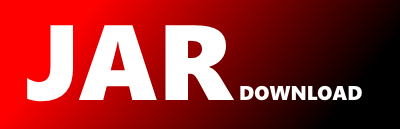
com.jameskleeh.excel.Formula.groovy Maven / Gradle / Ivy
package com.jameskleeh.excel
import groovy.transform.CompileStatic
import org.apache.poi.ss.util.CellReference
import org.apache.poi.xssf.usermodel.XSSFCell
/**
* A class to get references to cells for use in formulas
*
* @author James Kleeh
* @since 1.0.0
*/
@CompileStatic
class Formula {
private final XSSFCell cell
private final Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy