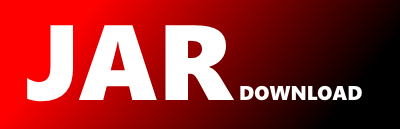
com.jamierf.dbtable.core.DbTable Maven / Gradle / Ivy
package com.jamierf.dbtable.core;
import com.google.common.base.Preconditions;
import com.google.common.collect.Table;
import com.jamierf.dbtable.core.mapper.result.field.ByteArrayFieldMapperFactory;
import com.jamierf.dbtable.core.mapper.result.field.FieldMapper;
import com.jamierf.dbtable.core.mapper.result.map.MapEntryMapper;
import com.jamierf.dbtable.core.mapper.result.table.TableCellMapper;
import com.jamierf.dbtable.core.mapper.result.table.TableCellMapperFactory;
import com.jamierf.dbtable.core.mapper.selection.FieldSelectionMapFactory;
import com.jamierf.dbtable.core.mapper.selection.SelectionMap;
import com.jamierf.dbtable.core.mapper.selection.TableCellSelectionMapFactory;
import org.skife.jdbi.v2.Handle;
import org.skife.jdbi.v2.PreparedBatch;
import org.skife.jdbi.v2.StatementContext;
import org.skife.jdbi.v2.util.ByteArrayMapper;
import org.skife.jdbi.v2.util.IntegerMapper;
import javax.annotation.Nullable;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
public class DbTable implements Table {
private static final TableCellMapperFactory TABLE_CELL_MAPPER_FACTORY = new TableCellMapperFactory<>(
new ByteArrayFieldMapperFactory(), new ByteArrayFieldMapperFactory(), new ByteArrayFieldMapperFactory()
);
private final String tableName;
private final Handle handle;
private final TableCellMapper tableCellMapper;
public DbTable(String tableName, Handle handle) {
this.tableName = Preconditions.checkNotNull(tableName);
this.handle = Preconditions.checkNotNull(handle);
tableCellMapper = TABLE_CELL_MAPPER_FACTORY.build("row_field", "column_field", "value_field");
createTableIfRequired();
}
private void createTableIfRequired() {
handle.execute(String.format("CREATE TABLE IF NOT EXISTS %s (row_field VARBINARY NOT NULL, column_field VARBINARY NOT NULL, value_field BLOB NOT NULL, PRIMARY KEY (row_field, column_field))", tableName));
}
@Override
public boolean contains(Object row, Object column) {
return handle.createQuery(String.format("SELECT 1 FROM %s WHERE row_field = :row_field AND column_field = :column_field", tableName))
.bind("row_field", row)
.bind("column_field", column)
.map(IntegerMapper.FIRST)
.first() != null;
}
@Override
public boolean containsRow(Object row) {
return handle.createQuery(String.format("SELECT 1 FROM %s WHERE row_field = :row_field", tableName))
.bind("row_field", row)
.map(IntegerMapper.FIRST)
.first() != null;
}
@Override
public boolean containsColumn(Object column) {
return handle.createQuery(String.format("SELECT 1 FROM %s WHERE column_field = :column_field", tableName))
.bind("column_field", column)
.map(IntegerMapper.FIRST)
.first() != null;
}
@Override
public boolean containsValue(Object value) {
return handle.createQuery(String.format("SELECT 1 FROM %s WHERE value_field = :value_field", tableName))
.bind("value_field", value)
.map(IntegerMapper.FIRST)
.first() != null;
}
@Override
public byte[] get(Object row, Object column) {
return handle.createQuery(String.format("SELECT value_field FROM %s WHERE row_field = :row_field AND column_field = :column_field", tableName))
.bind("row_field", row)
.bind("column_field", column)
.map(ByteArrayMapper.FIRST)
.first();
}
@Override
public boolean isEmpty() {
return handle.createQuery(String.format("SELECT 1 FROM %s", tableName))
.map(IntegerMapper.FIRST)
.first() == null;
}
@Override
public int size() {
return handle.createQuery(String.format("SELECT COUNT(value_field) FROM %s", tableName))
.map(IntegerMapper.FIRST)
.first();
}
@Override
public void clear() {
handle.execute(String.format("DELETE FROM %s", tableName));
}
@Override
public byte[] put(@Nullable byte[] row, @Nullable byte[] column, @Nullable byte[] value) {
final byte[] result = get(row, column);
handle.insert(String.format("REPLACE INTO %s VALUES (:row_field, :column_field, :value_field)", tableName), row, column, value);
return result;
}
@Override
public void putAll(@Nullable Table extends byte[], ? extends byte[], ? extends byte[]> table) {
final PreparedBatch batch = handle.prepareBatch(String.format("REPLACE INTO %s VALUES (:row_field, :column_field, :value_field)", tableName));
for (Table.Cell extends byte[], ? extends byte[], ? extends byte[]> cell : table.cellSet()) {
batch.add()
.bind("row_field", cell.getRowKey())
.bind("column_field", cell.getColumnKey())
.bind("value_field", cell.getValue());
}
batch.execute();
}
@Override
public byte[] remove(Object row, Object column) {
final byte[] result = get(row, column);
handle.execute(String.format("DELETE FROM %s WHERE row_field = :row_field AND column_field = :column_field", tableName), row, column);
return result;
}
@Override
@SuppressWarnings("unchecked")
public Map row(@Nullable byte[] row) {
final MapEntryMapper mapper = TABLE_CELL_MAPPER_FACTORY.getRowMapMapperFactory().build("column_field", "value_field");
return new DbMap(tableName, handle, SelectionMap.of("row_field", row), new FieldSelectionMapFactory("column_field"), mapper);
}
@Override
@SuppressWarnings("unchecked")
public Map column(@Nullable byte[] column) {
final MapEntryMapper mapper = TABLE_CELL_MAPPER_FACTORY.getColumnMapMapperFactory().build("row_field", "value_field");
return new DbMap<>(tableName, handle, SelectionMap.of("column_field", column), new FieldSelectionMapFactory("row_field"), mapper);
}
@Override
@SuppressWarnings("unchecked")
public Set> cellSet() {
return new DbSet<>(tableName, handle, SelectionMap.NONE, new TableCellSelectionMapFactory(
"row_field", "column_field", "value_field"), tableCellMapper);
}
@Override
@SuppressWarnings("unchecked")
public Set rowKeySet() {
final FieldMapper mapper = tableCellMapper.getRowMapper();
return new DbSet<>(tableName, handle, SelectionMap.NONE, new FieldSelectionMapFactory("row_field"), mapper);
}
@Override
@SuppressWarnings("unchecked")
public Set columnKeySet() {
final FieldMapper mapper = tableCellMapper.getColumnMapper();
return new DbSet<>(tableName, handle, SelectionMap.NONE, new FieldSelectionMapFactory("column_field"), mapper);
}
@Override
@SuppressWarnings("unchecked")
public Collection values() {
final FieldMapper mapper = tableCellMapper.getValueMapper();
return new DbCollection<>(tableName, handle, SelectionMap.NONE, new FieldSelectionMapFactory("value_field"), mapper);
}
@Override
@SuppressWarnings("unchecked")
public Map> rowMap() {
final MapEntryMapper> mapper = new MapEntryMapper<>(tableCellMapper.getRowMapper(), new FieldMapper |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy