* Email.
*
* @param email the email
* @return the account
*/
public Account email(String email) {
setEmail(email);
return this;
}
/**
* Firstname.
*
* @param firstname the firstname
* @return the account
*/
public Account firstname(String firstname) {
setFirstname(firstname);
return this;
}
/**
* Lastname.
*
* @param lastname the lastname
* @return the account
*/
public Account lastname(String lastname) {
setLastname(lastname);
return this;
}
/**
* Gets the authorities.
*
* @return the authorities
*/
@Override
public Collection extends GrantedAuthority> getAuthorities() {
return null;
}
/**
* Gets the username.
*
* @return the username
*/
@Override
public String getUsername() {
return getEmail();
}
/**
* Checks if is account non expired.
*
* @return true, if is account non expired
*/
@Override
public boolean isAccountNonExpired() {
return !expired;
}
/**
* Checks if is account non locked.
*
* @return true, if is account non locked
*/
@Override
public boolean isAccountNonLocked() {
return !locked;
}
/**
* Checks if is credentials non expired.
*
* @return true, if is credentials non expired
*/
@Override
public boolean isCredentialsNonExpired() {
return true;
}
/**
* Checks if is enabled.
*
* @return true, if is enabled
*/
@Override
public boolean isEnabled() {
return !locked;
}
/**
* Checks if is token expired.
*
* @return true, if is token expired
*/
public boolean isTokenExpired() {
Date time = getOneTokenTime();
if (time == null) {
return true;
}
if (JKDateTimeUtil.getMinutesDifference(new Date(), time) > TOKEN_TIME_OUT) {
return false;
}
return false;
}
/**
* Pre persist.
*/
@PrePersist
@Override
public void prePersist() {
super.prePersist();
}
/**
* Pre update.
*/
@Override
@PreUpdate
public void preUpdate() {
super.preUpdate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy