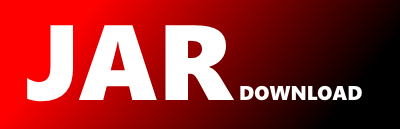
com.jameskleeh.excel.Sheet.groovy Maven / Gradle / Ivy
package com.jameskleeh.excel
import groovy.transform.CompileStatic
import org.apache.poi.xssf.usermodel.XSSFRow
import org.apache.poi.xssf.usermodel.XSSFSheet
import org.apache.poi.xssf.usermodel.XSSFWorkbook
/**
* A class used to create a sheet in an excel document
*/
@CompileStatic
class Sheet {
private final XSSFSheet sheet
private final XSSFWorkbook workbook
private int rowIdx
private Map defaultOptions
private Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy