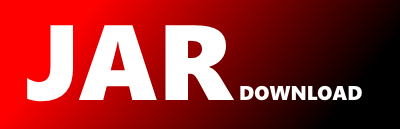
com.jk.util.JK Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jk-util Show documentation
Show all versions of jk-util Show documentation
This is utility classes used by my other projects.
The newest version!
/*
* Copyright 2002-2016 Jalal Kiswani.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.util;
import java.io.InputStream;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.concurrent.ThreadLocalRandom;
import com.jk.exceptions.handler.JKExceptionUtil;
import com.jk.locale.JKLocale;
/**
* The Class JK.
*
* @author Jalal Kiswani
*/
public class JK {
/**
* Debug.
*/
public static void debug() {
if (JKObjectUtil.isClassAvilableInClassPath("org.slf4j.impl.SimpleLogger")) {
System.setProperty("org.slf4j.simpleLogger.defaultLogLevel", "trace");
} else {
JK.error(
"org.slf4j.impl.SimpleLogger is not available in class path, consider adding slf4j-simple dependency to be able to debug.");
}
}
public static void error(Object message) {
System.err.println(message);
}
/** The Constant NEW_LINE. */
public static final String NEW_LINE = System.getProperty("line.separator");
/** The Constant FIELD_SEPARATOR. */
public static final String FIELD_SEPARATOR = ";";
/** The Constant CSV_SEPARATOR. */
public static final String CSV_SEPARATOR = ",";
/** The default locale. */
public static JKLocale DEFAULT_LOCALE = new JKLocale(Locale.ENGLISH);
/**
* Prints the.
*
* @param params the params
*/
public static void print(Object... params) {
String fullText = buildToString(params);
System.out.println(fullText);
}
/**
* Builds the to string.
*
* @param params the params
* @return the string
*/
public static String buildToString(Object... params) {
StringBuffer finalMessage = new StringBuffer();
int i = 0;
for (Object object : params) {
if (i++ > 1) {
finalMessage.append(FIELD_SEPARATOR);
}
if (object instanceof List>) {
finalMessage.append(JKCollectionUtil.toString((List>) object));
} else {
finalMessage.append(JKObjectUtil.toString(object, true));
}
}
String fullText = finalMessage.toString();
return fullText;
}
/**
* Concat.
*
* @param params the params
* @return the string
*/
public static String concat(Object... params) {
StringBuffer finalMessage = new StringBuffer();
for (Object object : params) {
finalMessage.append(JKObjectUtil.toString(object, true));
}
return finalMessage.toString();
}
/**
* Handle.
*
* @param t the t
*/
public static void handle(Throwable t) {
JKExceptionUtil.handle(t);
}
/**
* Line.
*/
public static void line() {
System.out.println("-------------------------------------------------");
}
/**
* Prints the block.
*
* @param params the params
*/
public static void printBlock(Object... params) {
line();
print(params);
line();
}
/**
* Throww.
*
* @param t the t
*/
public static void throww(Throwable t) {
JKExceptionUtil.handle(t);
}
/**
* To map.
*
* @param keys the keys
* @param values the values
* @return the map
*/
public static Map toMap(Object[] keys, Object[] values) {
Map map = new HashMap<>();
int i = 0;
for (Object key : keys) {
map.put(key, values[i++]);
}
return map;
}
/**
* To map.
*
* @param list the list
* @return the map
*/
public static Map toMap(Object... list) {
if (list.length % 2 != 0) {
throw new IllegalArgumentException("List size should be even");
}
Map map = new LinkedHashMap();
for (int i = 0; i < list.length; i += 2) {
map.put(list[i], list[i + 1]);
}
return map;
}
/**
* The main method.
*
* @param args the arguments
*/
public static void main(String[] args) {
System.out.println(toMap("key1", "value1", "key2", "value2"));
}
/**
* Random number.
*
* @return the int
*/
public static int randomNumber() {
return randomNumber(0, Integer.MAX_VALUE);
}
/**
* Random number.
*
* @param min the min
* @param max the max
* @return the int
*/
public static int randomNumber(int min, int max) {
return ThreadLocalRandom.current().nextInt(min, max);
}
/**
* Gets the input stream.
*
* @param fileName the file name
* @return the input stream
*/
public static InputStream getInputStream(String fileName) {
return JKIOUtil.getInputStream(fileName);
}
/**
* Validate null.
*
* @param name the name
* @param object the object
*/
public static void validateNull(String name, Object object) {
if (object == null) {
throw new IllegalStateException(name.concat(" cannot be null"));
}
}
/**
* New instance.
*
* @param the generic type
* @param className the class name
* @return the t
*/
public static T newInstance(String className) {
return JKObjectUtil.newInstance(className);
}
/**
* Sets the default locale.
*
* @param locale the new default locale
*/
public static void setDefaultLocale(JKLocale locale) {
DEFAULT_LOCALE = locale;
}
/**
* Restart.
*/
public static void restart() {
implementMe();
}
public static void exception(String message) {
throw new RuntimeException(message);
}
public static void implementMe() {
throw new IllegalStateException("This method is not implemented yet!");
}
public static void notNull(Object object) {
if (object == null) {
throw new IllegalStateException("This object cannot be null");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy