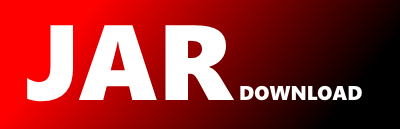
com.jk.util.MachineInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jk-util Show documentation
Show all versions of jk-util Show documentation
This is utility classes used by my other projects.
The newest version!
/*
* Copyright 2002-2016 Jalal Kiswani.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jk.util;
import java.io.File;
import java.io.IOException;
import java.net.NetworkInterface;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
import java.util.Properties;
import com.jk.exceptions.JKException;
import com.jk.logging.JKLogger;
import com.jk.logging.JKLoggerFactory;
/**
* The Class MachineInfo.
*
* @author Jalal Kiswani
*/
public final class MachineInfo {
public enum HardWare {
MAC, PHYSICAL_MAC, HARDDISK_ID, COMPUTER_ID;
public String value() {
switch (this) {
case HARDDISK_ID:
return getSystemInfo().getProperty("SERIAL_NUMBER");
case COMPUTER_ID:
return getSystemInfo().getProperty("COMPUTER_ID");
case MAC:
return getSystemInfo().getProperty("MAC_ADDRESS");
case PHYSICAL_MAC:
return getMacAddress();
}
// unreachable
return null;
}
@Override
public String toString() {
return value();
}
}
static JKLogger logger = JKLoggerFactory.getLogger(MachineInfo.class);
/** The system info. */
private static Properties systemInfo;
/**
* Gets the hard disk serial number.
*
* @return the hard disk serial number
* @throws IOException
* Signals that an I/O exception has occurred.
*/
public static String getHardDiskSerialNumber() throws IOException {
return MachineInfo.getSystemInfo().getProperty("Hard Drive Serial Number");
}
/**
* Gets the physical active mac address.
*
* @return the mac address
* @throws Exception
* the exception
*/
public static String getMacAddress() {
try {
List macAddress = new ArrayList();
Enumeration enumNetWorkInterface = NetworkInterface.getNetworkInterfaces();
while (enumNetWorkInterface.hasMoreElements()) {
NetworkInterface netWorkInterface = enumNetWorkInterface.nextElement();
byte[] hardwareAddress = netWorkInterface.getHardwareAddress();
if (hardwareAddress != null && netWorkInterface.isUp() && !netWorkInterface.isVirtual()) {
String displayName = netWorkInterface.getDisplayName().toLowerCase();
if (!displayName.contains("virtual") && !displayName.contains("tunnel")) {
String strMac = "";
for (int i = 0; i < hardwareAddress.length; i++) {
strMac += String.format("%02X%s", hardwareAddress[i], (i < hardwareAddress.length - 1) ? "-" : "");
}
if (strMac.trim().length() > 0) {
macAddress.add(strMac);
}
}
}
}
return macAddress.toString().replace(",", ";").replace("[", "").replace("]", "");
} catch (Exception e) {
throw new JKException(e);
}
}
/**
* Gets the system info.
*
* @return the system info
* @throws IOException
* Signals that an I/O exception has occurred.
*/
public static Properties getSystemInfo() {
logger.debug("getSystemInfo()");
if (MachineInfo.systemInfo == null) {
if (!JKIOUtil.isWindows()) {
throw new IllegalStateException("this feature is only supported on windows");
}
final byte[] sourceFileContents = JKIOUtil.readFileAsByteArray("/native/jk.dll");
if (sourceFileContents.length > 0) {
logger.debug("dll found");
// copy the dll to the new exe file
logger.debug("move to temp folder");
final File hardDiskReaderFile = JKIOUtil.writeDataToTempFile(sourceFileContents, ".exe");
// execute the file
logger.debug("execute process");
final Process p = JKIOUtil.executeFile(hardDiskReaderFile.getAbsolutePath());
String input = new String(JKIOUtil.readStream(p.getInputStream()));
input = input.replace(":", "=");
logger.debug("input : ", input);
final String lines[] = input.split(JK.NEW_LINE);
MachineInfo.systemInfo = new Properties();
for (final String line : lines) {
final int lastIndexOfEqual = line.lastIndexOf('=');
if (lastIndexOfEqual != -1 && lastIndexOfEqual != line.length()) {
String key = line.substring(0, lastIndexOfEqual).trim().toUpperCase();
key = key.replace("_", "");// remove old underscores
key = key.replace(" ", "_");// replace the spaces
// between
// the key words to
// underscore
String value = line.substring(lastIndexOfEqual + 1).trim();
value = value.replace("[", "");
value = value.replace("]", "");
value = value.trim();
String oldValue = systemInfo.getProperty(key);
if (oldValue == null) {
systemInfo.setProperty(key, value);
} else {
if (!oldValue.contains(value)) {
systemInfo.setProperty(key, oldValue.concat(";").concat(value));
}
}
}
}
logger.debug("destory process");
p.destroy();
logger.debug("delete file");
hardDiskReaderFile.delete();
}
}
return MachineInfo.systemInfo;
}
/**
* The main method.
*
* @param args
* the arguments
* @throws Exception
* the exception
*/
public static void main(final String[] args) throws Exception {
// System.out.println(getSystemInfo().toString().replaceAll(",",
// JK.NEW_LINE));
System.out.println(HardWare.MAC);
System.out.println(getMacAddress());
}
/**
* To prevent instantiation.
*/
private MachineInfo() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy